In the world of distributed systems and microservices, observability has become crucial for maintaining and troubleshooting complex applications. OpenTelemetry stands at the forefront of this observability revolution, providing a standardized way to collect and transmit telemetry data. But when it comes to implementing OpenTelemetry, you face a critical decision: should you use gRPC or HTTP for your tracing data transmission? This choice can significantly impact your system's performance, efficiency, and overall observability strategy.
What is OpenTelemetry and Why is Protocol Choice Important?
OpenTelemetry is an open-source framework for observability, which means it helps track and understand the behaviour of complex systems. Designed to work across various platforms, OpenTelemetry collects telemetry data like traces, metrics, and logs, giving developers insights into their applications' performance. This is especially valuable for troubleshooting and monitoring systems made up of many small, interconnected services—often called "microservices." By using OpenTelemetry, teams can take a vendor-neutral approach to observing their applications, which means they are not locked into any one tool or platform.
Choosing the right protocol to transmit this telemetry data is essential because it impacts four key areas:
- Performance: The speed and efficiency with which telemetry data moves between services can affect how responsive the system is. Faster transmission helps ensure accurate, real-time monitoring.
- Scalability: As systems grow and more telemetry data is generated, the protocol needs to handle this increase without slowing down or causing bottlenecks.
- Compatibility: The protocol should work well with your existing infrastructure and any monitoring tools you use, making it easier to integrate OpenTelemetry into your setup.
- Resource Usage: Different protocols use system resources like CPU, memory, and bandwidth differently. Optimizing this helps keep resource consumption low and predictable.
In OpenTelemetry, the two main protocols are gRPC and HTTP. gRPC is faster and more efficient, especially for high-volume data transfers, while HTTP is simpler and more compatible with a broader range of systems. Choosing between them is a critical decision that shapes how effective your observability setup will be.
Understanding gRPC in OpenTelemetry
Understanding gRPC in OpenTelemetry
gRPC, which stands for gRPC Remote Procedure Call, is a high-performance communication protocol developed by Google, designed to make data transfer fast and efficient. In OpenTelemetry, gRPC is often used for sending large amounts of telemetry data, such as traces and metrics, because of its speed and low-latency capabilities. A key part of gRPC’s design is its use of Protocol Buffers, a language-neutral, binary serialization format that makes data compact and quick to transmit. This helps OpenTelemetry systems efficiently manage data flow between various microservices and components.
Key Features of gRPC:
- Bi-directional Streaming: Allows data to be sent and received continuously in real-time.
- Flow Control and Multiplexing: gRPC can handle multiple requests over a single connection, thanks to HTTP/2, which reduces the load of managing separate connections.
- Binary Protocol: Using Protocol Buffers, gRPC ensures data is serialized efficiently, resulting in faster transmission and reduced bandwidth usage.
- Language Agnostic: Supports many languages, making it versatile for diverse software ecosystems.
Benefits of gRPC for OpenTelemetry:
- High Performance: Ideal for applications that need quick, high-throughput data transfer.
- Efficient Serialization: Protocol Buffers offer a compact data format, which is lighter than text-based formats like JSON.
- Strong Typing: Helps developers detect issues early, reducing potential errors in data transmission.
- Cross-Language Compatibility: Developers can use gRPC across different programming languages without integration issues.
Potential Drawbacks of gRPC:
- Setup Complexity: Compared to HTTP, gRPC may require more configuration and infrastructure.
- Firewall Compatibility: Some firewalls struggle with HTTP/2, which gRPC depends on, potentially requiring additional adjustments.
- Browser Limitations: gRPC lacks native support in web browsers, making it less flexible for direct browser-based applications.
In OpenTelemetry, gRPC shines in high-performance systems, especially those needing low-latency communication, efficient data handling, and robust support for complex data structures.
Technical Deep Dive: gRPC in Action with OpenTelemetry
gRPC is a high-performance RPC framework that utilizes Protocol Buffers for efficient data serialization, making it an ideal choice for the high-throughput requirements of OpenTelemetry. This section explores how gRPC is implemented in OpenTelemetry for trace data transmission, leveraging the advantages of HTTP/2 as its underlying transport protocol.
Defining Trace Services with Protocol Buffers
Protocol Buffers offer a highly efficient method of serializing structured data. Here is an example of how a service might be defined using Protocol Buffers for the purpose of sending trace data in an OpenTelemetry context:
syntax = "proto3";
package opentelemetry.proto.collector.trace.v1;
service TraceService {
rpc Export(ExportTraceServiceRequest) returns (ExportTraceServiceResponse) {}
}
message ExportTraceServiceRequest {
repeated ResourceSpans resource_spans = 1;
}
message ExportTraceServiceResponse {
// Response details might include status and error messages
}
This definition sets the foundation for robust data communication between clients and servers, specifying the data structure for trace information.
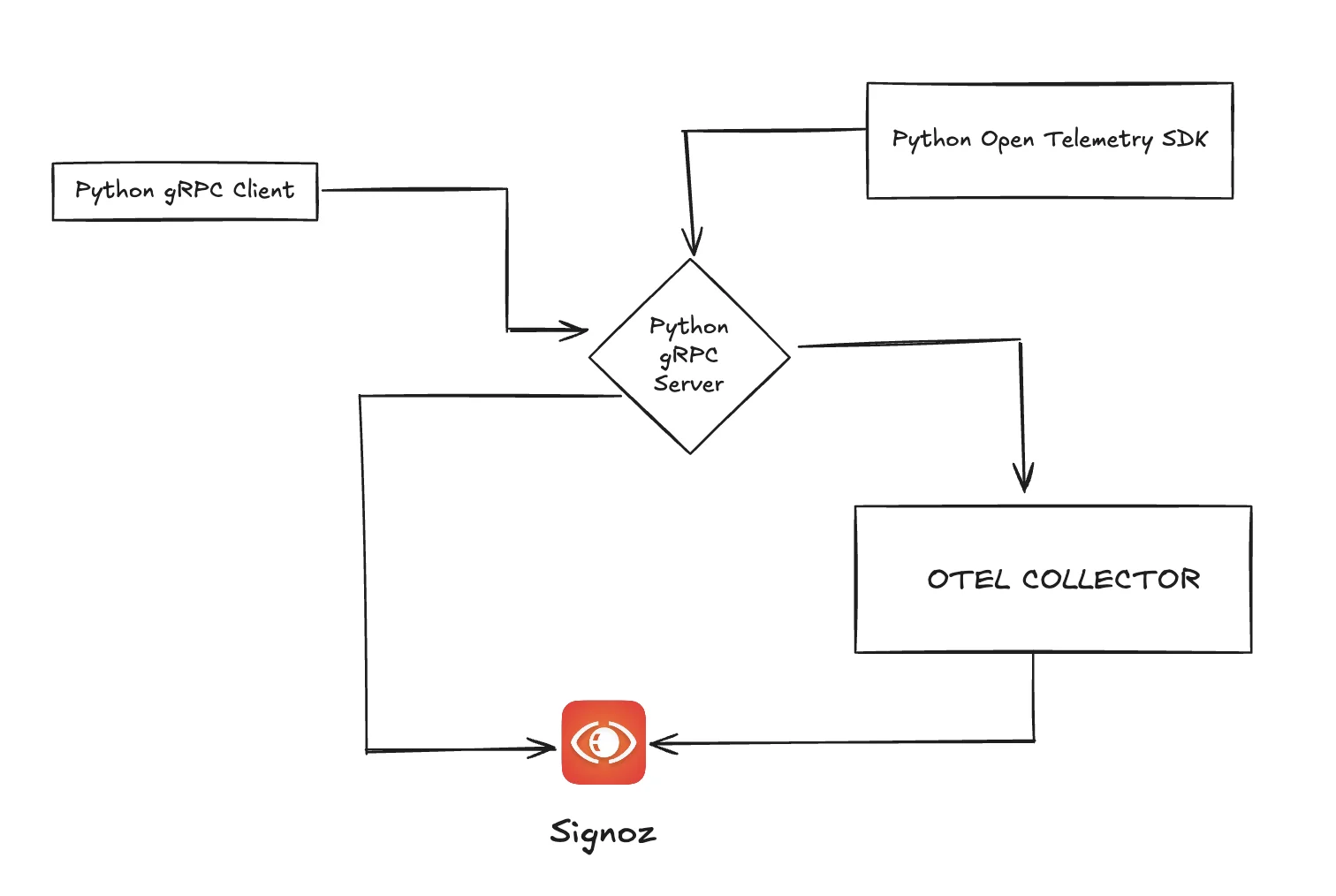
Advantages of Using HTTP/2 in gRPC
gRPC's use of HTTP/2 introduces several significant enhancements that boost its efficiency and performance:
- Header Compression: HTTP/2 compresses headers, reducing overhead and improving latency.
- Multiplexing: This feature allows multiple requests to be sent over a single TCP connection without waiting for responses to the previous ones, effectively reducing the resource costs and improving the throughput.
- Server Push: This capability enables servers to proactively send resources to a client, a feature that can optimize interactions that require multiple round-trips.
Implementing gRPC in Python for OpenTelemetry
Setting up a gRPC client in Python for exporting traces can be demonstrated with the following code snippet, showcasing the practical implementation of the gRPC service defined earlier:
import grpc
from opentelemetry.proto.collector.trace.v1 import trace_service_pb2_grpc
from opentelemetry.proto.collector.trace.v1 import trace_service_pb2
def create_grpc_channel():
return grpc.insecure_channel('localhost:4317')
def export_traces(channel, traces):
stub = trace_service_pb2_grpc.TraceServiceStub(channel)
request = trace_service_pb2.ExportTraceServiceRequest(resource_spans=traces)
response = stub.Export(request)
return response
# Usage
channel = create_grpc_channel()
export_traces(channel, traces_data)
This setup involves creating a gRPC channel, defining a stub for the trace service, and sending trace data through the Export
method, which communicates with the OpenTelemetry collector.
Performance Implications
In high-throughput environments, gRPC stands out due to its low latency and minimal CPU usage compared to traditional REST APIs. It can handle thousands of requests per second, thanks to the efficiencies brought by Protocol Buffers and HTTP/2. This makes gRPC a superior choice for systems where performance, efficiency, and scalability are critical.
In summary, gRPC’s integration with OpenTelemetry using HTTP/2 and Protocol Buffers not only enhances the performance of telemetry data transmission but also ensures greater scalability and efficiency in handling extensive tracing data across distributed systems.
HTTP Protocol in OpenTelemetry: A Practical Alternative
While gRPC is known for its high performance, HTTP remains a staple for telemetry data transmission within OpenTelemetry due to its widespread support and ease of use. Particularly when employed with the OpenTelemetry Protocol (OTLP), HTTP offers a familiar and accessible method for data transmission across various platforms.
Advantages of HTTP in Telemetry Data Transmission
HTTP's primary benefits include:
- Ubiquity: Supported by almost all network infrastructure, making it nearly universally applicable.
- Simplicity: Easier to configure and troubleshoot than more complex protocols like gRPC.
- Firewall Compatibility: Generally allowed by default on most network firewalls.
- Browser Support: Facilitates direct communication from web browsers, essential for client-side telemetry collection.
Despite its advantages, HTTP also has limitations, particularly when compared to gRPC:
- Performance Overhead: Less efficient, especially with large data volumes due to its verbose nature.
- Streaming Capabilities: Lacks native support for bi-directional streaming, though this is partially mitigated by HTTP/2.
- Bandwidth Usage: HTTP headers can significantly increase bandwidth consumption, impacting efficiency for smaller data payloads.
HTTP is often the preferred choice over gRPC when compatibility with legacy systems, simplicity in setup, and direct browser integration are prioritized, especially in environments not optimized for gRPC or where data volumes are lower.
Implementing OTLP/HTTP in OpenTelemetry
OTLP/HTTP, OpenTelemetry's implementation of its protocol over HTTP, enhances flexibility and compatibility. It supports both Protocol Buffers and JSON formats, catering to different needs and preferences in data encoding. This adaptability makes OTLP/HTTP particularly appealing for organizations seeking a straightforward approach to telemetry that integrates seamlessly with existing HTTP-based infrastructures.
Example: Sending Traces with OTLP/HTTP
Here’s how you might send trace data using OTLP/HTTP in Python:
import requests
import json
def send_traces_http(traces):
url = '<http://localhost:4318/v1/traces>'
headers = {'Content-Type': 'application/json'}
data = json.dumps(traces)
response = requests.post(url, headers=headers, data=data)
return response
send_traces_http(traces_data)
While OTLP/HTTP does not always match the performance metrics of gRPC in high-demand scenarios, its ease of use and broad compatibility make it a viable option for many applications, particularly where complex setup and maintenance are a concern. For many use cases, the simplicity and accessibility of HTTP provide a significant advantage, balancing out the performance trade-offs.
Comparative Analysis: gRPC vs. HTTP in OpenTelemetry Tracing
When implementing OpenTelemetry for tracing distributed systems, the choice between gRPC and HTTP protocols can significantly impact the efficiency and functionality of the observability infrastructure. Here's a detailed comparison of gRPC and HTTP based on their essential features, usage in network infrastructure, and implications on codebase and performance.
Key Features Comparison
The table below highlights the primary differences between gRPC and HTTP in various operational aspects relevant to OpenTelemetry:
Feature | gRPC | HTTP |
---|---|---|
Speed | Faster due to HTTP/2 | Slower, depends on version (HTTP/1.1, HTTP/2) |
Efficiency | High, with binary serialization | Lower, especially with textual data |
Ease of use | Complex setup and maintenance | Simpler and more straightforward |
Compatibility | May require specific configurations | Widely supported by existing infrastructures |
Streaming | Supports bi-directional streaming | Primarily unidirectional (HTTP/2 improves this) |
Data format | Primarily binary (Protocol Buffers) | More flexible (supports JSON, Binary) |
Browser support | Limited, not natively supported in browsers | Extensive, direct browser support |
Network Infrastructure Considerations
Firewalls and Security: gRPC, which operates over HTTP/2, might face challenges with some traditional firewalls that do not recognize or properly handle HTTP/2 traffic, potentially blocking gRPC communications. In contrast, HTTP, especially HTTP/1.1, is generally well-accepted by most firewall configurations.
Load Balancing and Proxies: Not all load balancers support gRPC as they need to understand HTTP/2 for effective load distribution. HTTP benefits from broader support with existing network load balancers and proxy servers, which are more accustomed to traditional HTTP traffic.
Impact on Codebase and Dependencies
Implementing gRPC involves integrating specific libraries tailored for gRPC communication, which can add complexity to the project. It requires developers to manage additional dependencies and potentially steepen the learning curve. Conversely, HTTP can typically be implemented using standard libraries present in most programming environments, making it a less burdensome choice for teams seeking simplicity and quick deployments.
Performance Implications
In terms of performance, gRPC generally outperforms HTTP in high-throughput and low-latency requirements. It efficiently handles multiple simultaneous requests with minimal overhead, thanks to HTTP/2's advanced features such as stream multiplexing and header compression. Real-world benchmarks often show gRPC handling significantly more requests per second compared to HTTP, illustrating its capability to support more demanding applications effectively.
Choosing Between gRPC and HTTP for OpenTelemetry: A Guide
Selecting the right protocol for your OpenTelemetry implementation is crucial for optimizing telemetry practices and system performance. Here’s how to determine whether gRPC or HTTP is more suitable for your needs:
Key Factors to Consider
- Scalability Requirements: gRPC is generally superior for handling high volumes of telemetry data efficiently due to its high throughput and low latency characteristics.
- Existing Infrastructure Compatibility: HTTP may be a more suitable choice if your existing system architecture and networking equipment are optimized for HTTP traffic, as it requires less specific configuration compared to gRPC.
- Team Expertise: The familiarity of your team with either protocol can significantly impact the ease of implementation and ongoing maintenance. gRPC might require a steeper learning curve due to its complex setup.
- Performance Needs: For applications where performance, especially low latency, is critical, gRPC offers advantages with its efficient communication model based on HTTP/2.
- Compatibility Requirements: If your application needs to interact seamlessly with a wide range of client environments, including web browsers, HTTP’s universal support makes it a practical choice.
Decision-Making Framework
To make an informed decision, follow these steps:
- Assess Telemetry Volume: Evaluate both current and anticipated data volumes to determine which protocol can handle your scalability needs.
- Evaluate Infrastructure Fit: Check how well your existing infrastructure can support each protocol, considering any necessary changes or updates.
- Analyze Team Skill Set: Reflect on your team’s expertise and the learning curve associated with each protocol.
- Performance vs. Simplicity: Balance the need for high performance with the operational simplicity your team can manage.
- Conduct Protocol Testing: If possible, perform tests with both protocols in your environment to observe and compare their performance and integration capabilities.
Best Practices for Implementation
- Instrumentation: Ensure your application is properly instrumented to collect telemetry data effectively.
- Utilize Official SDKs: Implement using the official OpenTelemetry SDKs and exporters to guarantee compatibility and support.
- Robust Error Handling: Develop a comprehensive error handling and retry strategy to enhance data integrity.
- Continuous Monitoring: Keep track of your telemetry pipeline’s performance to identify and resolve issues promptly.
Considering a Hybrid Approach
In some cases, a hybrid protocol approach may be beneficial. For instance, using gRPC for backend services where data volume and speed are critical, and HTTP for frontend or external services where compatibility and ease of integration are paramount.
This strategic approach allows you to leverage the strengths of both protocols tailored to specific parts of your system, ensuring optimal performance and flexibility.
Implementing Efficient Tracing with SigNoz
SigNoz is an open-source, OpenTelemetry-native Application Performance Monitoring (APM) tool that supports both gRPC and HTTP protocols for data ingestion. This flexibility allows you to choose the best protocol for your needs while benefiting from SigNoz's powerful analysis and visualization capabilities.
Key features of SigNoz:
- Full support for OpenTelemetry data formats
- Real-time monitoring and alerting
- Customizable dashboards
- Distributed tracing visualization
- Exception tracking and error monitoring
To set up SigNoz for efficient tracing:
- Install SigNoz using Docker or Kubernetes.
- Configure your application to send telemetry data to SigNoz.
- Use the SigNoz UI to analyze your traces, metrics, and logs.
SigNoz cloud is the easiest way to run SigNoz. Sign up for a free account and get 30 days of unlimited access to all features.

You can also install and self-host SigNoz yourself since it is open-source. With 20,000+ GitHub stars, open-source SigNoz is loved by developers. Find the instructions to self-host SigNoz.
Future Trends in OpenTelemetry Protocols
The OpenTelemetry ecosystem is continuously advancing, driven by the need to enhance observability in increasingly complex IT environments. Here are key trends that are shaping the future of OpenTelemetry protocols and might influence how organizations choose and implement these technologies:
- OTLP Enhancements: The OpenTelemetry Protocol (OTLP) is seeing continuous improvements aimed at boosting performance and providing greater flexibility. These enhancements ensure that OTLP remains adaptable to evolving data transmission needs, catering to both current and future observability requirements.
- Increased gRPC Adoption: gRPC is gaining broader acceptance across various platforms and infrastructures. Its efficiency in high-throughput scenarios makes it an attractive protocol for modern distributed systems. As more tools and systems integrate gRPC support, its adoption is expected to rise, offering enhanced performance benefits for telemetry data transmission.
- Advancements with HTTP/3: The upcoming HTTP/3 protocol introduces significant performance improvements over its predecessors, including reduced latency and better congestion control. These advancements may diminish the performance disparities between HTTP and gRPC, making HTTP/3 a more competitive choice for telemetry data handling in certain scenarios.
- Edge Computing Protocols: As edge computing grows, there's an increasing need for protocols that can efficiently handle edge-to-cloud communication. Future developments may introduce new or optimized protocols that are better suited for the latency-sensitive and bandwidth-efficient requirements of edge computing environments.
These evolving trends suggest a dynamic future for OpenTelemetry protocols, where ongoing innovations could substantially impact how telemetry data is collected, transmitted, and analyzed. For organizations leveraging OpenTelemetry, staying updated with these developments is crucial. Periodically reassessing protocol choices in light of new technologies and shifting requirements will be essential to maintain effective and efficient observability practices as the landscape evolves.
Key Takeaways
- Both gRPC and HTTP are viable options for OpenTelemetry tracing, each with unique strengths.
- gRPC offers high performance and efficiency, ideal for high-throughput scenarios.
- HTTP provides better compatibility and easier implementation in some environments.
- Your choice should be based on specific project requirements and infrastructure constraints.
- Regular evaluation of protocol performance and evolving needs is crucial for optimal tracing efficiency.
- Tools like SigNoz can help you implement efficient tracing regardless of your chosen protocol.
FAQs
What are the default ports for gRPC and HTTP in OpenTelemetry?
The default port for gRPC in OpenTelemetry is 4317, while for HTTP it's 4318.
Can I switch between gRPC and HTTP protocols without major code changes?
If you're using the official OpenTelemetry SDKs, switching between protocols often requires minimal code changes, mainly in the exporter configuration.
How does the choice between gRPC and HTTP affect data compression in OpenTelemetry?
gRPC uses Protocol Buffers, which provides efficient binary encoding. HTTP can use various compression methods (gzip, deflate) but generally isn't as efficient, especially for small payloads.
Are there any security implications to consider when choosing between gRPC and HTTP for OpenTelemetry?
Both protocols can be secured with TLS. However, HTTP has a more mature ecosystem of security tools and practices, while gRPC's security features are still evolving in some areas.