Nodejs is a popular Javascript runtime environment that executes Javascript code outside of a web browser. Express is the most popular web frameworks that sits on top of Nodejs and adds functionalities like middleware, routing, etc. to Nodejs.

You can monitor your express application using OpenTelemetry and a tracing backend of your choice. OpenTelemetry is the leading open-source standard under the Cloud Native Computing Foundation that aims to standardize the process of instrumentation across multiple languages.
In this article, we will be using SigNoz to store and visualize the telemetry data collected by OpenTelemetry from a sample Expressjs application.
Running an Express application with OpenTelemetry
OpenTelemetry is a set of tools, APIs, and SDKs used to instrument applications to create and manage telemetry data(logs, metrics, and traces).
Install SigNoz
You can get started with SigNoz using just three commands at your terminal.
git clone -b main https://github.com/SigNoz/signoz.git
cd signoz/deploy/
./install.sh
For detailed instructions, you can visit our documentation.
When you are done installing SigNoz, you can access the UI at:ย http://localhost:3301
The application list shown in the dashboard is from a sample app called HOT R.O.D that comes bundled with the SigNoz installation package.
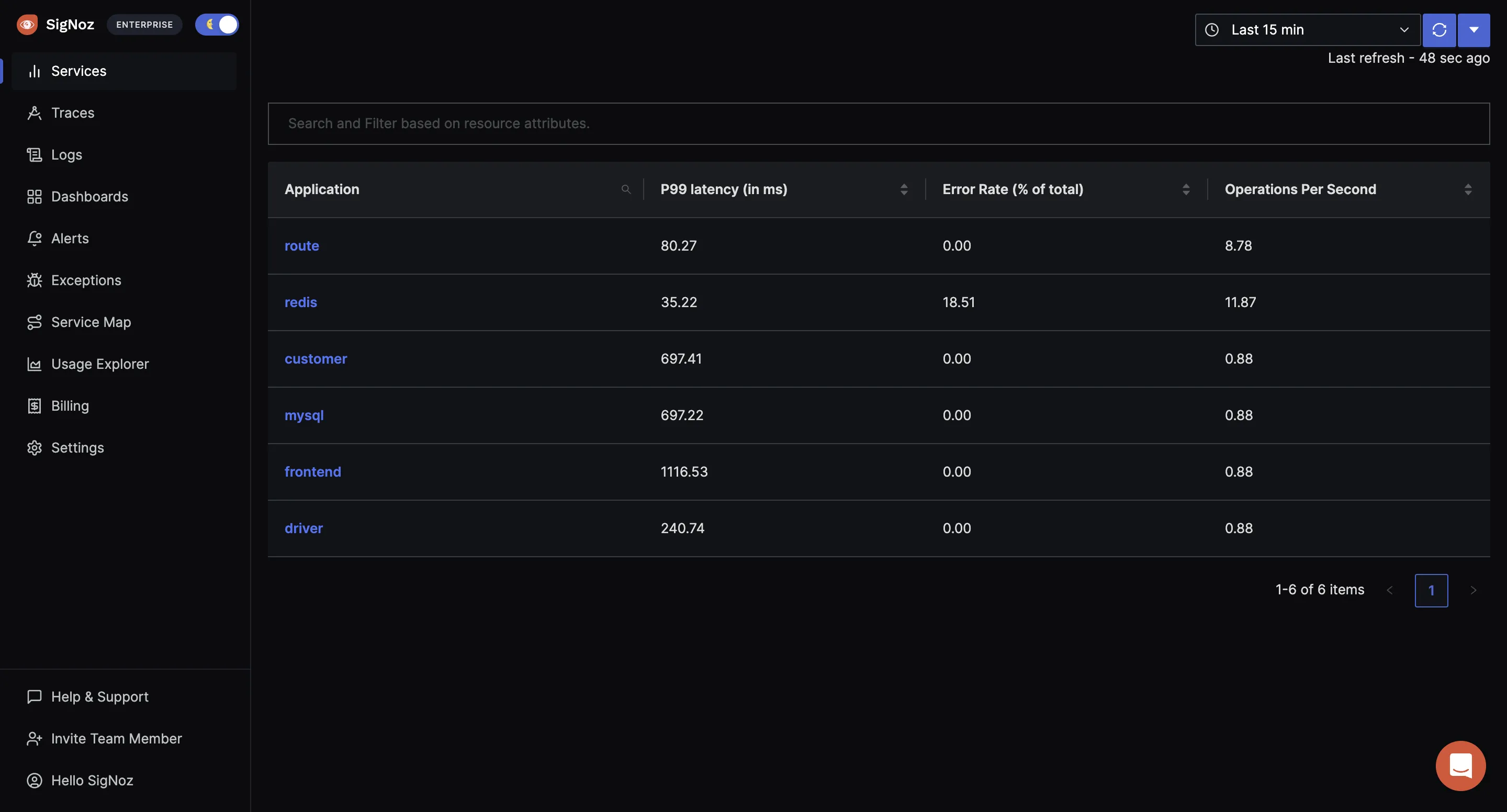
Creating a sample express application
You need to ensure that you have Node.js version 12 or newer. You can download the latest version of Node.js here. For the sample application, let's create a basic 'hello world' express.js application.
If you do not want to follow these steps manually, you can directly check out the GitHub repo of the sample application. You can run the app directly after cloning it and start sending data to SigNoz. The code is already instrumented with OpenTelemetry libraries.
But, it would be better if you follow these steps to understand what's happening.
Check if node is installed on your machine by using the below command:
node - v;
Steps to get the app set up and running:
Make a directory and install express
Make a directory for your sample app on your machine. Then open up the terminal, navigate to the directory path and install express with the following command:npm i express
Create index.js
Create a file calledindex.js
in your directory and with any text editor setup your 'Hello World' file with the code below:const express = require("express"); const cors = require("cors"); const PORT = process.env.PORT || "5555"; const app = express(); app.use(cors()); app.use(express.json()); app.all("/", (req, res) => { res.json({ method: req.method, message: "Hello World", ...req.body }); }); app.get("/404", (req, res) => { res.sendStatus(404); }); app.listen(parseInt(PORT, 10), () => { console.log(`Listening for requests on http://localhost:${PORT}`); });
Check if your application is working
Run your application by using the below command at your terminal.node index.js
You can check if your app is working by visiting: http://localhost:5555/
Once you are finished checking, exit the application by using
Ctrl + C
on your terminal.
Set up OpenTelemetry and send data to SigNoz
Install OpenTelemetry packages
You will need the following OpenTelemetry packages for this sample application.npm install --save @opentelemetry/sdk-node npm install --save @opentelemetry/auto-instrumentations-node npm install --save @opentelemetry/exporter-trace-otlp-http
The dependencies included are briefly explained below:
@opentelemetry/sdk-node
- This package provides the full OpenTelemetry SDK for Node.js including tracing and metrics.@opentelemetry/auto-instrumentations-node
- This module provides a simple way to initialize multiple Node instrumentations.@opentelemetry/exporter-trace-otlp-http
- This module provides the exporter to be used with OTLP (http/json
) compatible receivers.<Admonition"> If you run into any error, you might want to use these pinned versions of OpenTelemetry libraries used in this <a href = "https://github.com/SigNoz/sample-nodejs-app/blob/master/package.json" rel="noopener noreferrer nofollow" target="_blank">GitHub repo</a>. </Admonition>
Create
tracing.js
file
Instantiate tracing by creating atracing.js
file and using the below code.// tracing.js "use strict"; const process = require("process"); const opentelemetry = require("@opentelemetry/sdk-node"); const { getNodeAutoInstrumentations, } = require("@opentelemetry/auto-instrumentations-node"); const { OTLPTraceExporter, } = require("@opentelemetry/exporter-trace-otlp-http"); const { Resource } = require("@opentelemetry/resources"); const { SemanticResourceAttributes, } = require("@opentelemetry/semantic-conventions"); const exporterOptions = { url: "http://localhost:4318/v1/traces", }; const traceExporter = new OTLPTraceExporter(exporterOptions); const sdk = new opentelemetry.NodeSDK({ traceExporter, instrumentations: [getNodeAutoInstrumentations()], resource: new Resource({ [SemanticResourceAttributes.SERVICE_NAME]: "node_app", }), }); // initialize the SDK and register with the OpenTelemetry API // this enables the API to record telemetry sdk.start(); // gracefully shut down the SDK on process exit process.on("SIGTERM", () => { sdk .shutdown() .then(() => console.log("Tracing terminated")) .catch((error) => console.log("Error terminating tracing", error)) .finally(() => process.exit(0)); });
OpenTelemetry Node SDK currently does not detect the
OTEL_RESOURCE_ATTRIBUTES
from.env
files as of today. Thatโs why we need to include the variables in thetracing.js
file itself.About environment variables:
service_name
: name of the service you want to monitorenvironment
: dev, prod, staging, etc.http://localhost:4318/v1/traces
is the default url for sending your tracing data. We are assuming you have installed SigNoz on yourlocalhost
. Based on your environment, you can update it accordingly. It should be in the following format:http://<IP of SigNoz backend>:4318/v1/traces
๐ NoteRemember to allow incoming requests to port 4318 of machine where SigNoz backend is hosted.
Run your application
Now when you run your application, OpenTelemetry captures telemetry data from it and send it to SigNoz.node -r ./tracing.js index.js
You can check your application running at http://localhost:5555/. You need to generate some load in order to see data reported on SigNoz dashboard. Refresh the endpoint for 10-20 times, and wait for 2-3 mins.
And, congratulations! You have instrumented your sample Node.js app. You can now access the SigNoz dashboard at http://localhost:3301 to monitor your app for performance metrics.
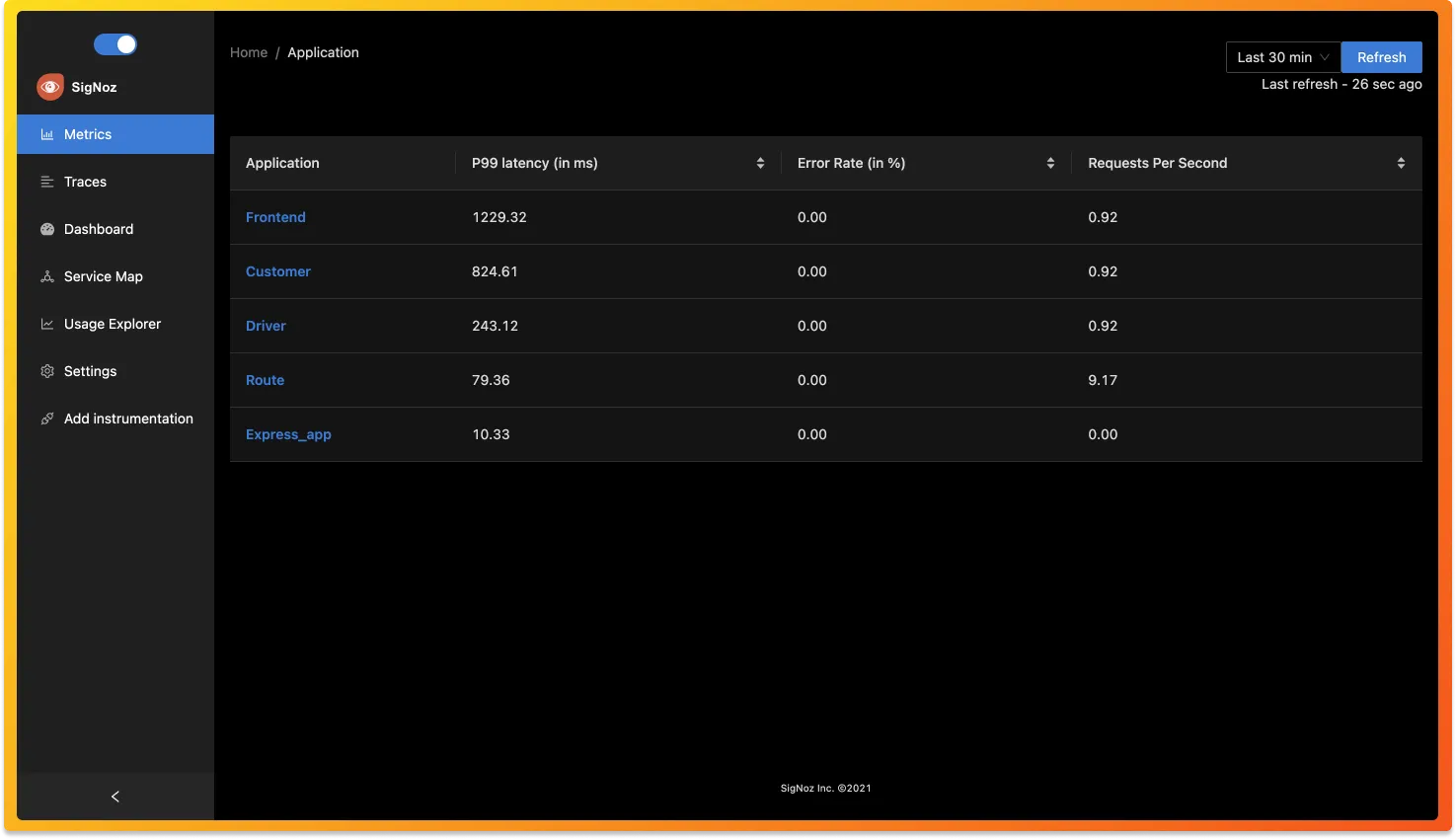
SigNoz is open-source, and a full-stack APM. It comes with charts of RED metrics and a seamless transition from metrics to traces.
Open-source tool to visualize telemetry data
SigNoz makes it easy to visualize metrics and traces captured through OpenTelemetry instrumentation.
SigNoz comes with out of box RED metrics charts and visualization. RED metrics stands for:
- Rate of requests
- Error rate of requests
- Duration taken by requests
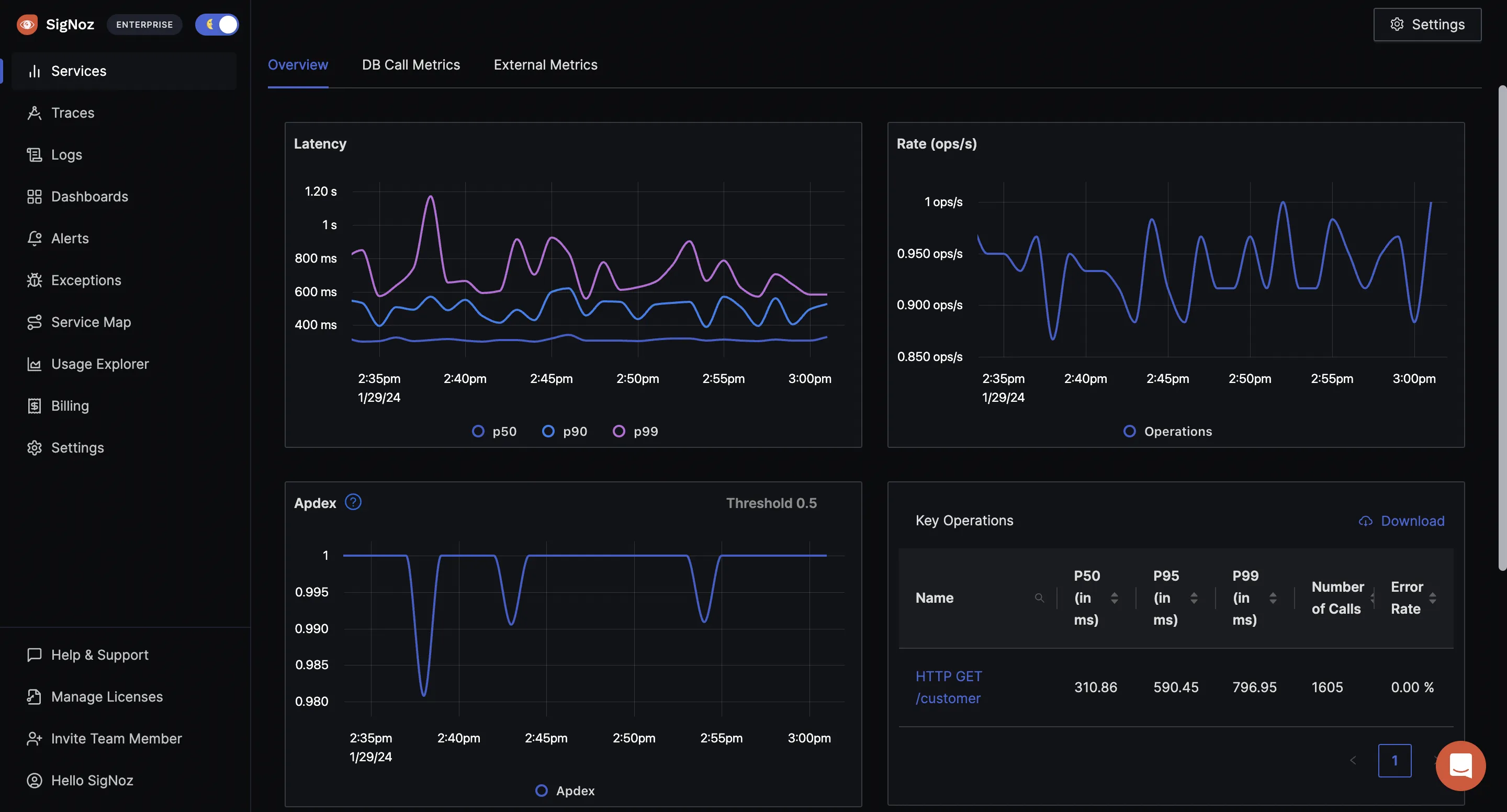
You can then choose a particular timestamp where latency is high to drill down to traces around that timestamp.
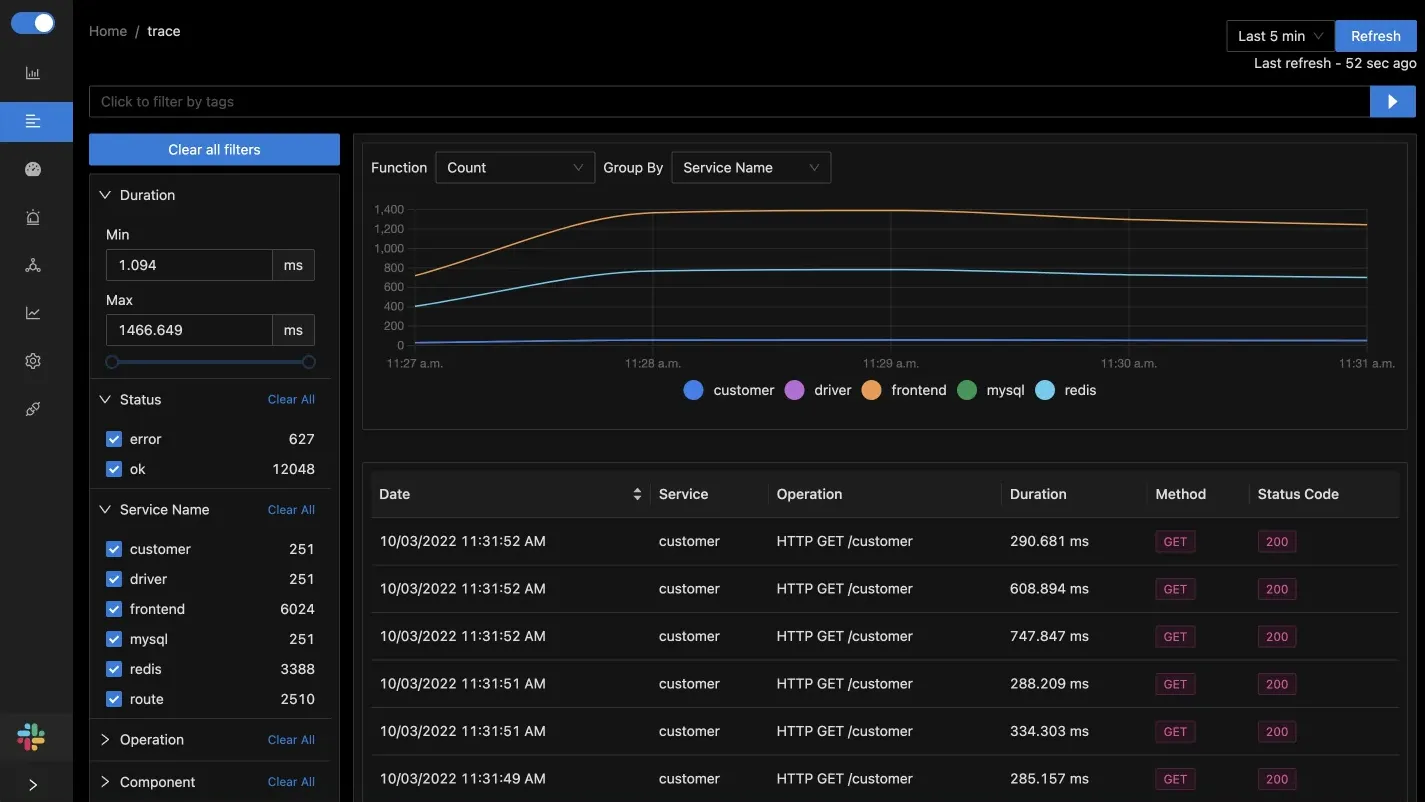
You can use flamegraphs to exactly identify the issue causing the latency.
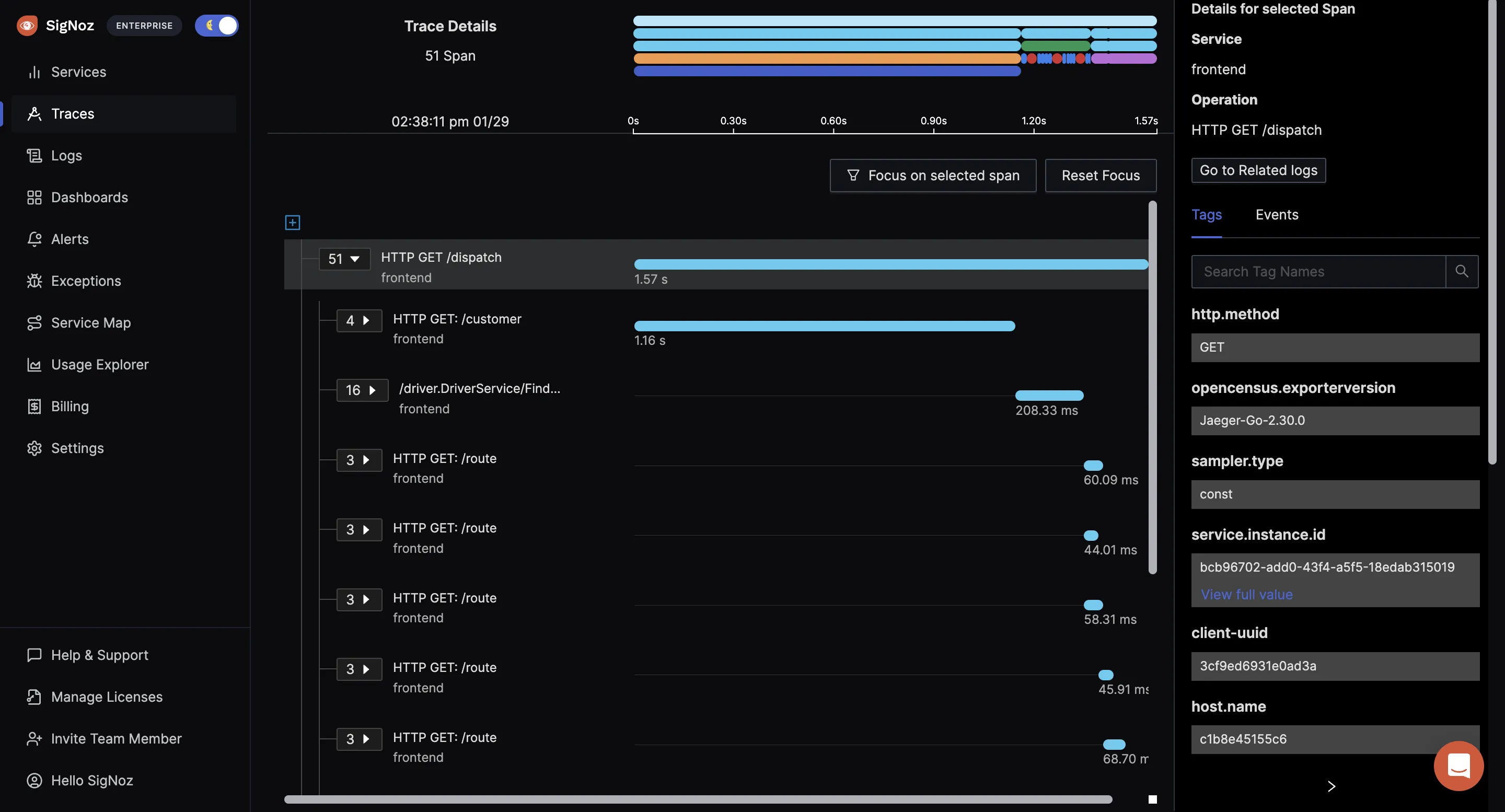
You can also build custom metrics dashboard for your infrastructure.
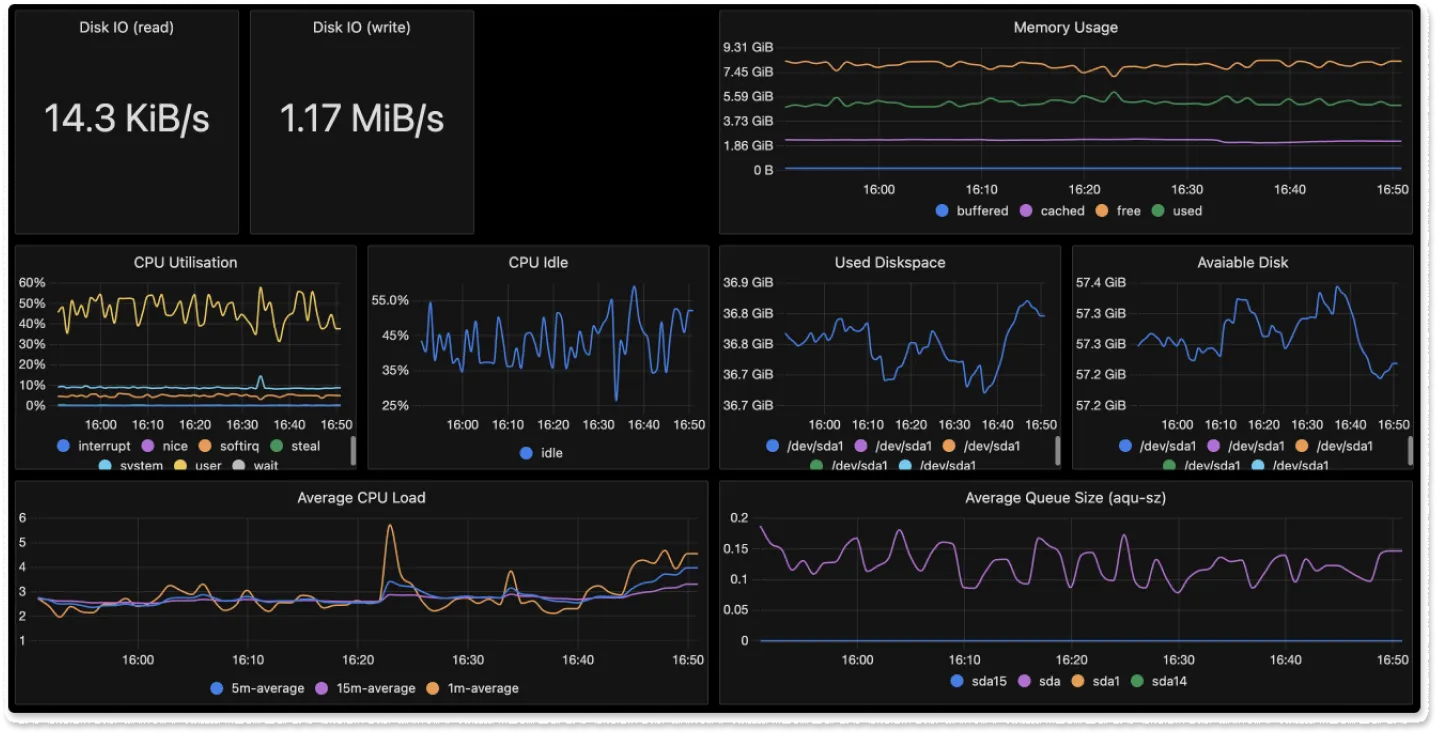
Conclusion
OpenTelemetry makes it very convenient to instrument your Express application. You can then use an open-source APM tool like SigNoz to analyze the performance of your app. As SigNoz offers a full-stack observability tool, you don't have to use multiple tools for your monitoring needs.
FAQs
What is OpenTelemetry and how does it relate to Express applications?
OpenTelemetry is an open-source standard for instrumentation that allows you to collect telemetry data (logs, metrics, and traces) from your applications. For Express applications, OpenTelemetry can be used to monitor performance, track requests, and gather insights into your app's behavior.
How do I set up OpenTelemetry for my Express application?
To set up OpenTelemetry for an Express application:
- Install necessary OpenTelemetry packages (SDK, auto-instrumentations, and exporter).
- Create a
tracing.js
file to initialize the OpenTelemetry SDK. - Configure the SDK with your desired service name and exporter URL.
- Run your application with the tracing file.
What is SigNoz and how does it work with OpenTelemetry?
SigNoz is an open-source, full-stack Application Performance Monitoring (APM) tool. It can be used as a backend to store and visualize telemetry data collected by OpenTelemetry from your Express application. SigNoz provides dashboards for metrics, distributed tracing, and custom visualizations.
What are the benefits of using OpenTelemetry with Express?
Benefits include:
- Standardized instrumentation across multiple languages and frameworks
- Vendor-agnostic approach, allowing you to switch between different observability backends
- Automatic instrumentation for many common libraries and frameworks
- Ability to collect detailed telemetry data for better understanding of application performance
How can I visualize the data collected by OpenTelemetry from my Express app?
You can visualize the data using an observability platform like SigNoz. SigNoz provides out-of-the-box visualizations for RED metrics (Rate, Error, and Duration), distributed traces, and allows you to create custom dashboards for your specific needs.
What are RED metrics and why are they important for monitoring Express apps?
RED metrics stand for Rate (requests per second), Error rate (percentage of failed requests), and Duration (latency of requests). These metrics are crucial for monitoring Express apps as they provide a quick overview of your application's health and performance, allowing you to identify issues rapidly.
How does OpenTelemetry differ from other monitoring solutions for Express.js?
OpenTelemetry stands out due to its vendor-neutral, open-source nature. It provides a standardized way to instrument applications, allowing you to switch between different backends without changing your instrumentation code.
Can OpenTelemetry be used with existing monitoring tools in my Express.js application?
Yes, OpenTelemetry is designed to work alongside existing monitoring solutions. Many popular monitoring tools offer OpenTelemetry integrations, allowing you to enhance your current setup.
What performance impact does OpenTelemetry have on my Express.js application?
While OpenTelemetry does introduce some overhead, it's generally minimal. The impact can be further reduced by implementing sampling strategies and optimizing your instrumentation.
How can I troubleshoot common issues when implementing OpenTelemetry in Express.js?
Common troubleshooting steps include:
- Verifying correct configuration of the SDK and exporters
- Checking for conflicting instrumentation libraries
- Ensuring proper context propagation in asynchronous code
- Reviewing logs for any OpenTelemetry-related errors or warnings
You can try out SigNoz by visiting its GitHub repo ๐
If you are someone who understands more from video, then you can watch the below video tutorial on the same with SigNoz.
If you have any questions or need any help in setting things up, join our slack community and ping us in #support
channel.
If you want to read more about SigNoz ๐
Golang Aplication Monitoring with OpenTelemetry and SigNoz
OpenTelemetry collector - full guide