In Java development, performance optimization is key. Java application profiling empowers developers by analyzing performance metrics like CPU usage, memory consumption, thread activity, and I/O operations. By identifying bottlenecks, profiling helps optimize applications for better efficiency and user experience. This guide will equip you with the knowledge and tools to master Java profiling, ensuring exceptional application performance.
What is Java Application Profiling?
Java application profiling is the process of analyzing your Java program's behavior and performance characteristics during runtime. While debugging targets functional issues, profiling targets performance inefficiencies that might not lead to immediate errors but degrade overall efficiency.
Profiling provides valuable insights that can lead to improved application responsiveness, reduced resource consumption, enhanced user experience, and lower operational costs. It is particularly useful in identifying performance bottlenecks in large-scale applications, optimizing memory usage, analyzing thread contention, and understanding I/O performance.
Profiling tools collect data on various aspects of your application, including method execution times, memory allocation patterns, thread activity, and I/O operations. This information can be used to pinpoint performance bottlenecks, identify memory leaks, and optimize resource usage.
Why is Java Application Profiling Important?
Profiling your Java applications is crucial for several reasons:
- Identify performance bottlenecks: Pinpoint slow methods or inefficient algorithms that impact your application's speed.
- Optimize resource usage: Improve how your application utilizes CPU, memory, and I/O resources.
- Enhance user experience: Faster, more responsive applications lead to happier users and better engagement.
- Reduce operational costs: Efficient code means lower resource requirements, potentially cutting cloud or hosting expenses.
Consider this scenario: Your e-commerce platform experiences slowdowns during peak hours. Without profiling, you might resort to costly hardware upgrades. However, profiling could reveal that a poorly optimized database query is the culprit, allowing you to fix the issue with a simple code change.
Key Dimensions of Java Application Profiling
To effectively profile your Java applications, you need to understand the four main dimensions of profiling:
CPU profiling analyzes method execution times to identify hotspots—areas of code that consume excessive processing power. For instance, in a real-world e-commerce application, you might discover that a product recommendation algorithm is consuming a significant portion of CPU resources. By optimizing this algorithm and reducing unnecessary method calls, you can greatly enhance application efficiency.
Memory profiling tracks object allocation and garbage collection patterns to detect memory leaks and optimize object management. This is particularly important in applications that handle large datasets or long-running processes, like data analytics platforms. Tuning garbage collection settings and optimizing object lifecycles can prevent out-of-memory errors and improve performance.
Thread profiling examines concurrent execution to identify issues like thread contention and deadlocks. In a high-traffic web server, for example, thread profiling might reveal that improper thread pool configurations are causing thread starvation, reducing responsiveness. By fine-tuning thread management, you can ensure smoother execution in multi-threaded environments.
I/O profiling monitors file and network operations, helping developers detect bottlenecks in database queries or file handling. For instance, an application that frequently accesses a database may experience high latency due to inefficient query designs. By optimizing these queries and improving network configurations, you can reduce I/O delays and boost overall application performance.
Essential Java Profiling Tools and Techniques
Java offers a variety of profiling tools, each with its strengths:
Built-in profiling tools:
- jconsole: A graphical tool for monitoring JVM performance
- jstat: A command-line tool for monitoring JVM statistics
- jmap: A utility for creating heap dumps
Open-source profilers:
- SigNoz: A modern, open-source observability tool that includes profiling capabilities for Java applications, ideal for continuous performance monitoring in distributed systems.
- VisualVM: A visual tool for monitoring and profiling Java applications
- YourKit: A powerful, feature-rich Java profiler
- JProfiler: An intuitive profiler with advanced features
IDE-integrated profiling tools:
- IntelliJ IDEA Profiler: Built-in profiling capabilities in the popular Java IDE
- Eclipse Profiler: The Eclipse Test & Performance Tools Platform (TPTP) provides basic profiling capabilities.
Cloud-based profiling solutions:
These tools offer profiling for distributed systems and microservices architectures
- AWS X-Ray: Distributed tracing on AWS.
- Google Cloud Profiler: Profiles CPU/memory in production.
Modern tools like SigNoz offer enhanced capabilities over traditional ones by providing real-time, distributed tracing, continuous profiling, and advanced analytics for microservices and cloud-native environments, which older tools often lack.
Setting Up Your Profiling Environment
To get started with profiling :
Enable necessary JVM flags:
java -agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005 YourApp
The -agentlib:jdwp=transport=dt_socket,server=y,suspend=n,address=5005
flag enables remote debugging and profiling. It uses JDWP for communication via a socket (dt_socket
), with the JVM acting as a server (server=y
). The application starts immediately without waiting for the debugger (suspend=n
), and the debugger connects on port 5005. This setup allows tools like VisualVM to profile the running application.
Integrate profiling tools into your development workflow:
- With Eclipse:
- Install the VisualVM Launcher plugin.
- Configure the JDK and VisualVM paths in Eclipse.
- Use VisualVM Launcher for run/debug actions. VisualVM will automatically open and profile your app.
- For CI/CD: Automate profiling with VisualVM's CLI, capturing performance snapshots during testing phases.
Follow best practices for minimal overhead profiling in production:
- Use Sampling Profilers: Tools like VisualVM use sampling to gather performance data with minimal impact. Sampling captures periodic snapshots of the application's state without adding significant overhead, as opposed to instrumentation-based profilers that monitor every method call.
- Profile in Short Bursts: Continuous profiling can negatively affect performance, especially in high-traffic environments. Instead, profile the application for short periods when necessary, such as during peak usage or after significant code changes.
- Implement Safeguards: Set up automatic safeguards to disable profiling if it impacts the performance beyond acceptable levels. VisualVM allows you to stop profiling or disconnect from the application easily once the required data is collected.
Quick Example with VisualVM
- Run your app with the JVM debugging flags.
- Open VisualVM (found in the JDK’s
bin
folder or installed separately). - In VisualVM, your app should appear under the Applications tab.
- Select your app and click on Profiler. From there, choose to profile the CPU, Memory, or Threads.
- Click Start to begin profiling and monitor your app's performance in real-time.
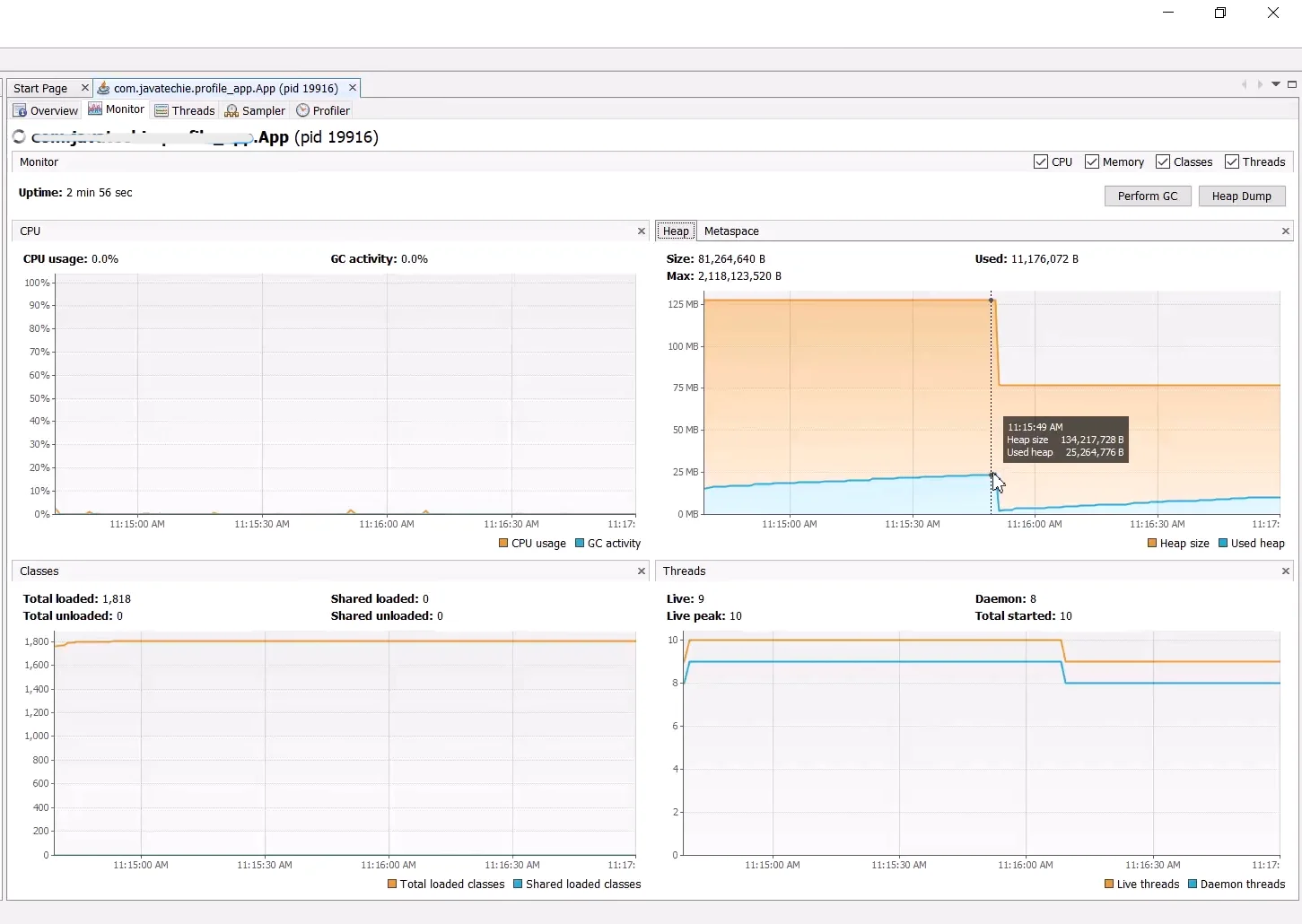
How to Perform CPU Profiling in Java
CPU profiling is often the first step in optimizing your Java application. Here's how to approach it and leverage Java's Just-In-Time (JIT) compilation to boost performance.
Identify CPU-intensive methods:
Use a profiler (such as JVisualVM, YourKit, or JProfiler) to generate a call graph or flame graph, which visualizes the CPU consumption of different methods.
Flame Graph to view CPU consumption for different methods The above image illustrates a flame graph generated by a Java profiler. It shows the structure of method invocations and the time spent in each method, with the width of each bar representing the time taken. This helps pinpoint which methods are consuming the most CPU.
Look for methods that consume a large percentage of CPU time
Analyze the call graph:
The call graph visualizes the relationships between method calls. Each method is represented as a block, and the blocks are stacked according to the method invocations. Here is an example of a call graph:
Call Graph Example In the provided flame graph, the method
org.gjt.jclasslib.structures.Structure.read(java.io.DataInput)
is consuming significant CPU time (14,443 µs). You can see the number of calls (419) and the percentage of self and total time spent in this method.Identify unnecessary or redundant method invocations by examining these relationships. Look for methods that take up excessive CPU time relative to their importance.
Leverage Java’s Just-In-Time (JIT) Compilation:
JIT compilation optimizes code during runtime by translating bytecode into native machine code, allowing frequently executed methods to run faster.
- Profile JIT behavior: Use tools like
XX:+PrintCompilation
or Java Flight Recorder (JFR) to monitor JIT-compiled methods. - Optimize for hot methods: Methods executed frequently (hot methods) are targeted by JIT for optimization. Refactor these to reduce unnecessary complexity, ensuring JIT compiles them efficiently.
- Watch for de-optimizations: Sometimes JIT reverts optimizations due to runtime conditions. Use JFR to spot these and adjust the code accordingly.
These JIT optimizations help reduce CPU time for high-frequency operations, boosting overall performance.
Optimize CPU usage:
- Refactor inefficient algorithms: Profiling tools can help you spot costly operations or unoptimized loops. Refactor these areas to reduce computational complexity.
- Use caching to avoid repeated computations: If you find the same method being called frequently with the same data, consider implementing caching to minimize redundant calculations.
- Leverage Java 8+ features: Java Streams,
Optional
, andCompletableFuture
can improve efficiency in handling data processing.
Case study: A team discovered their REST API was sluggish under load. CPU profiling revealed that a JSON serialization method was the bottleneck. By switching to a more efficient serialization library, they reduced response times by 40%.
Mastering Memory Profiling for Java Applications
Managing memory effectively is critical for ensuring that your Java application runs smoothly without slowing down or crashing. Memory profiling helps you monitor how memory is used, find leaks, and optimize the performance of your application.
Understand the Java Memory Model
Java applications use heap memory to store objects. Over time, the garbage collector (GC) cleans up unused objects to free up memory. However, if the GC runs too often, it can slow down your application. Using memory profilers like VisualVM or jconsole helps you track how memory is used and how the GC is usually working.
Detect Memory Leaks
A memory leak happens when objects no longer needed are still in memory because they haven't been properly released. This makes your application use more memory than necessary. To catch these leaks:
- Take heap dumps (snapshots of memory) using tools like VisualVM or JProfiler.
- Analyze these dumps to find objects that should have been removed but are still taking up space.
- The graph below demonstrates an issue where memory usage gradually increases (like stairs) due to objects remaining in memory and not being collected as garbage. Despite periodic GC events (represented by the dips in memory), the baseline keeps rising, indicating that objects are still being held in memory, which may eventually lead to OutOfMemoryError if left unchecked.
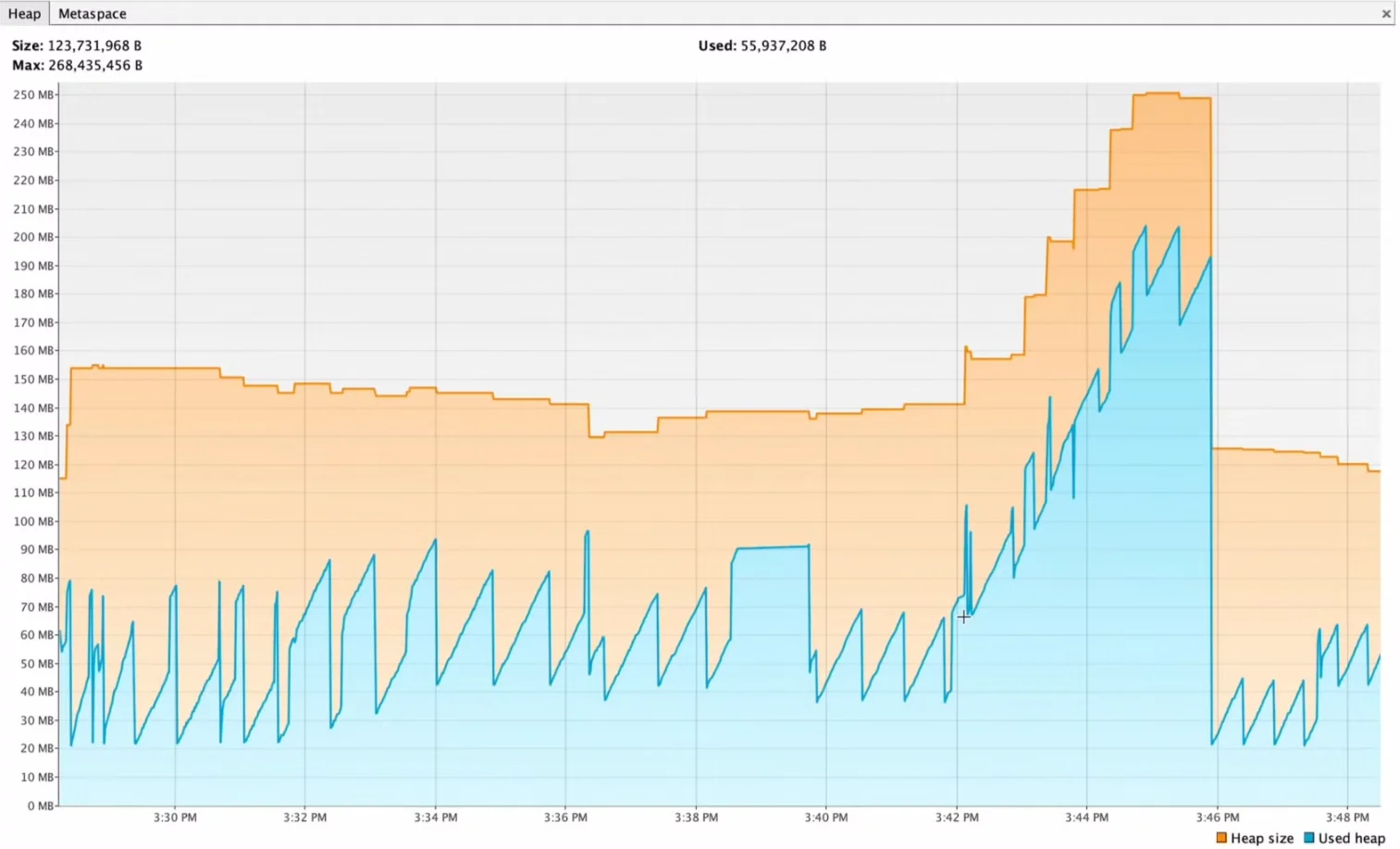
Optimize Object Allocation
Creating too many objects, especially in performance-critical areas like loops, can quickly use up memory. To optimize memory usage:
- Minimize object creation in frequently used sections of code.
- Use object pools to reuse objects instead of constantly creating new ones.
Reduce Memory Churn
Memory churn refers to creating and discarding objects too quickly, increasing the garbage collector's workload. To reduce this:
- Avoid String concatenation in loops. Instead, use StringBuilder to manage strings efficiently.
- Choose the right collection types to reduce memory usage (e.g., using an
ArrayList
instead of aLinkedList
when appropriate).
Tuning Garbage Collection
Frequent garbage collection (GC) can significantly impact application performance. When the JVM's garbage collector is busy reclaiming memory, it can lead to pauses and delays in application execution. Tuning GC settings can help minimize these pauses and optimize overall performance.
G1GC Tuning Example:
java -Xmx16g -Xms16g -XX:+UseG1GC -XX:G1HeapRegionSize=1024 -XX:MaxGCPauseMillis=200 -XX:ParallelGCThreads=8 -XX:InitiatingHeapOccupancyPercent=45 -jar your_application.jar
- XX:G1HeapRegionSize=1024: Sets the size of each heap region to 1024 MB.
- XX:MaxGCPauseMillis=200: Sets the target maximum pause time for GC cycles to 200 milliseconds.
- XX:ParallelGCThreads=8: Sets the number of threads used for parallel GC operations to 8.
- XX:InitiatingHeapOccupancyPercent=45: Specifies the percentage of heap occupancy at which the GC cycle is initiated.
By carefully tuning GC settings, you can significantly reduce the impact of garbage collection on your application's performance and improve its overall responsiveness.
Thread Profiling and Concurrency Analysis
Thread profiling is essential for identifying concurrency issues:
Identify thread contention and deadlocks:
- Run your application and use VisualVM or jconsole to take a thread dump.
- Example: In VisualVM, go to the Threads tab. There may be contention if you see multiple threads in the
BLOCKED
orWAITING
state. The threads represented with yellow color are in waiting state.
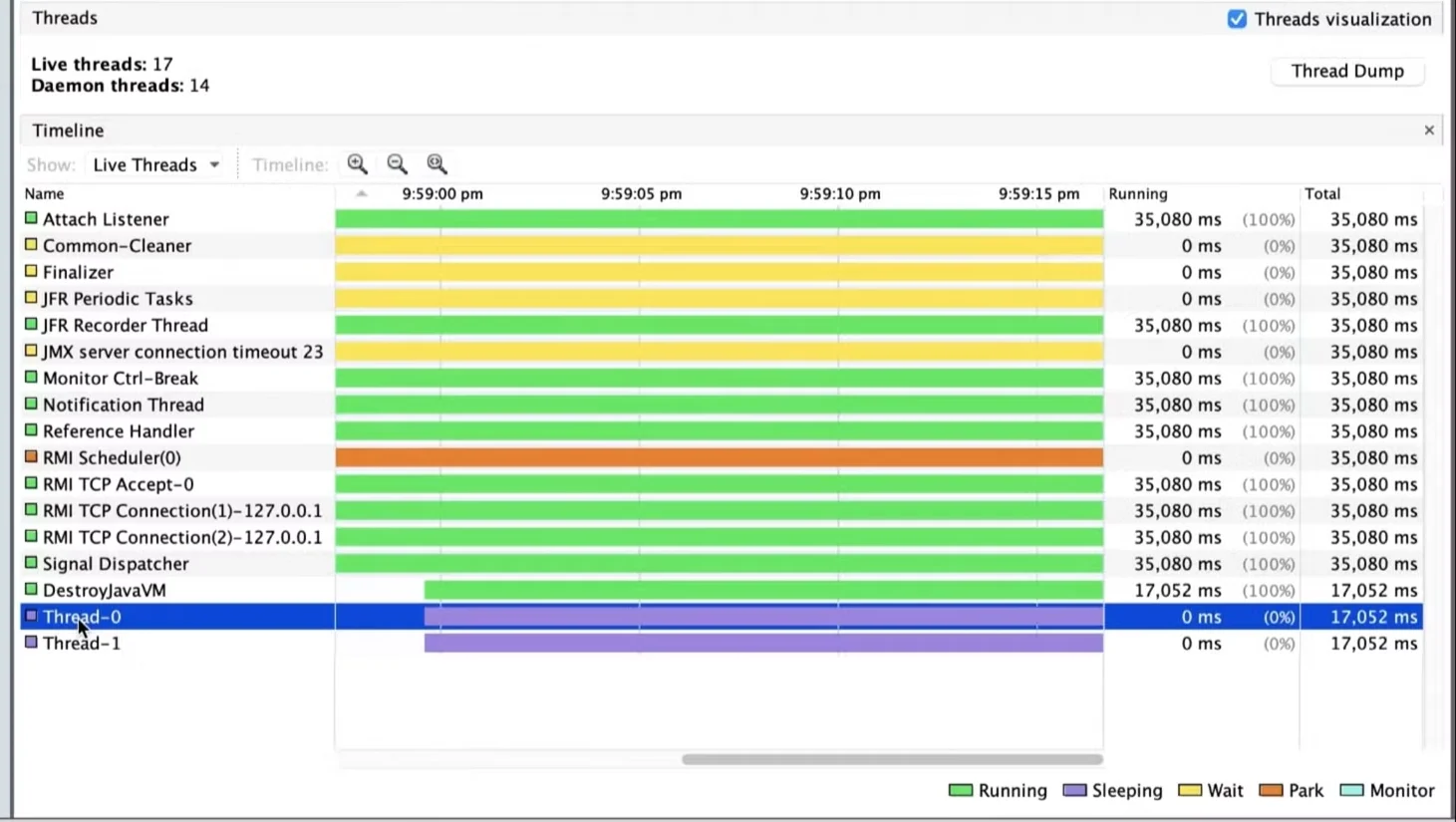
- To check for deadlocks, search for threads that are waiting on each other indefinitely.
- In VisualVM, you can identify nested synchronization issues by analyzing thread dumps and looking for threads stuck in the "BLOCKED" state, often due to multiple synchronized blocks or methods competing for the same lock. This visualization helps pinpoint where nested synchronization might be causing contention or deadlocks.
Analyze thread dumps:
- Use tools like jconsole or VisualVM to capture thread dumps
- Look for threads in BLOCKED or WAITING states
Optimize thread pool configurations:
- Adjust pool sizes based on profiling data
- Consider using different pool strategies for I/O-bound and CPU-bound tasks
Best practice: Write thread-safe code by favoring immutability, using concurrent collections, and leveraging higher-level concurrency utilities like ExecutorService and CompletableFuture.
Real-Time Monitoring and Continuous Profiling
For production environments, consider implementing always-on profiling:
Balance profiling depth with performance impact:
Continuous profiling can introduce overhead, so lightweight sampling techniques or adaptive profiling are essential to mitigate this. Adaptive profiling adjusts the level of detail based on the system's current load, ensuring that profiling remains efficient even under heavy usage.
Integrate profiling data with monitoring systems:
Profiling data should be fed into existing monitoring tools to provide a holistic view of application performance. By setting up alerts for sudden performance degradation, teams can quickly respond to issues without constant manual oversight.
Use SigNoz for comprehensive application performance monitoring:
SigNoz offers robust Java profiling capabilities while minimizing the overhead associated with continuous profiling. It provides a unified platform for tracing, metrics, and logs, making it easier to monitor distributed systems in production without impacting performance. With SigNoz, you can implement real-time monitoring and continuous profiling to track method-level performance, detect memory leaks, and correlate profiling data across services seamlessly.
Leveraging SigNoz for Java Application Profiling
SigNoz is a powerful, open-source application performance monitoring (APM) tool that offers exceptional capabilities for Java profiling. Here's an overview and a guide to get started:
Overview of SigNoz's Java Profiling Capabilities
- Continuous profiling with Minimal Overhead: Collect performance data over time with minimal impact on the application's performance.
- Integrated Distributed tracing: Track the flow of requests through microservices to identify bottlenecks or latency issues.
- Customizable dashboards: Adjust data visualization to meet specific needs and highlight key performance metrics.
Getting Started with SigNoz
- Install SigNoz: Follow the installation instructions on the SigNoz website.
- Add the SigNoz Java Agent:
Download the Java agent: Obtain the SigNoz Java agent from the SigNoz repository.
Add to your application's startup command: Modify your application's startup command to include the Java agent. For example: Replace
/path/to/signoz-java-agent.jar
with the actual path to the agent, andhttp://localhost:9000
with the address of your SigNoz backend.java -javaagent:/path/to/signoz-java-agent.jar -Dsignoz.collector.endpoint=http://localhost:9000 YourApplication
- Configure the Agent:
- Set agent properties: Configure the agent's properties (e.g., sampling rate, tracing level) as needed. Refer to the SigNoz documentation for detailed configuration options.
Analyzing Profiling Data with SigNoz
- Access the SigNoz UI: Open your web browser and navigate to the SigNoz UI (usually http://localhost:9000).
- Explore Profiling Data:
- Method-level performance: Use SigNoz's intuitive UI to explore method-level performance data, including execution times, call counts, and error rates.
- Distributed traces: Correlate profiling information with traces to understand the flow of requests across services and identify bottlenecks.
- Logs: Integrate logs with profiling data to gain a comprehensive view of your application's behavior.
For more detailed instructions and advanced features, refer to the official SigNoz documentation.
Advanced Profiling Techniques for Microservices and Distributed Systems
Profiling becomes more complex in distributed environments:
Implement distributed tracing:
Tools like OpenTelemetry can trace requests across microservices, providing insights into end-to-end latency and identifying cross-service bottlenecks. For instance, if a request is slow, distributed tracing can help you pinpoint which service or network call is causing the delay, allowing targeted optimizations.
Correlate profiling data across services:
By using consistent tracking IDs, you can correlate profiling data across different services and look for patterns in inter-service communication that affect performance. For example, if service A consistently waits for service B to respond, this may indicate a need for better load balancing or more efficient resource allocation in service B.
Optimize resource utilization in containerized environments:
In containerized environments, it’s important to profile both the application and the container’s resource usage.
For example, a microservice may experience high latency due to CPU throttling within its container, which can be resolved by increasing CPU limits or optimizing the service's CPU usage.
Similarly, if a Java service frequently crashes due to memory leaks, profiling can help detect the issue, and adjusting memory limits can prevent further crashes. Profiling can also uncover I/O bottlenecks caused by inefficient storage configurations, which can be improved by optimizing file handling or switching to faster storage solutions.
Best Practices for Effective Java Application Profiling
To make the most of your profiling efforts:
- Profile early and often: Integrate profiling into your development workflow to catch performance issues early.
- Use multiple tools: Different profiling tools offer different insights. Use a combination of tools for a comprehensive analysis.
- Analyze in context: Always consider the context in which your application runs. Profiling results can vary based on different environments and workloads.
- Establish baselines: Measure initial performance and define KPIs.
- Implement a systematic approach: Develop a profiling plan and iterate.
- Integrate into CI/CD: Incorporate profiling into your CI/CD pipelines.
- Minimize overhead: Be mindful of profiling overhead.
- Foster a performance-oriented culture: Encourage developers to be conscious of performance.
- Consider tools and techniques: Choose suitable tools and explore profiling techniques.
- Analyze and interpret data: Use profiling tools to analyze data and identify root causes.
Key Takeaways
- Java application profiling is essential for optimizing performance and resource usage
- Profiling covers CPU, memory, thread, and I/O analysis
- A combination of tools and techniques is necessary for comprehensive profiling
- Continuous profiling and monitoring, such as with SigNoz, are crucial for maintaining optimal performance
FAQs
What's the difference between sampling and instrumentation profiling?
Sampling profiling periodically collects data about the application's state, providing a statistical view of performance with minimal overhead. Instrumentation profiling modifies the bytecode to collect detailed data, offering more accurate results but with higher performance impact.
How often should I profile my Java application?
Profile your application regularly, especially after significant code changes or before major releases. Continuous profiling with tools like SigNoz should be considered for production environments to catch performance issues early.
Can profiling impact my application's performance in production?
Yes, profiling can introduce overhead. However, modern profiling tools like SigNoz are designed to minimize impact. Use sampling profilers and configure them to balance data collection with performance concerns.
How do I choose the right profiling tool for my project?
Consider factors like your application's architecture, your team's expertise, and specific performance concerns. For most projects, a combination of built-in JVM tools and a comprehensive APM solution like SigNoz will cover all bases.