SLF4J logger or Simple Logging Facade for Java is a straightforward abstraction layer for various logging frameworks in Java. It simplifies the process of logging by allowing you to integrate with any logging framework like Log4j, Logback, or JUL (java.util.logging) using just a single dependency. This means you can switch to a different logging framework at runtime or during deployment without changing your code.
Imagine you have multiple remote controls for different devices: one for your TV, another for your sound system, and another for your Blu-ray player. It’s a hassle to manage all of them, right? Now, think of SLF4J as that one smart remote that can control all your devices.
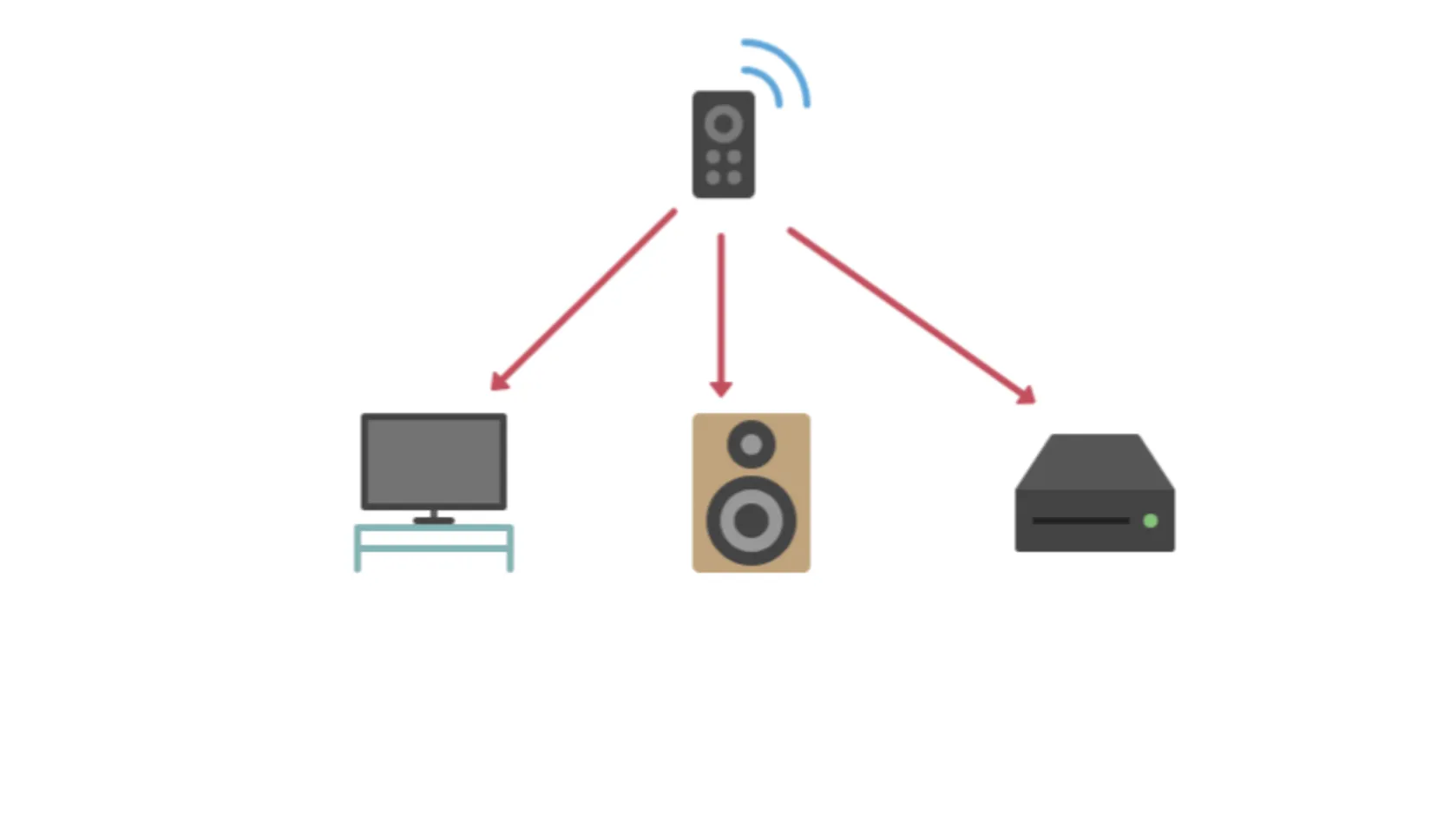
With SLF4J, you have a single, unified interface that works with various logging frameworks like Log4j, Logback, and JUL (java.util.logging).
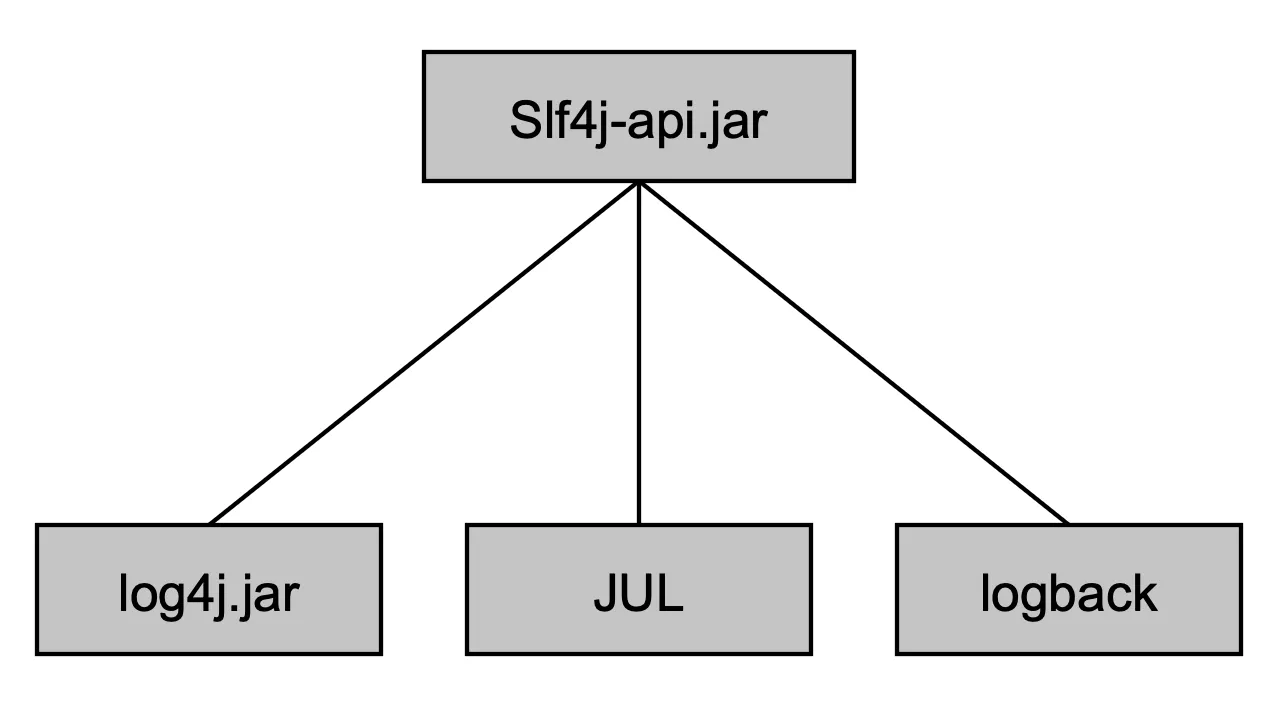
SLF4J Setup
Log4j2 Setup
To use Log4j2 with SLF4J, you need to include the appropriate dependencies and configure Log4j2. Here's a step-by-step guide on how to set up Log4j2 with SLF4J in a Java project:
- Add Dependencies
To use Log4j2 with SLF4J, you need to include the following dependencies in your project. If you are using Maven, add these to your pom.xml
file:
<dependencies>
<!-- SLF4J API -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.36</version>
</dependency>
<!-- Log4j2 Core -->
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.17.2</version>
</dependency>
<!-- Log4j2 SLF4J Binding -->
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-slf4j-impl</artifactId>
<version>2.17.2</version>
</dependency>
<!-- Log4j2 API -->
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-api</artifactId>
<version>2.17.2</version>
</dependency>
</dependencies
For Gradle, add these dependencies to your build.gradle
file:
dependencies {
implementation 'org.slf4j:slf4j-api:1.7.36'
implementation 'org.apache.logging.log4j:log4j-core:2.17.2'
implementation 'org.apache.logging.log4j:log4j-slf4j-impl:2.17.2'
implementation 'org.apache.logging.log4j:log4j-api:2.17.2'
}
- Configuration
Create a log4j2.xml
configuration file in the src/main/resources
directory. This file defines how Log4j2 will handle logging events.
Here's a basic example of a log4j2.xml
configuration:
<?xml version="1.0" encoding="UTF-8"?>
<Configuration status="WARN">
<Appenders>
<Console name="Console" target="SYSTEM_OUT">
<PatternLayout pattern="%d{HH:mm:ss.SSS} [%t] %-5level %logger{36} - %msg%n"/>
</Console>
<File name="File" fileName="logs/app.log">
<PatternLayout pattern="%d{ISO8601} [%t] %-5p %c - %m%n"/>
</File>
</Appenders>
<Loggers>
<Root level="info">
<AppenderRef ref="Console"/>
<AppenderRef ref="File"/>
</Root>
</Loggers>
</Configuration>
This configuration logs messages to both the console and a file named app.log
.
- Using SLF4J with Log4j2
You can now use SLF4J in your Java code to log messages, and they will be handled by Log4j2 according to the configuration.
The Logger
interface in the org.slf4j
package serves as the main entry point to the SLF4J API. It acts like the walkie-talkie you'll use to transmit your log messages. We will cover everything about the Logger
in the following section.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
private static final Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Application started");
logger.debug("Debugging information");
logger.error("An error occurred", new RuntimeException("Test error"));
}
}
Output:
[INFO] Application started
[ERROR] An error occurred
java.lang.RuntimeException: Test error
at MyApp.main(MyApp.java:9)
- Running the Application
When you run your application, Log4j2 will initialize according to the log4j2.xml
configuration file. The log messages will be output to the console and written to the app.log
file.
Logback Setup
Logback is a powerful and flexible logging framework often used as a replacement for Log4j. It is designed by the same developers who created Log4j and offers better performance and more features. Here’s a step-by-step guide on how to set up Logback with SLF4J in a Java project:
- Add Dependencies
To use Logback with SLF4J, you need to include the appropriate dependencies. If you are using Maven, add these to your pom.xml
file:
<dependencies>
<!-- SLF4J API -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.36</version>
</dependency>
<!-- Logback Classic Module -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.10</version>
</dependency>
<!-- Logback Core Module -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>1.2.10</version>
</dependency>
</dependencies>
For Gradle, add these dependencies to your build.gradle
file:
dependencies {
implementation 'org.slf4j:slf4j-api:1.7.36'
implementation 'ch.qos.logback:logback-classic:1.2.10'
implementation 'ch.qos.logback:logback-core:1.2.10'
}
- Configuration
Create a logback.xml
configuration file in the src/main/resources
directory. This file defines how Logback will handle logging events.
Here's a basic example of a logback.xml
configuration:
<configuration>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<appender name="FILE" class="ch.qos.logback.core.FileAppender">
<file>logs/app.log</file>
<encoder>
<pattern>%d{ISO8601} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="INFO">
<appender-ref ref="CONSOLE" />
<appender-ref ref="FILE" />
</root>
</configuration>
This configuration logs messages to both the console and a file named app.log
.
- Using SLF4J with Logback
You can now use SLF4J in your Java code to log messages, and they will be handled by Logback according to the configuration.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
private static final Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Application started");
logger.debug("Debugging information");
logger.error("An error occurred", new RuntimeException("Test error"));
}
}
Output:
[INFO] Application started
[ERROR] An error occurred
java.lang.RuntimeException: Test error
at MyApp.main(MyApp.java:9)
- Running the Application
When you run your application, Logback will initialize according to the logback.xml
configuration file. The log messages will be output to the console and written to the app.log
file.
JCL Setup
Jakarta Commons Logging (JCL) is another popular logging facade in the Java ecosystem. It provides a thin wrapper to various logging frameworks, such as Log4j, java.util.logging, and Logback. To set up JCL with SLF4J and a specific logging implementation, follow these steps:
- Add Dependencies
You need to include the appropriate dependencies in your project. If you are using Maven, add these to your pom.xml
file:
<dependencies>
<!-- JCL API -->
<dependency>
<groupId>commons-logging</groupId>
<artifactId>commons-logging</artifactId>
<version>1.2</version>
</dependency>
<!-- SLF4J JCL Bridge -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>jcl-over-slf4j</artifactId>
<version>1.7.36</version>
</dependency>
<!-- SLF4J API -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.36</version>
</dependency>
<!-- Logback Classic Module -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.10</version>
</dependency>
<!-- Logback Core Module -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>1.2.10</version>
</dependency>
</dependencies>
For Gradle, add these dependencies to your build.gradle
file:
dependencies {
implementation 'commons-logging:commons-logging:1.2'
implementation 'org.slf4j:jcl-over-slf4j:1.7.36'
implementation 'org.slf4j:slf4j-api:1.7.36'
implementation 'ch.qos.logback:logback-classic:1.2.10'
implementation 'ch.qos.logback:logback-core:1.2.10'
}
- Configuration
Create a logback.xml
configuration file in the src/main/resources
directory. This file defines how Logback will handle logging events. The configuration can be similar to the one used for Logback:
<configuration>
<appender name="CONSOLE" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<appender name="FILE" class="ch.qos.logback.core.FileAppender">
<file>logs/app.log</file>
<encoder>
<pattern>%d{ISO8601} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="INFO">
<appender-ref ref="CONSOLE" />
<appender-ref ref="FILE" />
</root>
</configuration>
- Using JCL with SLF4J
You can now use JCL in your Java code to log messages. The jcl-over-slf4j
bridge will route these logging calls to SLF4J, which will then be handled by Logback according to the configuration.
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
public class MyApp {
private static final Log logger = LogFactory.getLog(MyApp.class);
public static void main(String[] args) {
logger.info("Application started");
logger.debug("Debugging information");
logger.error("An error occurred", new RuntimeException("Test error"));
}
}
Output:
[INFO] Application started
[DEBUG] Debugging information
[ERROR] An error occurred
java.lang.RuntimeException: Test error
at MyApp.main(MyApp.java:11)
- Running the Application
When you run your application, the logging calls made using JCL will be routed through SLF4J and handled by Logback as configured in the logback.xml
file. The log messages will be output to the console and written to the app.log
file.
SLF4J Architecture
The Facade and its Bindings
SLF4J (Simple Logging Facade for Java) acts as an abstraction layer that decouples application code from the underlying logging frameworks, providing a consistent and flexible logging interface. This design allows developers to write logging code that is independent of the actual logging framework used in deployment. When working with SLF4J, you’ll frequently use several key classes and methods. Think of these as the essential tools in your developer toolkit. Therefore, there’s a need to assimilate the need for referenced API.
What is a Referenced API?
A referenced API (Application Programming Interface) is a set of tools, definitions, and protocols for building and integrating application software. It allows different software components to communicate with each other. In this context, SLF4J provides a standardized way to interact with various logging frameworks in Java, making it easier to log messages across different systems.
Logger Interface
The Logger interface in the org.slf4j package is your main entry point into the SLF4J API. It’s like the walkie-talkie you'll use to communicate your log messages.
- void debug(String msg) Logs a message at the DEBUG level. Use this when you need to record detailed information for debugging purposes.
Logger logger = LoggerFactory.getLogger(MyClass.class);
logger.debug("This is a debug message");
- void error(String msg) Logs a message at the ERROR level. This is for when something has gone wrong, and you need to capture the error details.
Logger logger = LoggerFactory.getLogger(MyClass.class);
logger.error("An error occurred");
- void info(String msg) Logs a message at the INFO level. Use this for general information about the application's running state.
Logger logger = LoggerFactory.getLogger(MyClass.class);
logger.info("This is an info message");
- void trace(String msg) Logs a message at the TRACE level. This is for even more fine-grained details than DEBUG, useful for tracing program execution.
Logger logger = LoggerFactory.getLogger(MyClass.class);
logger.trace("This is a trace message");
- void warn(String msg) Logs a message at the WARN level. Use this to indicate potential problems that aren’t necessarily errors.
Logger logger = LoggerFactory.getLogger(MyClass.class);
logger.warn("This is a warning message");
LoggerFactory Class
The LoggerFactory class in the org.slf4j package is like your factory that produces walkie-talkies. It generates Logger instances that you’ll use throughout your application.
- Logger getLogger(String name) Accepts a string (like a channel name for your walkie-talkie) and returns a Logger object. This allows you to create a logger with a specific name.
Logger logger = LoggerFactory.getLogger("MyLogger");
Profiler Class
The Profiler class in the org.slf4j package is a bit like a stopwatch for tracking how long tasks take. It’s known as a "poor man’s profiler," perfect for measuring performance without fancy tools.
- void start(String name) Starts a new named stopwatch and stops any previous ones. Think of this as starting a new lap on your stopwatch while stopping the previous lap.
Profiler profiler = new Profiler("MyProfiler");
profiler.start("task1");
- TimeInstrument stop() Stops the current stopwatch and returns a Time Instrument representing the elapsed time. It's like hitting stop on your stopwatch and noting the time.
TimeInstrument timeInstrument = profiler.stop();
- void setLogger(Logger logger) Associates a Logger with the Profiler. Imagine assigning your stopwatch results to a specific walkie-talkie channel.
profiler.setLogger(logger);
- void log() Logs the results of the current time instrument to the associated logger. It's like broadcasting your stopwatch results over your walkie-talkie.
profiler.log();
- void print() Prints the results of the current time instrument. This is like showing the stopwatch results on a display screen.
profiler.print();
Now, let's create a code example step by step, making sure we understand each line as we add it.
- Logger Initialization We need to create a Logger instance using
LoggerFactory
. This logger will be used to log messages from our application.
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
- Logging an Informational Message Let's log a message at the INFO level to indicate the application has started.
logger.info("Application started");
- Profiler Initialization We'll create a
Profiler
instance namedMyProfiler
. We then associate the logger with this profiler to log profiling results.
Profiler profiler = new Profiler("MyProfiler");
profiler.setLogger(logger);
- Starting the Profiler Starts the profiler for a task named
Task1
. This is like starting a new stopwatch for this specific task.
profiler.start("Task1");
- Simulating a Task We'll now simulate a task by making the thread sleep for 1 second. During this time, we log a debug message. If the task is interrupted, we log an error message.
try {
Thread.sleep(1000);
logger.debug("Task1 is running");
} catch (InterruptedException e) {
logger.error("Task interrupted", e);
}
- Stopping the Profiler and Logging the Results We need to stop the profiler and get the
TimeInstrument
, which contains the elapsed time forTask1
. We then log these results using the profiler.
TimeInstrument timeInstrument = profiler.stop();
profiler.log();
- Logging an Informational Message We'll log a message at the INFO level to indicate the application has finished.
logger.info("Application finished");
Here’s a small slf4j logger example to tie it all together:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.slf4j.profiler.Profiler;
import org.slf4j.profiler.TimeInstrument;
public class MyClass {
// Creating a Logger instance using LoggerFactory. This is like tuning our walkie-talkie to a specific channel named "MyClass".
private static final Logger logger = LoggerFactory.getLogger(MyClass.class);
public static void main(String[] args) {
// Logging an informational message indicating that the application has started.
logger.info("Application started");
// Creating a Profiler instance with a name "MyProfiler". This is like starting a stopwatch with a specific name.
Profiler profiler = new Profiler("MyProfiler");
// Associating the logger with the profiler. Now, the profiler will use this logger to log its messages.
profiler.setLogger(logger);
// Starting the profiler for a task named "Task1". This is like starting a new lap on our stopwatch.
profiler.start("Task1");
try {
// Simulating a task by making the thread sleep for 1 second. This represents some task we want to measure.
Thread.sleep(1000);
// Logging a debug message to indicate that "Task1" is running.
logger.debug("Task1 is running");
} catch (InterruptedException e) {
// Logging an error message if the task gets interrupted.
logger.error("Task interrupted", e);
}
// Stopping the profiler and getting the time instrument, which represents the elapsed time for "Task1".
TimeInstrument timeInstrument = profiler.stop();
// Logging the results of the profiler to the associated logger. This broadcasts our stopwatch results over our walkie-talkie.
profiler.log();
// Logging an informational message indicating that the application has finished.
logger.info("Application finished");
}
}
Output:
[INFO] Application started
[INFO] MyProfiler - Task1: 1,000,123 microseconds
[INFO] Application finished
Understanding Logging Messages at Different Levels with SLF4J
Imagine you are a journalist covering a big event. You have different types of notes for different kinds of information: urgent alerts, important warnings, general news, detailed background information, and minute-by-minute updates. In programming, logging works similarly, where messages are categorized by their importance or verbosity. SLF4J supports logging at five different levels:
Type | Description |
---|---|
Error | Critical issues that need immediate attention, like a fire alarm. |
Warn | Important warnings that should be addressed soon, like a low battery warning. |
Info | General informational messages about the program's progress. |
Debug | Detailed information for diagnosing problems, like a detailed report on your workday. |
Trace | Very detailed information for tracing the program execution, like a minute-by-minute diary entry. |
Let's look at slf4j logger example example that demonstrates logging at different levels:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
// Creating a logger instance for the MyApp class.
final static Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
// Logging messages at different levels.
logger.error("Error level log"); // Critical issue
logger.warn("Warn level log"); // Important warning
logger.info("Info level log"); // General information
logger.debug("Debug level log"); // Detailed debug information
logger.trace("Trace level log"); // Very detailed trace information
}
}
Output:
Error level log
Warn level log
Info level log
Note: Depending on your logging configuration, 'Debug level log' and 'Trace level log' might not be shown.
Understanding Log Destinations in Java Logging Frameworks
Imagine you're a writer who needs to keep track of notes, drafts, and final versions of your articles. You have different ways (destinations) to store these notes: in a notebook, on your computer, by emailing them to yourself, or even by recording voice notes. Similarly, Java logging frameworks offer various handlers to direct where your log messages go. Let's explore these handlers using simple analogies.
1. ConsoleHandler
Analogy: Imagine reading your notes out loud to yourself.
- Description: This handler outputs log messages directly to the console (the screen). There's no way to save these messages once the console session ends.
- Use Case: Ideal for quick debugging or testing.
Example Configuration:
handlers=java.util.logging.ConsoleHandler
2. FileHandler
Analogy: Writing your notes in a notebook.
- Description: This handler writes logs to a file. You can configure it to create new files when the current file reaches a certain size (like starting a new notebook when one is full).
- Use Case: Useful for long-term logging and record-keeping.
Example Configuration:
handlers=java.util.logging.FileHandler
java.util.logging.FileHandler.limit = 34534 # Maximum file size in bytes
java.util.logging.FileHandler.append = false # Do not append to existing file
java.util.logging.FileHandler.pattern = log.%u.txt # Log file naming pattern
3. SocketHandler
Analogy: Calling a friend to dictate your notes.
- Description: This handler sends log messages to a network socket, which can be picked up by another service or application. Typically, logs are formatted in XML.
- Use Case: Useful for centralized logging in distributed systems.
Example Configuration:
handlers=java.util.logging.SocketHandler
4. StreamHandler
Analogy: Writing your notes on a blank piece of paper, which can later be placed anywhere (notebook, computer, etc.).
- Description: This handler writes logs to an
OutputStream
, which can then be directed to various destinations. - Use Case: Acts as a foundation for creating custom handlers.
Example Configuration:
handlers=java.util.logging.StreamHandler
5. MemoryHandler
Analogy: Storing your notes in your memory until you decide to write them down in your notebook.
- Description: This handler stores log messages in an in-memory buffer. It writes to a more permanent storage only when certain conditions are met, such as when a log message of a specified level arrives.
- Use Case: Useful for high-performance applications where you want to defer the cost of writing logs until necessary.
Example Configuration:
handlers=java.util.logging.MemoryHandler
Code
Let's look at how we can set up and use these handlers in a Java application:
Sure! Let's go through the code provided and explain the expected output at each step, along with an explanation.
Here's the example code again:
import java.util.logging.*;
public class MyApp {
private static final Logger logger = Logger.getLogger(MyApp.class.getName());
public static void main(String[] args) {
try {
// ConsoleHandler: Logs to the console
ConsoleHandler consoleHandler = new ConsoleHandler();
logger.addHandler(consoleHandler);
// FileHandler: Logs to a file with specified settings
FileHandler fileHandler = new FileHandler("app.log", 34534, 1, false);
logger.addHandler(fileHandler);
// SocketHandler: Logs to a network socket (assuming the socket server is running)
SocketHandler socketHandler = new SocketHandler("localhost", 5000);
logger.addHandler(socketHandler);
// StreamHandler: Logs to an OutputStream (e.g., ByteArrayOutputStream)
StreamHandler streamHandler = new StreamHandler(System.out, new SimpleFormatter());
logger.addHandler(streamHandler);
// MemoryHandler: Logs to memory and flushes to a primary handler based on conditions
MemoryHandler memoryHandler = new MemoryHandler(consoleHandler, 100, Level.WARNING);
logger.addHandler(memoryHandler);
// Logging messages at different levels
logger.severe("Severe level log");
logger.warning("Warning level log");
logger.info("Info level log");
logger.fine("Fine level log");
logger.finer("Finer level log");
} catch (Exception e) {
e.printStackTrace();
}
}
}
- ConsoleHandler: This handler logs messages to the console.
- FileHandler: This handler logs messages to a file named
app.log
with a maximum size of 34534 bytes. If the file exceeds this size, a new log file is created.append
is set tofalse
, meaning it will overwrite the existing file if it exists. - SocketHandler: This handler sends log messages to a network socket at
localhost
on port5000
.- Note: For this to work, a socket server needs to be running at this address and port.
- StreamHandler: This handler logs messages to an
OutputStream
, which in this case is the console (standard output), using aSimpleFormatter
. - MemoryHandler: This handler logs messages to an in-memory buffer and flushes them to
ConsoleHandler
when the buffer reaches 100 records or when a log message ofLevel.WARNING
or higher is logged.
Logging Messages
logger.severe("Severe level log");
logger.warning("Warning level log");
logger.info("Info level log");
logger.fine("Fine level log");
logger.finer("Finer level log");
Output:
ConsoleHandler and StreamHandler Output:
- Both
ConsoleHandler
andStreamHandler
will output all the log messages to the console, formatted by theSimpleFormatter
.
Jun 02, 2024 12:34:56 PM MyApp main SEVERE: Severe level log Jun 02, 2024 12:34:56 PM MyApp main WARNING: Warning level log Jun 02, 2024 12:34:56 PM MyApp main INFO: Info level log
- Messages with
Level.FINE
andLevel.FINER
may not appear on the console if the default logging level is set higher thanFINE
.
- Both
FileHandler Output:
- All the log messages will be written to
app.log
with similar formatting.
Jun 02, 2024 12:34:56 PM MyApp main SEVERE: Severe level log Jun 02, 2024 12:34:56 PM MyApp main WARNING: Warning level log Jun 02, 2024 12:34:56 PM MyApp main INFO: Info level log
- Messages with
Level.FINE
andLevel.FINER
will be logged to the file if the logging level allows it.
- All the log messages will be written to
SocketHandler Output:
- Logs will be sent to the network socket at
localhost:5000
. The exact output depends on the receiving application.
- Logs will be sent to the network socket at
MemoryHandler Output:
MemoryHandler
will buffer log messages and flush them toConsoleHandler
when the buffer reaches 100 entries or when aWARNING
or higher-level message is logged.
- Logging Levels: Ensure the logging configuration allows for the desired log levels to be displayed or saved.
- Handlers Configuration: The behavior of each handler (like
FileHandler
limits,SocketHandler
connections) can be adjusted as needed. - Error Handling: The try-catch block ensures any exceptions during logging setup are caught and handled.
By using these handlers, you can effectively manage how and where your application's log messages are recorded, much like choosing different methods for storing your notes based on your needs.
- ConsoleHandler: Like reading notes out loud.
- FileHandler: Like writing notes in a notebook.
- SocketHandler: Like dictating notes over the phone.
- StreamHandler: Like writing notes on a blank paper to be stored later.
- MemoryHandler: Like remembering notes until you decide to write them down.
By understanding and using these handlers, you can effectively manage where and how your logs are stored, much like organizing your notes in the most efficient way possible.
Features of SLF4J
SLF4J (Simple Logging Facade for Java) provides several key features that make it a versatile and powerful logging framework for Java applications. Below are the main features of SLF4J, along with explanations and examples for each:
- Logging Abstraction
Feature: SLF4J provides a unified logging API that decouples application code from specific logging frameworks.
Example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
private static final Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("This is an info message");
}
}
- Binding Flexibility
Feature: SLF4J allows the use of various underlying logging frameworks by changing the binding at deployment time.
Example:
- Using Logback as the underlying framework:
- Add
logback-classic.jar
andlogback-core.jar
to the classpath.
- Add
- Using Log4j:
- Add
slf4j-log4j12.jar
andlog4j.jar
to the classpath.
- Add
- Parameterization
Feature: SLF4J supports parameterized logging messages, which avoids the cost of string concatenation when the log level is disabled.
Example:
logger.debug("User {} has logged in", userId);
- Marker Support
Feature: Markers are special tags that enrich log statements with additional information, which can be useful for filtering or routing logs.
Example:
import org.slf4j.Marker;
import org.slf4j.MarkerFactory;
Marker securityMarker = MarkerFactory.getMarker("SECURITY");
logger.info(securityMarker, "Security event for user {}", userId);
Best Practices with @Slf4j and Logging
Choose the Right Logging Framework
Selecting the right logging framework is crucial. SLF4J is highly recommended as it provides a simple abstraction over various logging frameworks like Log4j, Logback, and java.util.logging (JUL).
Example:
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
private static final Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("Application started");
}
}
Output:
INFO MyApp - Application started
The INFO level message "Application started" is logged, indicating that the application has started.
Log at Appropriate Levels
Use the correct log level for messages to avoid cluttering your logs. Here’s a quick guide:
Example:
public void processOrder(Order order) {
logger.debug("Processing order: {}", order.getId());
if (order == null) {
logger.error("Order is null");
return;
}
logger.info("Order processed successfully: {}", order.getId());
}
Output (for a non-null order):
DEBUG MyApp - Processing order: 12345
INFO MyApp - Order processed successfully: 12345
Output (for a null order):
ERROR MyApp - Order is null
The log shows different levels of messages based on the condition of the order. If the order is non-null, both DEBUG and INFO messages are logged. If the order is null, an ERROR message is logged.
Avoid Logging Sensitive Information
Be mindful of logging sensitive data like passwords, credit card numbers, or personal information. Always sanitize your logs to protect user privacy.
Example:
logger.debug("User login: [username={}, password=]", username);
Output:
DEBUG MyApp - User login: [username=johndoe, password=]
Sensitive information such as the password is masked with to avoid exposing it in the logs.
Use Structured Logging
Structured logging makes it easier to search and analyze logs. Use key-value pairs instead of plain text messages.
Example:
logger.info("User action", kv("userId", userId), kv("action", "login"));
Output:
INFO MyApp - User action userId=johndoe action=login
The log message includes structured key-value pairs, making it easier to parse and analyze.
Rotate Log Files
Set up log rotation to manage log file sizes and avoid running out of disk space.
Example (with Logback):
<configuration>
<appender name="FILE" class="ch.qos.logback.core.rolling.RollingFileAppender">
<file>logs/app.log</file>
<rollingPolicy class="ch.qos.logback.core.rolling.TimeBasedRollingPolicy">
<fileNamePattern>logs/app.%d{yyyy-MM-dd}.log</fileNamePattern>
<maxHistory>30</maxHistory>
</rollingPolicy>
<encoder>
<pattern>%d{HH:mm:ss.SSS} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="FILE" />
</root>
</configuration>
Output:
# logs/app.log (current log file)
INFO MyApp - Application started
# logs/app.2023-06-01.log (log file from previous day)
INFO MyApp - Application started
The log files are rotated daily and saved with a timestamp. Only logs from the last 30 days are kept.
Use Contextual Information
Include contextual information like user ID, session ID, or transaction ID to make logs more meaningful.
Example:
MDC.put("userId", userId);
logger.info("User logged in");
// Remember to clear MDC after logging
MDC.clear();
Output:
INFO MyApp - User logged in userId=johndoe
The log message includes contextual information (userId) which is helpful for tracking actions performed by specific users.
Avoid Logging in Tight Loops
Logging inside tight loops can significantly degrade performance. Log critical information only.
Example:
for (int i = 0; i < items.size(); i++) {
if (i % 100 == 0) {
logger.debug("Processing item {}", i);
}
processItem(items.get(i));
}
Output:
DEBUG MyApp - Processing item 0
DEBUG MyApp - Processing item 100
DEBUG MyApp - Processing item 200
Instead of logging every item, the log message is only recorded every 100 items, reducing the logging overhead.
Test Your Logging Configuration
Always test your logging configuration to ensure it behaves as expected in different environments (development, testing, production).
Example:
public static void main(String[] args) {
// Test logging configuration
logger.info("Starting application");
try {
// Simulate application logic
} catch (Exception e) {
logger.error("Unexpected error occurred", e);
}
logger.info("Application finished");
}
Output:
INFO MyApp - Starting application
INFO MyApp - Application finished
The log messages confirm that the application has started and finished successfully. Any unexpected errors would be logged with an ERROR
message.
Monitor Logs with an Observability Platform
Implementing a robust log monitoring system is crucial for maintaining the health and performance of your Java applications. An observability platform can help you collect, analyze, and manage logs efficiently, providing valuable insights into your application's behavior.
This can be done with dedicated paid or open-source software like SigNoz. Refer to the article Collecting Application Logs Using OTEL Java Agent to collect application logs.
By incorporating log monitoring with an observability platform into your logging strategy, you can enhance your ability to detect, diagnose, and resolve issues in your Java applications quickly and efficiently.
For a more in-depth understanding of log monitoring, you can read the Log Monitoring 101 Detailed Guide.
Conclusion
- SLF4J logger serves as a crucial component in modern Java applications.
- It provides a powerful and flexible logging abstraction.
- SLF4J logger seamlessly integrates with various logging frameworks like Logback, Log4j, and java.util.logging.
- Features such as parameterized logging, markers, and Mapped Diagnostic Context (MDC) enhance logging capabilities.
- Its thread-safe design ensures reliable operation in multithreaded environments.
- Widely adopted in frameworks like Spring Boot, SLF4J logger promotes unified logging practices.
- The abstraction of underlying logging implementations simplifies configuration and management.
FAQs
What is SLF4J logger in Java?
SLF4J (Simple Logging Facade for Java) is a logging facade that provides a simple abstraction for various logging frameworks (such as Log4j, Logback, and java.util.logging). It allows developers to write logging code that is independent of the underlying logging implementation, making it easier to switch between different logging frameworks without changing the application code.
What is SLF4J vs Log4j?
- SLF4J: It is a facade or abstraction layer for various logging frameworks. SLF4J does not perform the actual logging; instead, it routes the logging calls to the underlying logging framework specified at runtime (e.g., Log4j, Logback, java.util.logging).
- Log4j: It is a specific logging framework that provides the actual logging functionality, including configuration, log message formatting, and log message output. Log4j can be used directly or via SLF4J as an underlying logging implementation.
How to get logger from SLF4J?
To obtain a logger instance from SLF4J, you use the LoggerFactory
class provided by SLF4J:
javaCopy code
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApp {
private static final Logger logger = LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
logger.info("This is an info message");
}
}
What are the advantages of SLF4J logger?
- Abstraction Layer: Allows you to decouple your application code from the underlying logging framework, making it easier to switch between different logging frameworks.
- Parameterized Logging: Supports parameterized logging messages, which avoid the cost of string concatenation when the log level is disabled.
- Markers: Provides the ability to attach additional information to log messages, which can be used for filtering and routing.
- Compatibility: Works with a wide range of logging frameworks, including Log4j, Logback, java.util.logging, and others.
Can I use SLF4J without Log4j?
Yes, you can use SLF4J without Log4j. SLF4J supports multiple underlying logging frameworks. For example, you can use SLF4J with Logback, java.util.logging, or any other supported framework by including the appropriate SLF4J binding.
Why logger is used in Java?
Loggers are used in Java to record information about the application's runtime behavior. This information can be critical for debugging, monitoring, and maintaining the application. Logging helps developers understand the flow of the application, capture errors and exceptions, and analyze performance issues.
Why use SLF4J in Spring Boot?
Spring Boot uses SLF4J as its logging facade for several reasons:
- Flexibility: It allows developers to choose their preferred logging framework (e.g., Logback, Log4j2) while providing a consistent logging API.
- Unified Logging: It ensures that all logging in the Spring ecosystem can be routed through a single logging framework, simplifying configuration and management.
- Dependency Management: Spring Boot's default logging setup uses Logback, which works seamlessly with SLF4J.
Do I need SLF4J for Log4j2?
No, you do not strictly need SLF4J to use Log4j2, as Log4j2 can be used directly. However, using SLF4J provides the benefit of abstraction, allowing you to easily switch to a different logging framework if needed without changing the application code.
Is SLF4J thread-safe?
Yes, SLF4J is designed to be thread-safe. The underlying logging frameworks it delegates to (such as Logback and Log4j) are also thread-safe, ensuring that logging operations are safe to use in multithreaded applications.
Is SLF4J safe?
Yes, SLF4J is considered safe to use in applications. It is widely used in the Java ecosystem and is designed to be thread-safe and efficient. However, as with any library, it is essential to use it correctly and keep dependencies up-to-date to avoid potential vulnerabilities.
What is the difference between Logstash and SLF4J?
- SLF4J: A logging facade that provides a simple abstraction for various logging frameworks.
- Logstash: An open-source data processing pipeline that ingests, transforms, and sends data (including logs) to various destinations (e.g., Elasticsearch, files, databases). Logstash is part of the Elastic Stack (formerly ELK Stack) and is used for log management and analysis.
What does SLF4J annotation do?
SLF4J itself does not provide annotations. However, some frameworks (such as Lombok) offer annotations that can be used in conjunction with SLF4J to simplify logging. For example, Lombok's @Slf4j
annotation automatically generates a logger field in the annotated class:
javaCopy code
import lombok.extern.slf4j.Slf4j;
@Slf4j
public class MyApp {
public static void main(String[] args) {
log.info("This is an info message");
}
}
This @Slf4j
annotation from Lombok generates the following code:
javaCopy code
public class MyApp {
private static final org.slf4j.Logger log = org.slf4j.LoggerFactory.getLogger(MyApp.class);
public static void main(String[] args) {
log.info("This is an info message");
}
}