Nextjs is a production-ready React framework for building single-page web applications. It enables you to build fast and user-friendly static websites, as well as web applications using Reactjs. Using OpenTelemetry Nextjs libraries, you can set up end-to-end tracing for your Nextjs applications.
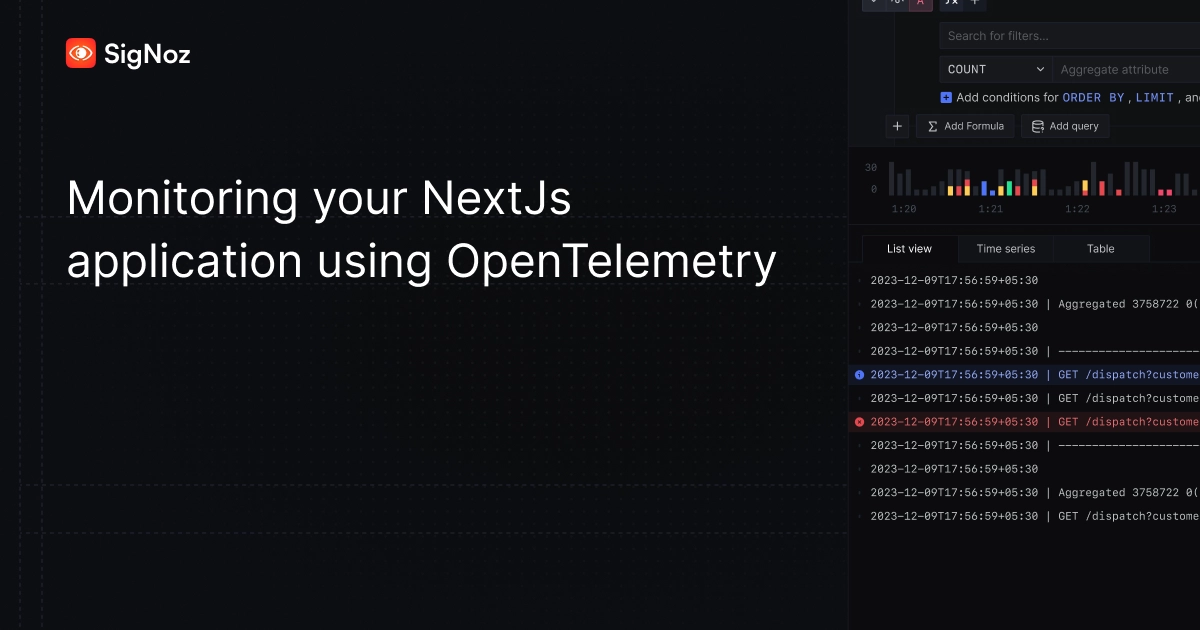
Nextjs has its own monitoring feature, but it is only limited to measuring the metrics like core web vitals and real-time analytics of the application. It doesn’t have end-to-end tracing, monitoring the database calls, etc. That’s where OpenTelemetry comes in.
OpenTelemetry is an open-source standard under the Cloud Native Computing Foundation (CNCF) used for instrumenting applications for generating telemetry data. You can monitor your Nextjs application using OpenTelemetry and a tracing backend of your choice.
What is OpenTelemetry Nextjs instrumentation? Instrumentation is the process of enabling your application code to generate telemetry data like logs, metrics, and traces. Using OpenTelemetry Nextjs client libraries, you can generate end-to-end tracing data from your Nextjs application.
OpenTelemetry provides client libraries to take care of instrumentation. You then need to send the collected data to an analysis backend. In this tutorial, we will be using SigNoz to store and visualize the telemetry data collected by OpenTelemetry from the sample Nextjs application.
Before we demonstrate how to implement the OpenTelemetry Nextjs libraries, let’s have a brief overview of OpenTelemetry.
What is OpenTelemetry?
OpenTelemetry is an open-source vendor-agnostic set of tools, APIs, and SDKs used to instrument applications to create and manage telemetry data(logs, metrics, and traces). It aims to make telemetry data(logs, metrics, and traces) a built-in feature of cloud-native software applications.
The telemetry data is then sent to an observability tool for storage and visualization.
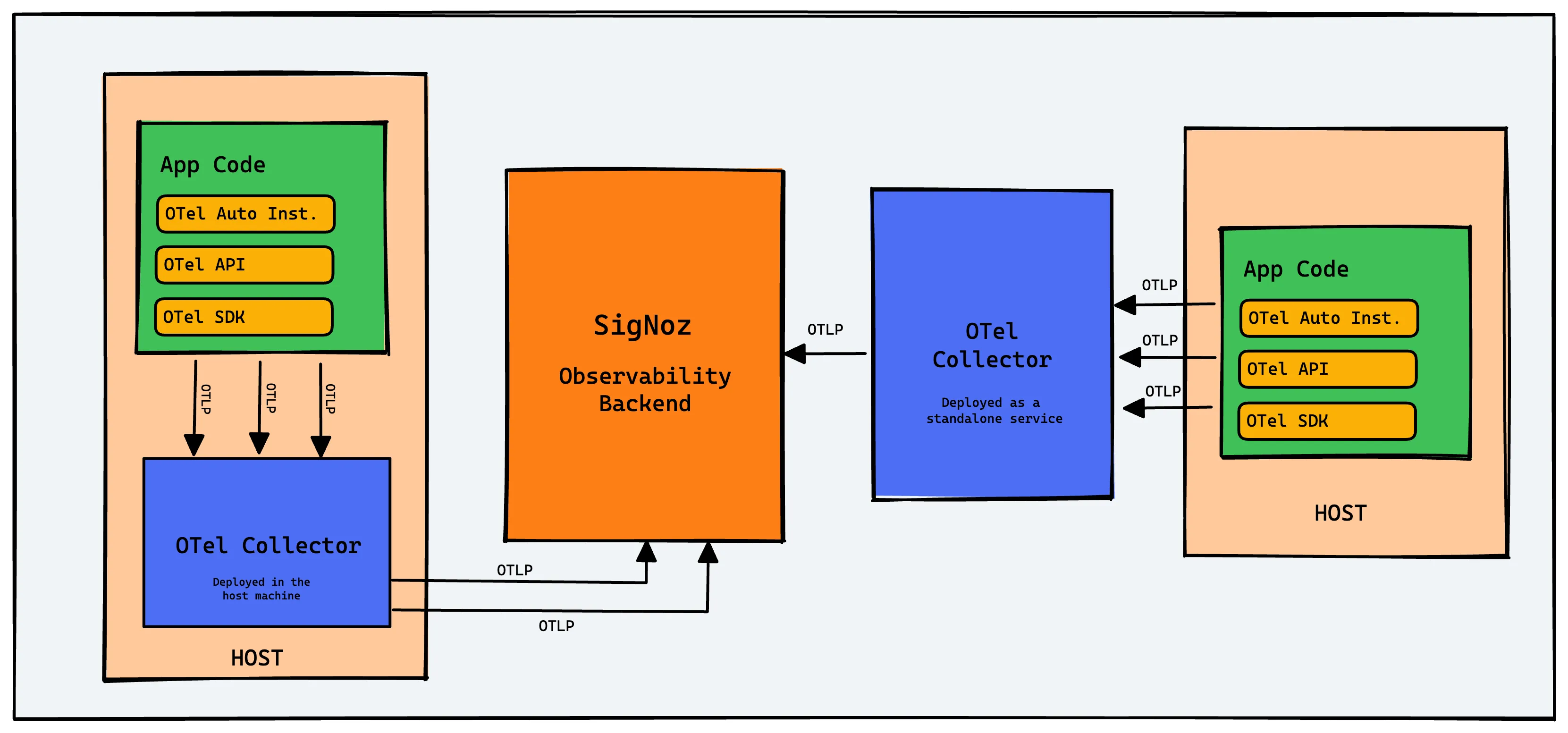
OpenTelemetry is the bedrock for setting up an observability framework. It also provides you the freedom to choose a backend analysis tool of your choice. You will never get locked in with any vendor if you use OpenTelemetry for your instrumentation layer.
OpenTelemetry and SigNoz
In this tutorial, we will use SigNoz as our backend analysis tool. SigNoz is a full-stack open-source APM tool that can be used for storing and visualizing the telemetry data collected with OpenTelemetry. It is built natively on OpenTelemetry and works on the OTLP data formats. SigNoz provides query and visualization capabilities for the end-user and comes with out-of-box charts for application metrics and traces.
Now let’s get down to how to implement OpenTelemetry Nextjs libraries for a sample Nextjs application and then visualize the collected data in SigNoz.
Creating a SigNoz cloud account
SigNoz cloud is the easiest way to run SigNoz. You can sign up here for a free account and get 30 days of unlimited access to all features.
After you sign up and verify your email, you will be provided with details of your SigNoz cloud instance. Once you set up your password and log in, you will be greeted with the following onboarding screen.
Creating a sample Nextjs application
Before instrumenting your application, you'll need a Next.js app to work with. If you already have one, you can skip to the next section. Otherwise, follow these steps to create a new Next.js application or check out the sample application at this GitHub repo.
Prerequisites
Node.js: Ensure you have Node.js 12.22.0 or later installed on your machine. You can verify your Node.js version by running:
node -v
Package Manager: You can use
npm
,yarn
, orpnpm
as your package manager.
Steps to Create a New Next.js Application
To get started with building a Next.js application, follow these steps to quickly set up your project using the official create-next-app
CLI tool. It automates the setup process, ensuring you have everything you need to begin developing.
Step 1: Create a New project
Use one of the following commands based on your preferred package manager:
Using
npx
with npm:npx create-next-app@latest
Using Yarn:
yarn create next-app
Using pnpm:
pnpm create next-app
Step 2: Follow the Interactive Prompts
The CLI will prompt you for information such as the project name, template selection, and whether to use TypeScript, ESLint, etc. Fill in the details as per your requirements.
Step 3: Navigate to the Project Directory:
cd your-project-name
Step 4: Start the Development Server
Use the appropriate command based on your package manager:
npm:
npm run dev
yarn
yarn dev
pnpm
pnpm dev
Step 5: Verify the Application is Running
Open your browser and navigate to http://localhost:3000. You should see the default Next.js welcome page.
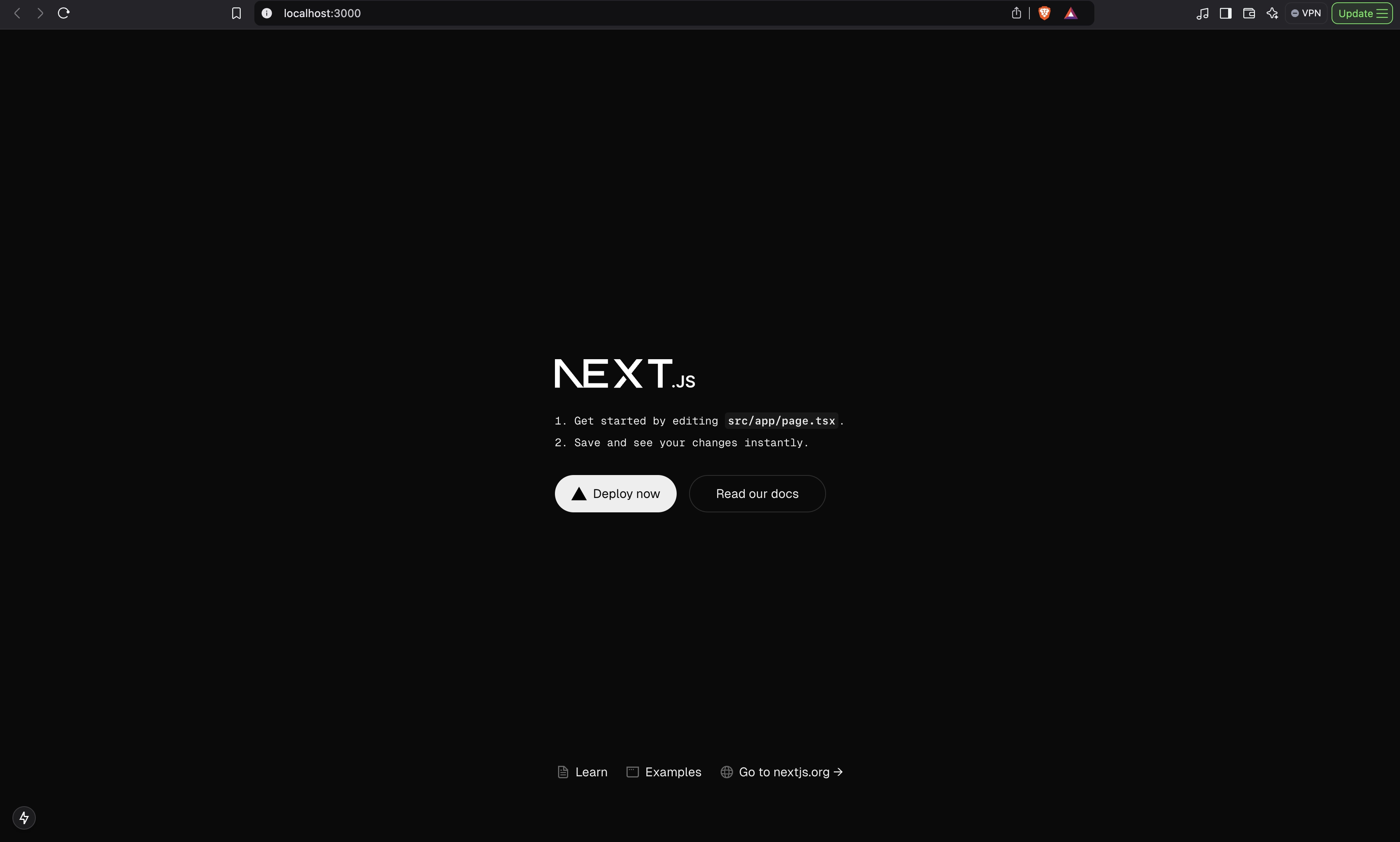
Instrumenting the Nextjs application with OpenTelemetry
Instrumenting your application involves integrating OpenTelemetry libraries to collect telemetry data (metrics, logs, traces) and configuring exporters to send this data to SigNoz for analysis.
There are two primary methods to instrument a Next.js application:
- Using Vercel OTEL Integration
- Using OpenTelemetry SDK (Node.js Runtime Only)
We'll cover both options in detail.
Option 1: Using Vercel OTEL Integration
Vercel OTEL Integration simplifies the process of adding OpenTelemetry to your Next.js application by providing a streamlined setup. This method is suitable if you're deploying your application on Vercel or prefer an easier integration process.
Step 1: Install Required Packages
Begin by installing the necessary OpenTelemetry and Vercel OTEL packages.
npm install @vercel/otel @opentelemetry/sdk-logs @opentelemetry/api-logs @opentelemetry/instrumentation @opentelemetry/exporter-trace-otlp-http
Where:
@vercel/otel
: Vercel's OpenTelemetry integration package.@opentelemetry/sdk-logs
: SDK for handling log data.@opentelemetry/api-logs
: API for log instrumentation.@opentelemetry/instrumentation
: Core instrumentation utilities.@opentelemetry/exporter-trace-otlp-http
: Exporter for sending trace data via OTLP over HTTP.
Alternative with Yarn or pnpm:
If you prefer using Yarn or pnpm, replace npm install
with yarn add
or pnpm add
Step 2: Configure the Trace Exporter
Create a file named instrumentation.node.ts
at the root of your project. This file configures the OpenTelemetry trace exporter, which sends trace data to SigNoz.
'use strict'
import { OTLPTraceExporter } from '@opentelemetry/exporter-trace-otlp-http';
// Add otel logging
import { diag, DiagConsoleLogger, DiagLogLevel } from '@opentelemetry/api';
diag.setLogger(new DiagConsoleLogger(), DiagLogLevel.ERROR); // set diaglog level to DEBUG when debugging
const exporterOptions = {
url: 'https://ingest.[region].signoz.cloud:443/v1/traces', // Set your own data region or set to http://localhost:4318/v1/traces if using selfhost SigNoz
headers: { 'signoz-ingestion-keys': '[YOUR-SIGNOZ-INGESTION-KEY]' }, // Set if you are using SigNoz Cloud
}
export const traceExporter = new OTLPTraceExporter(exporterOptions);
- Diagnostics Configuration:
diag.setLogger(new DiagConsoleLogger(), DiagLogLevel.ERROR);
: Sets up a console logger for OpenTelemetry diagnostics. TheDiagLogLevel.ERROR
ensures that only error messages are logged. For debugging purposes, you can set this toDiagLogLevel.DEBUG
to receive more verbose logs.
- Exporter Options:
url
: The endpoint where trace data will be sent. The full URL format ishttps://ingest.[region].signoz.cloud:443/v1/traces
. Depending on the choice of your region for SigNoz cloud, the ingest endpoint will vary according to this table.Region Endpoint US ingest.us.signoz.cloud:443/v1/traces IN ingest.in.signoz.cloud:443/v1/traces EU ingest.eu.signoz.cloud:443/v1/traces headers
: Contains thesignoz-ingestion-keys
, which is your SigNoz ingestion key. This key authenticates your application with SigNoz and ensures that data is sent securely. If you haven't created one yet, you can follow this guide to generate your ingestion key.
Step 3. Register Instrumentation
Create another file named instrumentation.ts
at the root of your project. This file registers the OpenTelemetry instrumentation with Vercel's OTEL integration.
import { registerOTel } from '@vercel/otel';
import { traceExporter } from './instrumentation.node';
export function register() {
registerOTel({
serviceName: 'Sample Next.js App',
traceExporter: traceExporter,
});
}
registerOTel
: A function provided by@vercel/otel
that initializes OpenTelemetry with the given configurations.serviceName
: A unique name for your application. This name will appear in the SigNoz dashboard, helping you identify traces from different services.traceExporter
: The exporter instance we configured ininstrumentation.node.ts
that sends trace data to SigNoz.
💡 Note: Ensure that both instrumentation.node.ts
and instrumentation.ts
are placed in the root directory of your project. If you use a src
folder, place them inside src
alongside other directories like pages
and app
. You can also check the sample application at this GitHub repo.
Step 4. Run and Validate
Once you're done configuring the exporter options, try running your application and validate if your application is sending traces to SigNoz cloud here.
npm
npm run dev
yarn
yarn dev
pnpm
pnpm dev
Generate Telemetry Data
To begin generating telemetry data for analysis, open your browser and navigate to http://localhost:3000. Ensure that your application is running and accessible.
Hit the URL a couple of times to generate some dummy data which can later be visualized and monitored in SigNoz.
Option 2: Using OpenTelemetry SDK (Node.js Runtime Only)
This method provides more granular control over the OpenTelemetry setup and is ideal for advanced use cases or self-hosted environments. It involves manually configuring the OpenTelemetry SDK without relying on Vercel's OTEL integration.
Step 1: Install Required Packages
Install the necessary OpenTelemetry packages for Node.js instrumentation.
npm i @opentelemetry/sdk-node
npm i @opentelemetry/auto-instrumentations-node
npm i @opentelemetry/exporter-trace-otlp-http
npm i @opentelemetry/resources
npm i @opentelemetry/semantic-conventions
Where:
@opentelemetry/sdk-node
: Provides the core SDK for Node.js applications.@opentelemetry/auto-instrumentations-node
: Automatically instruments supported Node.js libraries and frameworks.@opentelemetry/exporter-trace-otlp-http
: Exporter for sending trace data via OTLP over HTTP.@opentelemetry/resources
: Allows defining resource attributes (like service name).@opentelemetry/semantic-conventions
: Provides standard semantic attributes for resources and spans.
Step 2: Configure the OpenTelemetry SDK
Create a file named instrumentation.node.ts
at the root of your project. This file sets up the OpenTelemetry SDK, configures automatic instrumentation for the Node.js backend (which powers Next.js), and defines the trace exporter for sending telemetry data to SigNoz.
'use strict'
import process from 'process';
import {NodeSDK} from '@opentelemetry/sdk-node';
import { getNodeAutoInstrumentations } from '@opentelemetry/auto-instrumentations-node';
import { OTLPTraceExporter } from '@opentelemetry/exporter-trace-otlp-http'
import { Resource } from '@opentelemetry/resources'
import { SEMRESATTRS_SERVICE_NAME } from '@opentelemetry/semantic-conventions'
// Add otel logging
import { diag, DiagConsoleLogger, DiagLogLevel } from '@opentelemetry/api';
diag.setLogger(new DiagConsoleLogger(), DiagLogLevel.ERROR); // set diaglog level to DEBUG when debugging
const exporterOptions = {
url: 'https://ingest.[region].signoz.cloud:443/v1/traces', // use your own data region
headers: { 'signoz-ingestion-key': 'SIGNOZ_INGESTION_KEY' }, // Use if you are using SigNoz Cloud
}
const traceExporter = new OTLPTraceExporter(exporterOptions);
const sdk = new NodeSDK({
traceExporter,
instrumentations: [getNodeAutoInstrumentations()],
resource: new Resource({
[SEMRESATTRS_SERVICE_NAME]: 'next-app',
}),
});
// initialize the SDK and register with the OpenTelemetry API
// this enables the API to record telemetry
sdk.start()
// gracefully shut down the SDK on process exit
process.on('SIGTERM', () => {
sdk.shutdown()
.then(() => console.log('Tracing terminated'))
.catch((error) => console.log('Error terminating tracing', error))
.finally(() => process.exit(0));
});
This setup ensures that OpenTelemetry traces are sent to SigNoz for your Next.js application’s backend (Node.js). It:
- Automatically instruments your Node.js backend (Next.js API routes, server-side rendering, etc.).
- Sends trace data to SigNoz, where you can analyze performance metrics.
- Logs OpenTelemetry diagnostic information at the error level.
- Gracefully shuts down the SDK when your application terminates, ensuring that traces are properly exported before exit.
💡 Note: Be sure to replace 'SIGNOZ_INGESTION_KEY'
with your actual SigNoz ingestion key. If you haven't created one yet, follow this guide to generate your ingestion key. Make sure to update the URL with the correct SigNoz ingestion endpoint for your region, as explained in the previous step.
Step 3: Register Instrumentation
Create another file named instrumentation.ts
at the root of your project. This file conditionally imports the Node.js instrumentation based on the runtime environment.
/**
* Registers OpenTelemetry instrumentation based on the runtime environment.
*
* - If running in Node.js, it imports and initializes the Node.js instrumentation.
*/
export async function register() {
if (process.env.NEXT_RUNTIME === 'nodejs') {
await import('./instrumentation.node')
}
}
Conditional Import:
- The function
register
checks if the application is running in the Node.js runtime environment by examining theNEXT_RUNTIME
environment variable. - If true, it dynamically imports the
instrumentation.node.ts
file, initializing the OpenTelemetry SDK for Node.js.
Step 5: Run and Validate
With the SDK configured, proceed to run your application and verify that telemetry data is being sent to SigNoz cloud here.
npm
npm run dev
yarn
yarn dev
pnpm
pnpm dev
Generate Telemetry Data
To begin generating telemetry data for analysis, open your browser and navigate to http://localhost:3000. Ensure that your application is running and accessible.
Hit the URL a couple of times to generate some dummy data which can later be visualized and monitored in SigNoz.
Monitoring your Application in SigNoz
Once your Next.js application is instrumented and running and telemetry data is being generated, the next step is to validate that this data is being received in SigNoz and begin monitoring your application's performance.
To get started, log in to your SigNoz cloud account. Under the Services
tab on the SigNoz dashboard, you should see your application listed among other services.
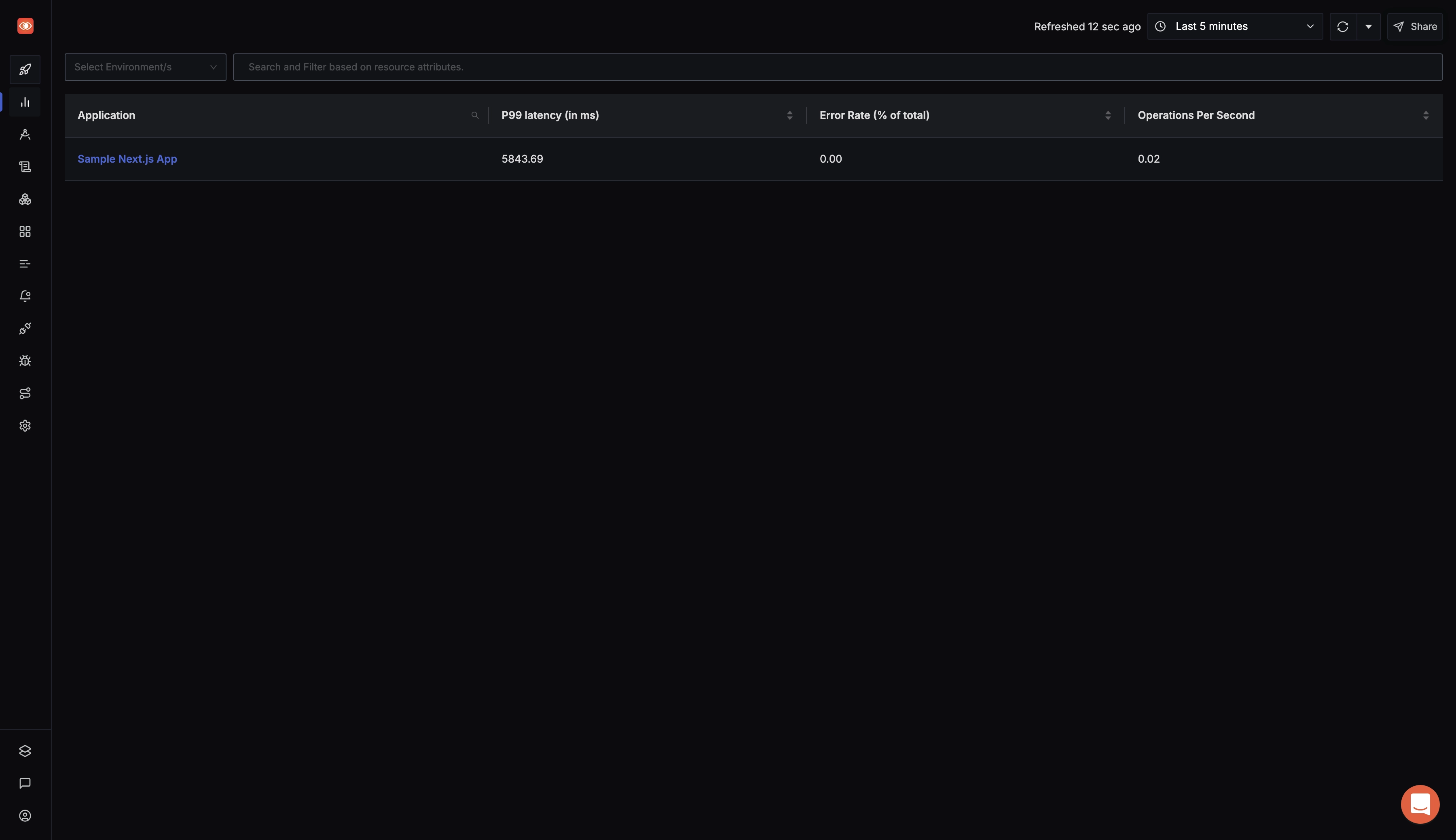
Click on your application to access the Metrics
page. This page provides out-of-the-box, pre-built dashboards displaying critical application metrics such as application latency, requests per second, and error percentages.
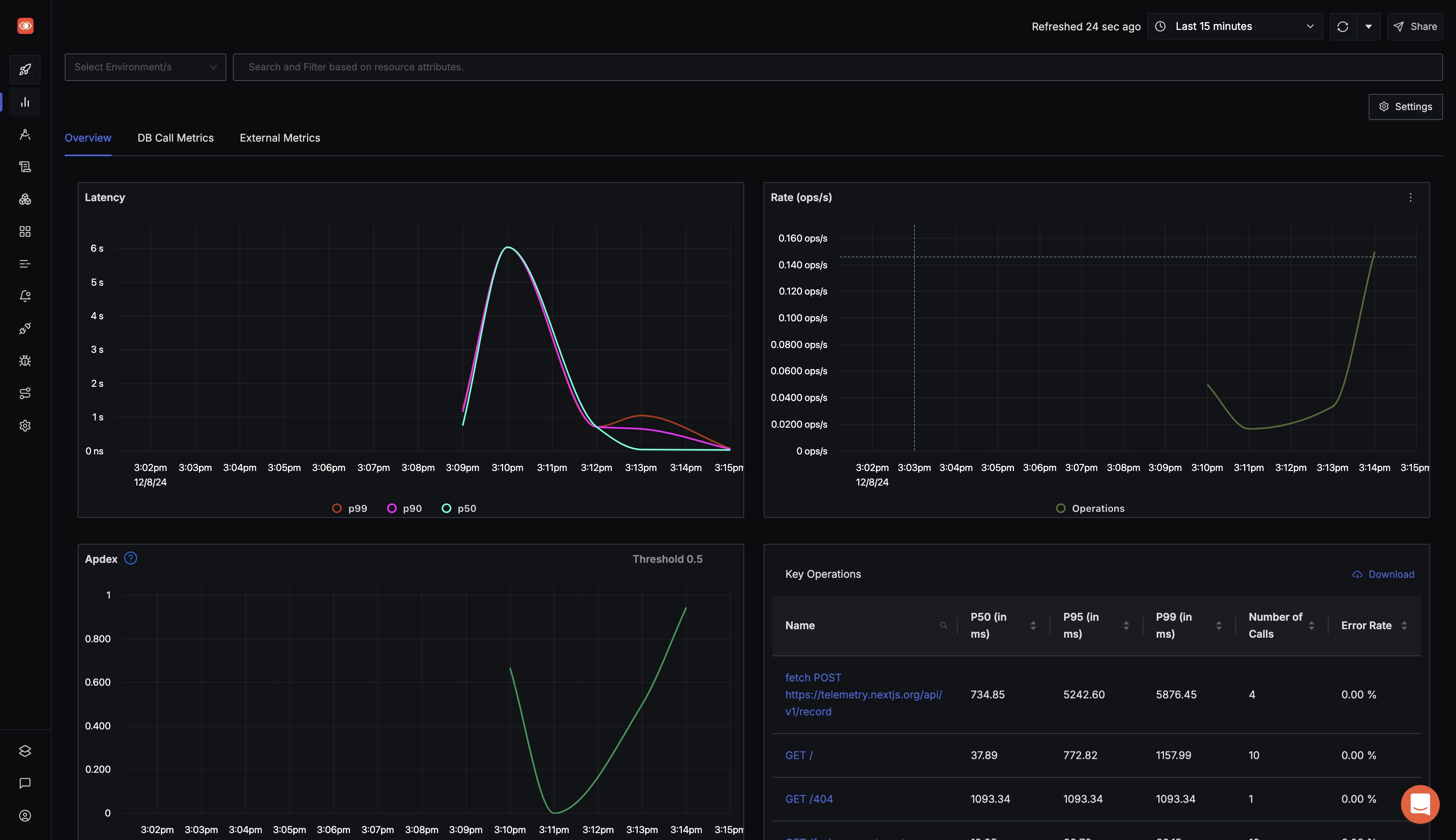
OpenTelemetry also captures tracing data, which is invaluable for visualizing how user requests flow through various services, especially in multi-service architectures. To visualize your application’s tracing data, navigate to the Traces
section via the left sidebar of the SigNoz dashboard.
In the Traces
tab, you can filter tracing data by tags, status codes, service names, operations, and more. This filtering capability makes it easier to isolate and analyze specific requests or identify performance bottlenecks.
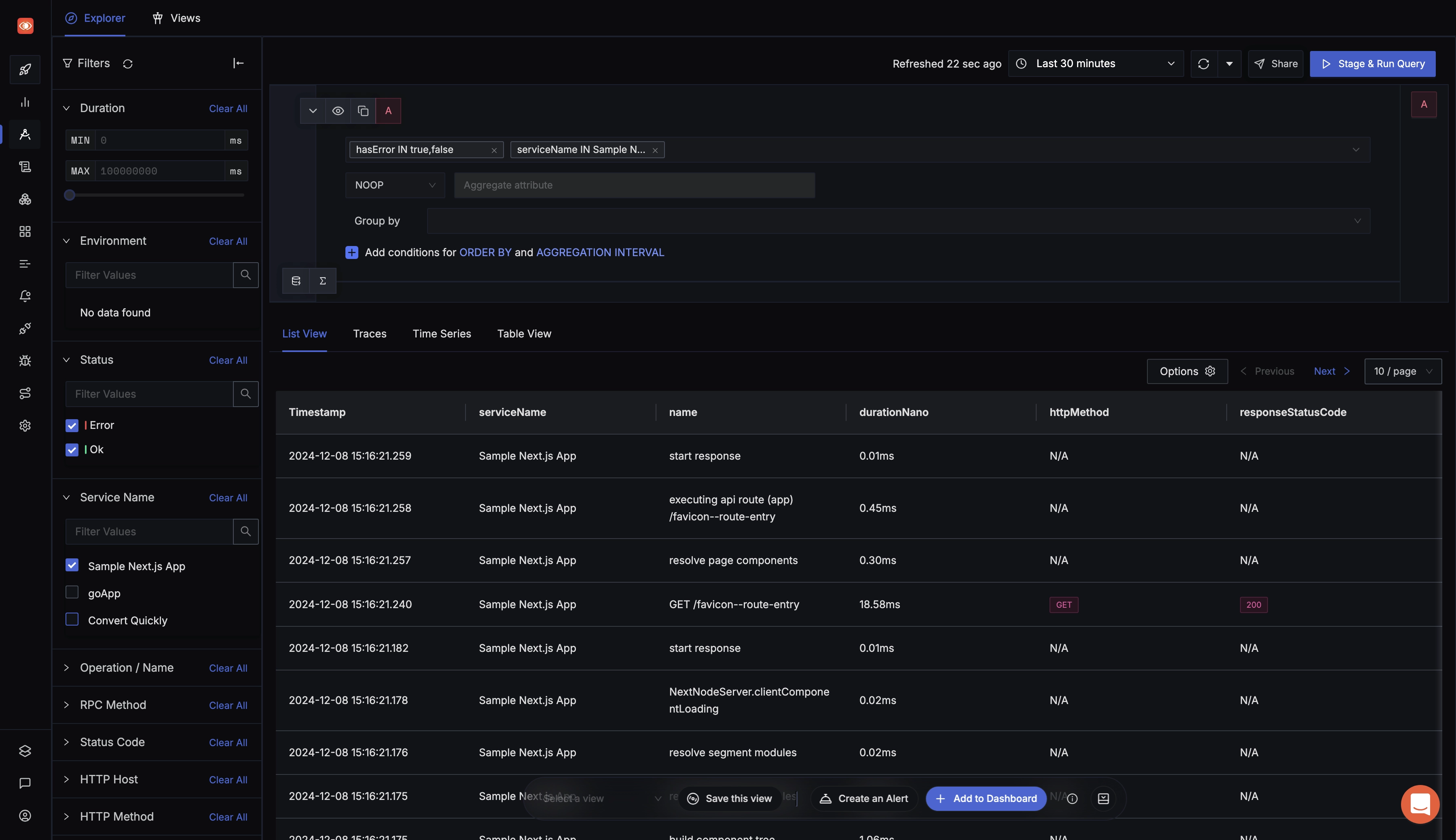
Tracing data can also be visualized using flamegraphs and Gantt charts, which provide a clear picture of how requests are handled across services. These visualizations make it easy to pinpoint inefficiencies and understand the end-to-end performance of your application.
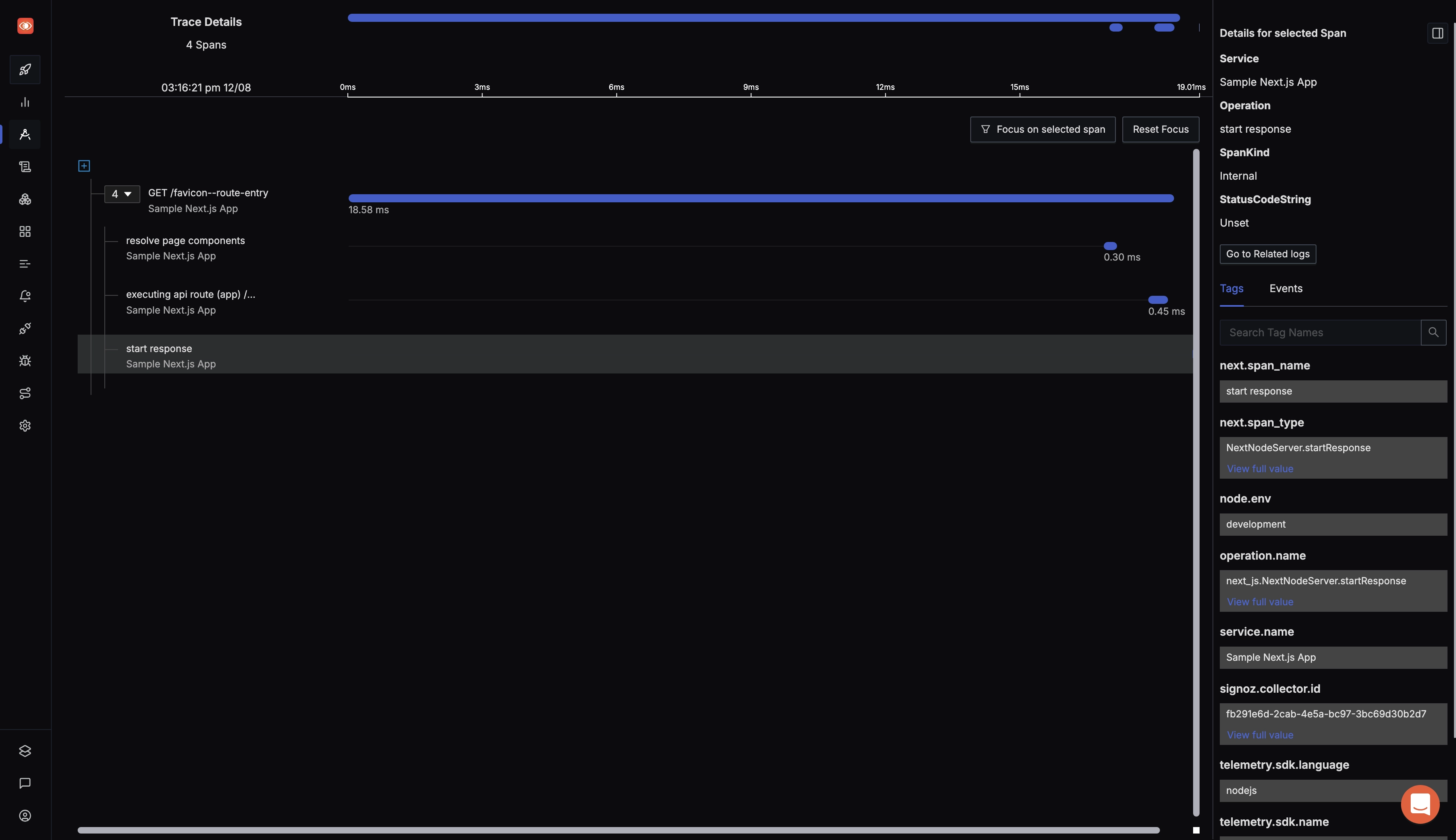
Conclusion
Using OpenTelemetry libraries, you can instrument your Nextjs applications for end-to-end tracing. You can then use an open-source APM tool like SigNoz to ensure the smooth performance of your Nextjs application.
OpenTelemetry is the future for setting up observability for cloud-native apps. It is backed by a huge community and covers a wide variety of technology and frameworks. Using OpenTelemetry, engineering teams can instrument polyglot and distributed applications and be assured about compatibility with a lot of technologies.
SigNoz cloud is the easiest way to run SigNoz. You can sign up here for a free account and get 30 days of unlimited access to all features.
If you face any issues while trying out SigNoz, you can reach out with your questions in #support channel 👇
Further Reading
Implementing OpenTelemetry in Angular application
Monitor your Nodejs application with OpenTelemetry and SigNoz