Web Vitals Monitoring
This documentation provides a step-by-step guide to setting up web vitals monitoring using SigNoz and OpenTelemetry.
Overview
Web vitals are essential metrics to measure the user experience on your website. SigNoz allows you to monitor these vitals seamlessly using OpenTelemetry. The key web vitals are:
- Largest Contentful Paint (LCP)
- First Input Delay (FID)
- Cumulative Layout Shift (CLS)
- Time to First Byte (TTFB)
- Total Blocking Time (TBT)
Prerequisites
- SigNoz Cloud account
- A web application where you want to monitor web vitals
Setup
Step 1: Setup OTel Collector
Install Otel collector binary using these instructions.
Step 2: Install dependencies
npm install web-vitals
npm install @opentelemetry/api
npm install @opentelemetry/sdk-trace-web
npm install @opentelemetry/auto-instrumentations-web
npm install @opentelemetry/exporter-trace-otlp-http
npm install @opentelemetry/exporter-metrics-otlp-http
npm install @opentelemetry/sdk-metrics
npm install @opentelemetry/resources
npm install @opentelemetry/semantic-conventions
Step 3: Create an instrumentation file
This file (e.g., instrument.ts
) is needed to setup the MeterProvider
which is used to create custom metrics.
import { MeterProvider, PeriodicExportingMetricReader } from '@opentelemetry/sdk-metrics';
import { OTLPMetricExporter } from '@opentelemetry/exporter-metrics-otlp-http';
import { resourceFromAttributes } from '@opentelemetry/resources';
import { metrics } from '@opentelemetry/api';
// Define your resource, e.g., service name, environment.
const resource = resourceFromAttributes({
'service.name': 'yourServiceName',
});
// Create a metric reader with OTLP exporter configured to send metrics to a local collector.
const metricReader = new PeriodicExportingMetricReader({
exporter: new OTLPMetricExporter({
url: 'http://127.0.0.1:4318/v1/metrics',
}),
exportIntervalMillis: 10000, // Export metrics every 10 seconds.
});
// Initialize a MeterProvider with the above configurations.
const myServiceMeterProvider = new MeterProvider({
resource,
readers: [metricReader],
});
// Set the initialized MeterProvider as global to enable metric collection across the app.
metrics.setGlobalMeterProvider(myServiceMeterProvider);
Step 4: Capture Web Vitals
Use the web-vitals library to capture key performance metrics like CLS, FID, LCP, TTFB, and FCP. These metrics are captured through callback functions that you define.
import { onCLS, onFCP, onFID, onLCP, onTTFB } from 'web-vitals';
function yourCustomCallback(metric) {
// Send the metric to your analytics server or perform any custom logic
}
onCLS(yourCustomCallback);
onFID(yourCustomCallback);
onLCP(yourCustomCallback);
onTTFB(yourCustomCallback);
onFCP(yourCustomCallback);
Step 5: Export Web Vitals
Using OpenTelemetry, create Observable Async Gauges to periodically observe and export these metrics. This ensures that the captured web vitals are correctly recorded and sent to SigNoz for monitoring.
import { metrics } from '@opentelemetry/api';
import { onCLS, onFCP, onFID, onLCP, onTTFB } from 'web-vitals';
const meter = metrics.getMeter('web-vitals');
const lcp = meter.createHistogram('lcp');
const cls = meter.createObservableGauge('cls');
const fid = meter.createHistogram('fid');
const ttfb = meter.createHistogram('ttfb');
const fcp = meter.createHistogram('fcp');
function sendToAnalytics(metric) {
switch (metric.name) {
case 'LCP': {
lcp.record(metric.value);
break;
}
case 'CLS': {
cls.addCallback((result) => {
result.observe(metric.value);
});
break;
}
case 'FID': {
fid.record(metric.value);
break;
}
case 'TTFB': {
ttfb.record(metric.value);
break;
}
case 'FCP': {
fcp.record(metric.value);
break;
}
default: {
console.log('unexpected metric name');
}
}
}
onCLS(sendToAnalytics);
onFID(sendToAnalytics);
onLCP(sendToAnalytics);
onTTFB(sendToAnalytics);
onFCP(sendToAnalytics);
This code captures web vitals (LCP, CLS, FID, TTFB, FCP) using the web-vitals library and logs these metrics, setting up observable gauges with OpenTelemetry to record the values for each metric. It defines a callback function, sendToAnalytics, that processes and observes each metric value, ensuring they are monitored appropriately.
To better understand how this is used, you can check out this GitHub link which shows how web-vitals are used for monitoring SigNoz.
Step 6: Setup Dashboard and alerts
You can create a custom dashboard and set alerts to monitor your core web-vitals and be notified about anything critical.
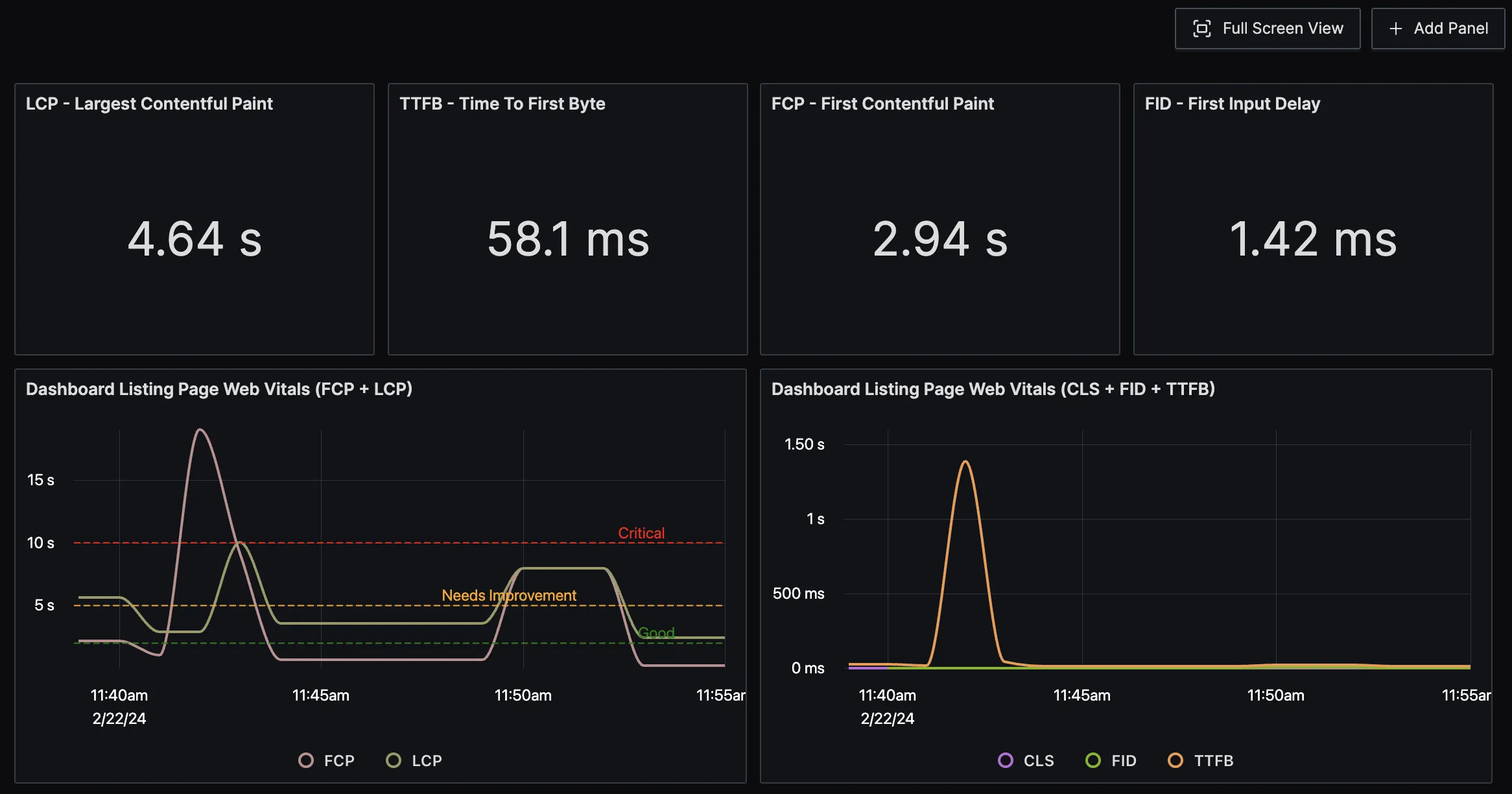
One of the cool feature of SigNoz charts is that you can create thresholds in the chart with different color coding. For example, in the below graph there are three thresholds for good
, needs improvement
, and critical
level.
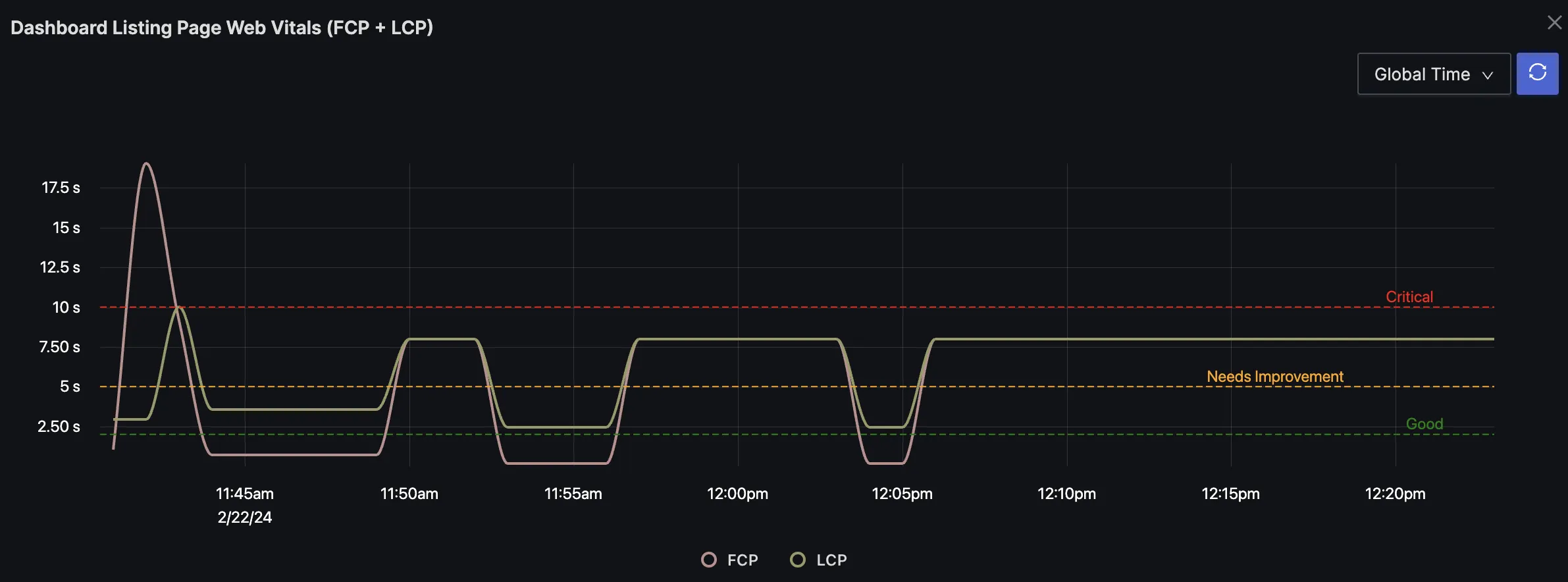