Error Logging is a mechanism that helps capture and record the errors or issues occurring in the application. Errors refer to issues that disrupt the normal operation of the application, such as missing files, server failures, or network problems. It is very helpful to grab attention at the earliest and debug the cause of the problem. Error logs are crucial for monitoring and security management.
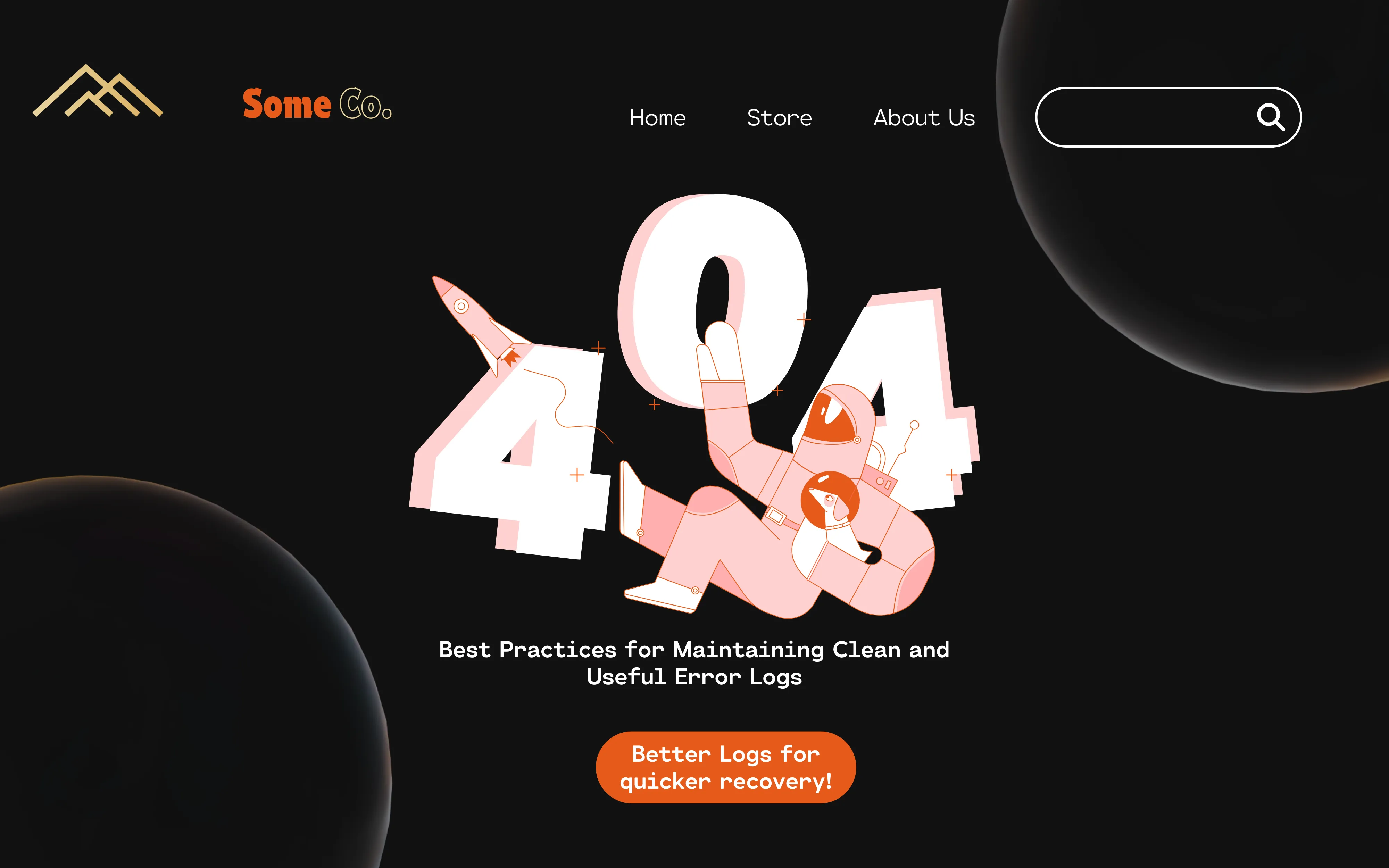
Error logs are specifically meant to record errors, which can be related to server or network operating systems or third-party applications. A well-written error log provides enough information to locate and resolve the problem. Additionally, it may indicate the seriousness of the issue along with details of the module or trace ID to dig deeper.
Example: Reading a File in Java
Let us take an example of reading a file in Java. If the file exists we can simply read the file but if the file is corrupt or does not exist then we must log an error to inform that the reading of the file failed so the developer can take proper actions.
import java.io.File;
import java.io.FileNotFoundException;
import java.text.SimpleDateFormat;
import java.util.Scanner;
import java.util.logging.Level;
import java.util.logging.Logger;
public class MyProgram {
private static final Logger logger = Logger.getLogger(MyProgram.class.getName());
private static final SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
public static void main(String[] args) {
File dataFile = new File("data.txt");
try {
Scanner scanner = new Scanner(dataFile);
while (scanner.hasNextLine()) {
String line = scanner.nextLine();
System.out.println(line);
}
scanner.close();
} catch (FileNotFoundException e) {
String timestamp = sdf.format(new java.util.Date());
logger.log(Level.SEVERE, timestamp + " Error reading data file: " + dataFile.getAbsolutePath(), e);
}
}
}
Output:
2024-07-13 00:30:00.000 SEVERE: MyProgram [path.to.your.package.MyProgram] - Error reading data file: /path/to/your/data.txt
The above output log provides a timestamp, severity level SEVERE
, class name, and the error message along with the file path, helping developers quickly identify and address the issue. In output we get the complete path of the file because when we create a file object with the relative path, the file is created in the current directory and its relative path. The getAbsolutePath()
method on the File
object gets the absolute path of the file using the path of the current directory and returns a string containing absolute path.
Importance of Error Logs
- Faster Troubleshooting - When non-desired behavior is detected, error logs are the first thing checked to figure out root cause, identify the type of the error, and get additional contextual detail along with stack traces. This lookup saves hours of debugging code and speeds up the troubleshooting process.
- Quick Decision Making - Error logs assist in identifying areas of concern and the parts of the application affected by the issue. In large programs, it can be challenging to locate these areas manually. Error logs enable rapid identification and addressing of critical issues, facilitating swift decision-making.
- Better Performance - Error logs can reveal patterns that cause the system to run slowly or impact performance. By analyzing past logs, trends, and recurring errors, developers can identify and rectify issues that degrade system performance, preventing major breakdowns.
- Better Security - Error logs play a crucial role in security management by reporting suspicious activities such as unauthorized access or security breaches. They provide information on the specific areas where the malfunction occurred, helping to improve overall security measures.
Key Components of Error Logs
Irrespective of the programming language or the platform error logs must contain these pieces of information. to make the most out of it.
Timestamp
The first element is a timestamp. A properly formatted timestamp, including the date and the exact time of the event's occurrence, must be included. The format should be consistent for the entire application or the company’s standard format.
A precise timestamp can help developers track the occurrence of events across different systems and understand what led to an error in the first place. It usually appears at the beginning of the log.
Severity Level
A Severity Level indicates the seriousness of the log record. Higher severity indicates quick attention. Error Log Level is a severe level and needs immediate attention. Other log levels include DEBUG, WARN, INFO, FATAL, etc. FATA or SEVERE is even more severe than ERROR, rest are less serious and are used either just for info or debugging.
Error Code
Common errors are usually given a unique code to identify them and resolve them easily. This quick reference helps on understand the error and what potential places could be causing it. Error code is of much help in large systems.
Error Message (stack traces)
The error message for exceptions or critical errors can contain a stack trace of the call sequence that led to the error. Giving context about errors helps in diagnosing the issue faster. Many times the error is thrown at a certain method and is caught much later.
Source
The source refers to the class, method, module, etc. from where the error is logged. Source is a must even if we don't provide a complete stack trace. The same errors can be raised from multiple locations in large systems where the source can help identify potential causes.
Types of Error Logs
Error logs can be categorized based on their origin like application, server, hardware, etc. to resolve the error.
Application Logs - Application logs capture software-specific logs for example application crash exception or unexpected behavior.
Example:
2024-07-18 14:30:15 ERROR [UserService] - NullPointerException: User object is null java.lang.NullPointerException: Cannot invoke "User.getUsername()" because "user" is null at com.example.UserService.processUser(UserService.java:45) at com.example.UserController.getUserDetails(UserController.java:30)
This log shows a NullPointerException in the UserService, providing the exact line and method where the error occurred.
System Logs - System logs are more focused on operating systems, hardware failures, performance metrics, etc. These help monitor the efficiency and troubleshoot system-wide issues specific to single or multiple systems.
Example:
Jul 18 15:45:23 server kernel: [62456.302490] Out of memory: Kill process 1234 (java) score 236 or sacrifice child Jul 18 15:45:23 server kernel: [62456.302495] Killed process 1234 (java) total-vm:8052516kB, anon-rss:7836428kB, file-rss:0kB, shmem-rss:0kB
This system log shows an out-of-memory error that resulted in the termination of a Java process.
Security Logs - These capture security issues and detect suspicious actions and security breaches. It is useful to separate them from the application logs as these can be separately forwarded to security developers.
Example:
2024-07-18 16:20:45 WARN [SecurityFilter] - Failed login attempt for user 'admin' from IP 192.168.1.100 2024-07-18 16:20:50 ERROR [SecurityFilter] - Multiple failed login attempts detected. Possible brute force attack from IP 192.168.1.100
This security log shows failed login attempts and a potential security threat.
Network Logs (Grouped under System Logs) - Network-related issues like high traffic, multiple attempts to connect, problems with data transformation, server downtime, etc. are covered under this. It lets network engineers solve the issue faster by providing device ID, timestamp, error message, etc.
Example:
2024-07-18 17:05:30 ERROR [NetworkMonitor] - Connection timeout: Unable to reach database server at 10.0.0.5:5432 2024-07-18 17:05:35 WARN [NetworkMonitor] - High network latency detected: 500ms response time from API server
This network log shows a database connection timeout and a warning about high network latency.
By categorizing logs in this manner, teams can quickly identify the source of an error and route it to the appropriate team for resolution. For instance, application logs would typically be handled by software developers, system logs by system administrators, security logs by the security team, and network logs by network engineers.
SigNoz supports various log collection methods, including OpenTelemetry and legacy logging libraries.
Common Error Logging Tools
JUL (Java Util Logging)
JUL is a standard logging library in Java. It offers basic logging functionalities like level categorization, set output various destinations, etc. JUL is simple to use and comes bundled with the Java Development Kit (JDK).
LOG4J
Log4J was a popular logging framework for Java applications. However, it has several critical security vulnerabilities and therefore advanced frameworks are in use nowadays.
LogBack
It is a successor of Log4J and it addresses security concerns and offers enhanced features. It offers flexibility in configuration and allows customizations in logging behavior, output formats, and filtering options.
LOG4J 2
It is rewritten and designed from beginning, it offers better performance, scalability, and security. It supports features like asynchronous logging and pluggable appenders.
SLF4J
It is not a logging framework it is a logging facade. It acts as a bridge between frameworks and standard logging API. It simplifies switching between various frameworks without having to change code.
Best Practices for Effective Error Logging
Consistent Formatting - Use the same structure throughout or make a standard for consistent logging. For example,
[Timestamp] [Severity Level] [Source] [Message] [Context]
This will help the developer understand the log at first glance and can only refer to sections of their interest.
Comprehensive Logging - While logging errors are crucial, logging every relevant event is necessary. Log all the informational messages, warnings, user actions, etc. to get an entire picture of the system and its flow.
Regular Monitoring - Make use of these logs by monitoring and analyzing them regularly to make the system robust. Consider leveraging automated tools available for monitoring, analysis, and alerts.
Alerting and Notification - To minimize downtime and address critical issues faster, developers can set up appropriate notification channels to notify relevant teams of critical errors. Remember when some e-commerce websites sold iPhones at an 80-90% discount due to some technical errors and faced a huge loss, it can be helpful in such situations to detect unusual activity.
Log Retention Policies - Servers and applications are running constantly and adding huge amounts of logs which need to be taken care of. Thus, we need policies to decide for how long we can keep the logs handy, what happens to the logs after that period, what kind of logs to save and which ones to discard, etc. Proper regulations need to be made to make logs manageable and make the process cost-efficient.
Security Considerations - Sensitive information of the company must not be logged like passwords, API keys, personal information, etc. Additionally, logs contain important information so these must be stored securely with encryption with access controls.
Create Meaningful Alerts - Rather than having generic alerts or alerts for every small error, write alerts for meaningful and big errors only. There is a possibility of the alert being ignored if sent too frequently or not given proper information on the alert.
Troubleshooting with Error Logs
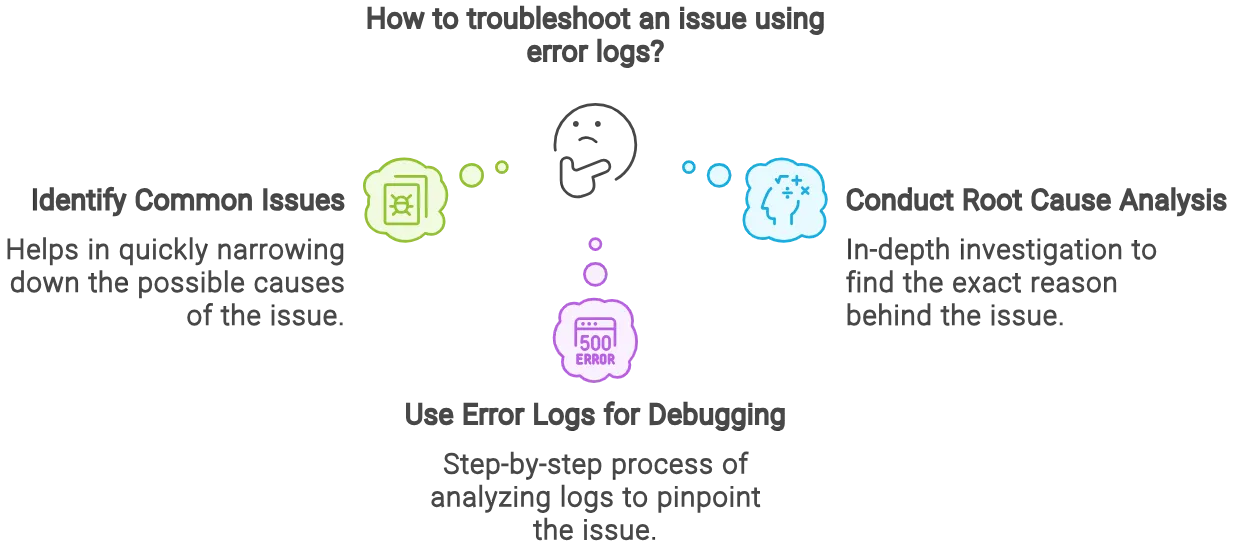
- Identifying Common Issues - The very first step in troubleshooting is to identify errors that occur frequently. This can be done by aggregating logs from various sources, filtering and searching using tools to identify frequent log messages, and recognizing patterns to resolve issues.
- Root Cause Analysis - Once the error is logged, the next step is to find the origin of the issue. Root Cause Analysis (RCA) is crucial for pinpointing the exact origin of issues, preventing recurrence, enhancing system performance, and improving decision-making. This can be done using log context, looking for recurring patterns in the errors, using stack traces, and using monitoring tools.
- Using Error Logs for Debugging - Error logs are effectively used for debugging. Error messages provide enough information to debug the issues.
SigNoz provides functionalities for capturing and analyzing exceptions and errors.
Real-World Scenario
Let us take an example of the issue where users are not able to log in to a web application. The first step will be gathering all the logs and finding logs where the user is logging in. If the issue is with all the users common errors can be useful. If only some users are facing this issue, then the root cause of the issue must be analyzed.
Next, look for errors or exceptions reporting that the user login failed. It can be due to several issues, there can be a problem with the authentication, database connection, or issue with the input method.
Error logs along with other logs are used to identify the issue. Developers can try reproducing the issue using stack traces and logs. One caught issue can be resolved and users can effortlessly log in again.
Example Module: Potential login failure
File: LoginController.java
public class LoginController {
public String loginUser(String username, String password) {
try {
User user = validateCredentials(username, password); // This might throw an exception
return loginSuccess(user);
} catch (LoginException e) {
// Log the error and return a login failed message
logError(e.getMessage());
return "Login failed!";
}
}
}
File: UserService.java
public class UserService {
public User validateCredentials(String username, String password) throws LoginException {
// Code to check username and password against database
if (!isValidPassword(username, password)) {
throw new LoginException("Invalid username or password");
}
return getUserByUsername(username);
}
}
Potential Stack Trace:
java.lang.RuntimeException: Login failed!
at LoginController.loginUser(LoginController.java:20)
at LoginServlet.doPost(LoginServlet.java:35)
... (other function calls)
Caused by: com.myapp.LoginException: Invalid username or password
at UserService.validateCredentials(UserService.java:42)
... (other function calls)
Debugging Using Logs:
- The error log in
LoginController
indicates aLoginException
was thrown. - The stack trace points to the
validateCredentials
method inUserService
as the source of the exception. - Further investigation in
UserService
reveals the specific issue is an invalid username or password.
Common Pitfalls in Error Logging and How to Avoid Them
While error logging is crucial for maintaining and troubleshooting applications, there are several common mistakes that developers and teams often make. Being aware of these pitfalls can help you implement more effective error logging practices.
Overly Verbose Logging
Pitfall: Logging too much information can lead to log bloat, making it difficult to find relevant information when needed.
How to avoid:
- Use appropriate log levels (DEBUG, INFO, WARN, ERROR) and adjust them based on the environment (development, staging, production).
- Implement log sampling for high-volume, low-priority logs.
Insufficient Context in Log Messages
Pitfall: Vague log messages without enough context can make troubleshooting difficult.
How to avoid:
- Include relevant details such as user IDs, request IDs, and specific variables in your log messages.
- Use structured logging to make logs more easily parseable and searchable.
Logging Sensitive Information
Pitfall: Accidentally logging sensitive data like passwords, API keys, or personal information can lead to security breaches.
How to avoid:
- Implement data masking for sensitive fields.
- Use secure logging practices and regularly audit logs for sensitive information.
Inconsistent Log Formats
Pitfall: Inconsistent log formats across different parts of the application can make log analysis challenging.
How to avoid:
- Establish and enforce a consistent log format across your entire application or organization.
- Use a logging framework that encourages consistent formatting.
Neglecting Error Log Monitoring
Pitfall: Setting up logging but not actively monitoring or analyzing the logs defeats the purpose of logging.
How to avoid:
- Implement automated log monitoring and alerting systems.
- Regularly review and analyze logs, not just when issues occur.
Failure to Log Exception Stack Traces
Pitfall: Logging only the exception message without the stack trace can make it difficult to trace the root cause of an error.
How to avoid:
- Always include the full stack trace when logging exceptions.
- Use logging frameworks that automatically include stack traces.
Inadequate Log Retention
Pitfall: Not retaining logs for a sufficient period can hinder historical analysis and compliance efforts.
How to avoid:
- Implement a log retention policy that balances storage costs with analytical and compliance needs.
- Consider using log archival solutions for long-term storage of important logs.
Ignoring Performance Impact
Pitfall: Excessive logging, especially in high-traffic applications, can impact performance.
How to avoid:
- Use asynchronous logging to minimize impact on application performance.
- Benchmark your logging implementation and optimize as necessary.
Lack of Standardization Across Teams
Pitfall: Different teams using different logging practices can lead to inconsistency and confusion.
How to avoid:
- Establish organization-wide logging standards and best practices.
- Provide training and tools to ensure all teams follow the same logging guidelines.
Failing to Evolve Logging Practices
Pitfall: Not adapting logging practices as the application grows and changes can lead to outdated or irrelevant logs.
How to avoid:
- Regularly review and update logging practices.
- Seek feedback from developers and operations teams on the usefulness of current logs.
By being aware of these common pitfalls and taking steps to avoid them, you can significantly improve the effectiveness of your error logging practices. Remember, the goal of error logging is to provide clear, actionable information that helps quickly identify and resolve issues in your application.
Send Error Logs to SigNoz
An observability platform helps us to collect, monitor, and manage logs effectively. A robust log monitoring system is crucial for modern-day applications.
SigNoz is a full-stack open-source APM tool that simplifies the process of monitoring logs, metrics, and traces in a single pane of glass.
The logs tab in SigNoz is packed with advanced features that streamline the process of analyzing logs. Features such as a log query builder, search across multiple fields, structured table view, and JSON view make the process of analyzing logs easier and more efficient.
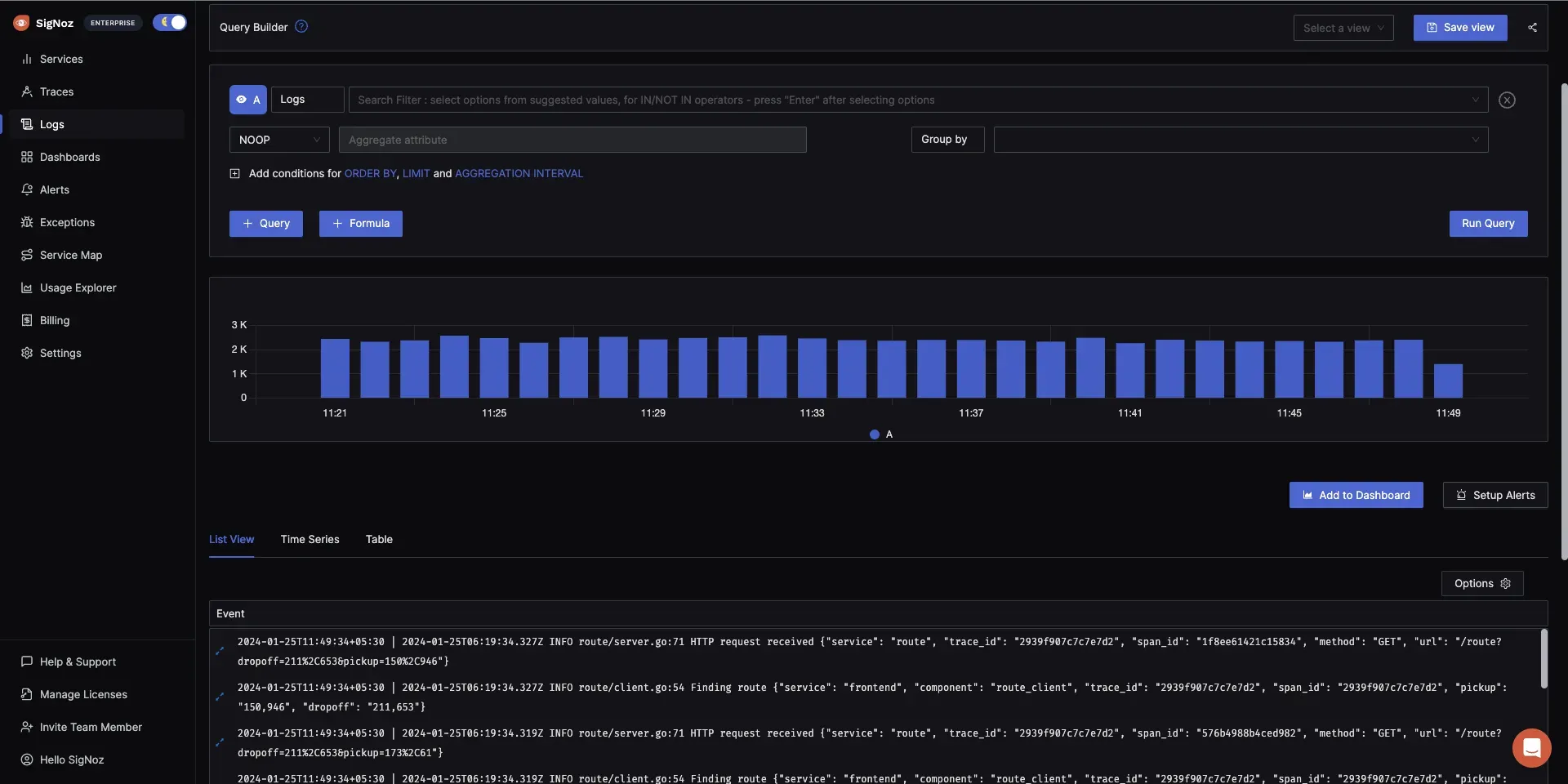
Logs management in SigNoz
SigNoz offers real-time analysis of logs, enabling you to search, filter, and visualize them as they are generated. This can assist in identifying patterns, trends, and problems in the logs and resolving issues efficiently.
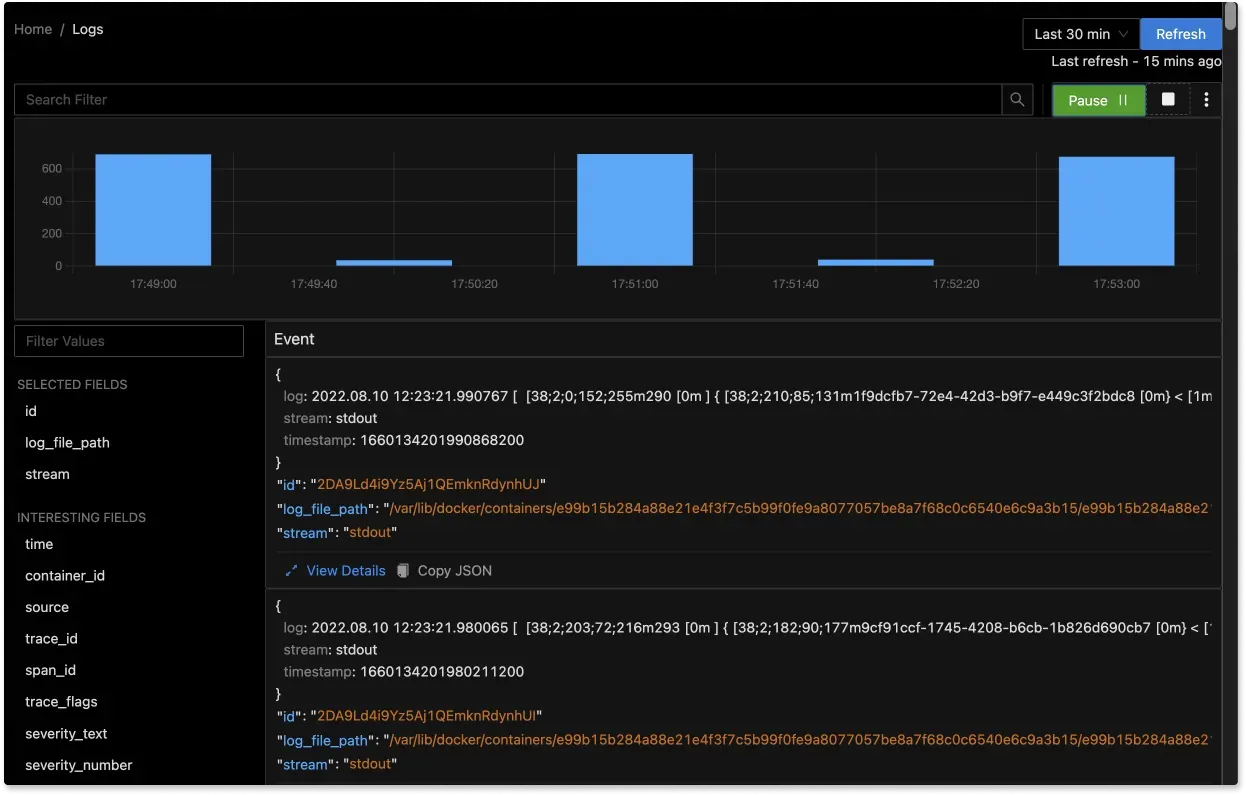
Live tail logging in SigNoz
With the advanced Log Query Builder, you can filter out logs quickly with a mix and match of fields.
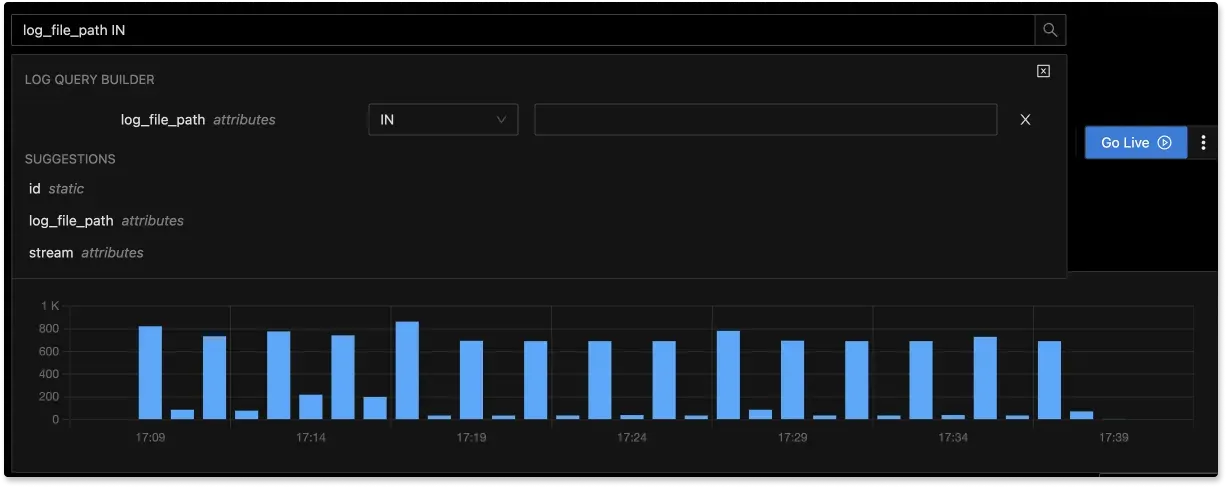
Advanced query builder to search and filter logs quickly in SigNoz dashboard
Refer to this article for complete details on using SigNoz for analyzing error logs.
Conclusion
- Error logs are used to log severe issues with the application. These help in debugging errors, better performance, and better security.
- Error logs consist of timestamp, security level, error code, error message, and source.
- The error logs can further be divided into different types to send the logs to appropriate teams. Different types of error logs are application logs, system logs, security logs, and network logs.
- Common error logging tools are JUL, Log4J, LogBack, Log4J 2, SLF4J.
- It is very important to follow best practices for effective logging. Make sure to do consistent formatting, regular monitoring, create meaningful alerts and notifications and set proper log retention policies.
FAQs
What is the error code log?
An error code log can be a file or a record representing the error within a software application, system, or service. It contains information about the error such as error code, error message, date and time, etc.
What is the log error function?
Error Log function is a function used to log an error. It helps developers add a log of the level error, provide a message, the method from which the error is generated, etc.
How to find error from log file?
There are several methods to filter error logs from a log file, we can manually search using the file search
option, and there are CLI or UI tools that can help filter and analyze the logs, we can also set the log level to error to get the logs which are error or higher priority.
What is HTTP error log?
HTTP error log reports HTTP-related errors such as 404 (Not found), 500 (Internal Server Error), etc. A web server encounters these and indicates problems with serving web requests.