Nestjs OpenTelemetry Instrumentation
This document contains instructions on how to set up OpenTelemetry instrumentation in your Nestjs applications. OpenTelemetry, also known as OTel for short, is an open source observability framework that can help you generate and collect telemetry data - traces, metrics, and logs from your Nestjs application.
Requirements
Supported Versions
>=4.0.0
Send traces to SigNoz Cloud
Based on your application environment, you can choose the setup below to send traces to SigNoz Cloud.
From VMs, there are two ways to send data to SigNoz Cloud.
Send traces directly to SigNoz Cloud
Step 1. Install OpenTelemetry packages
npm install --save @opentelemetry/api@^1.6.0
npm install --save @opentelemetry/sdk-node@^0.45.0
npm install --save @opentelemetry/auto-instrumentations-node@^0.39.4
npm install --save @opentelemetry/exporter-trace-otlp-http@^0.45.0
Step 2. Create tracer.ts
file
You need to configure the endpoint for SigNoz cloud in this file.
'use strict';
import { getNodeAutoInstrumentations } from '@opentelemetry/auto-instrumentations-node';
import { OTLPTraceExporter } from '@opentelemetry/exporter-trace-otlp-http';
import { Resource } from '@opentelemetry/resources';
import * as opentelemetry from '@opentelemetry/sdk-node';
import { SemanticResourceAttributes } from '@opentelemetry/semantic-conventions';
// Configure the SDK to export telemetry data to the console
// Enable all auto-instrumentations from the meta package
const exporterOptions = {
//highlight-start
url: 'https://ingest.{region}.signoz.cloud:443/v1/traces',
//highlight-end
};
const traceExporter = new OTLPTraceExporter(exporterOptions);
const sdk = new opentelemetry.NodeSDK({
traceExporter,
instrumentations: [getNodeAutoInstrumentations()],
resource: new Resource({
[SemanticResourceAttributes.SERVICE_NAME]: '<service_name>',
}),
});
// initialize the SDK and register with the OpenTelemetry API
// this enables the API to record telemetry
sdk.start();
// gracefully shut down the SDK on process exit
process.on('SIGTERM', () => {
sdk
.shutdown()
.then(() => console.log('Tracing terminated'))
.catch((error) => console.log('Error terminating tracing', error))
.finally(() => process.exit(0));
});
export default sdk;
<service_name>
: Name of your service.
Depending on the choice of your region for SigNoz cloud, the ingest endpoint will vary according to this table.
Region | Endpoint |
---|---|
US | ingest.us.signoz.cloud:443/v1/traces |
IN | ingest.in.signoz.cloud:443/v1/traces |
EU | ingest.eu.signoz.cloud:443/v1/traces |
Step 3. On main.ts
file or file where your app starts import tracer using below command.
The below import should be the first line in the main file of your application (Ex -> main.ts
)
const tracer = require('./tracer')
Step 4. Start the tracer
In the async function boostrap
section of the application code, initialize the tracer as follows:
const tracer = require('./tracer')
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
// All of your application code and any imports that should leverage
// OpenTelemetry automatic instrumentation must go here.
async function bootstrap() {
// highlight-start
await tracer.start();
//highlight-end
const app = await NestFactory.create(AppModule);
await app.listen(3001);
}
bootstrap();
Step 5. Run the application
OTEL_EXPORTER_OTLP_HEADERS="signoz-access-token=<SIGNOZ_INGESTION_KEY>" nest start
The data captured with OpenTelemetry from your application should start showing on the SigNoz dashboard.
SIGNOZ_INGESTION_KEY
is the API token provided by SigNoz. You can find your ingestion key from SigNoz cloud account details sent on your email.
Step 6. You can validate if your application is sending traces to SigNoz cloud here.
In case you encounter an issue where all applications do not get listed in the services section then please refer to the troubleshooting section.
Send traces via OTel Collector binary
OTel Collector binary helps to collect logs, hostmetrics, resource and infra attributes. It is recommended to install Otel Collector binary to collect and send traces to SigNoz cloud. You can correlate signals and have rich contextual data through this way.
You can find instructions to install OTel Collector binary here in your VM. Once you are done setting up your OTel Collector binary, you can follow the below steps for instrumenting your Javascript application.
Step 1. Install OpenTelemetry packages
npm install --save @opentelemetry/api@^1.6.0
npm install --save @opentelemetry/sdk-node@^0.45.0
npm install --save @opentelemetry/auto-instrumentations-node@^0.39.4
npm install --save @opentelemetry/exporter-trace-otlp-http@^0.45.0
Step 2. Create tracer.ts
file
'use strict';
import { getNodeAutoInstrumentations } from '@opentelemetry/auto-instrumentations-node';
import { OTLPTraceExporter } from '@opentelemetry/exporter-trace-otlp-http';
import { Resource } from '@opentelemetry/resources';
import * as opentelemetry from '@opentelemetry/sdk-node';
import { SemanticResourceAttributes } from '@opentelemetry/semantic-conventions';
// Configure the SDK to export telemetry data to the console
// Enable all auto-instrumentations from the meta package
const exporterOptions = {
//highlight-start
url: 'http://localhost:4318/v1/traces',
//highlight-end
};
const traceExporter = new OTLPTraceExporter(exporterOptions);
const sdk = new opentelemetry.NodeSDK({
traceExporter,
instrumentations: [getNodeAutoInstrumentations()],
resource: new Resource({
[SemanticResourceAttributes.SERVICE_NAME]: '<service_name>',
}),
});
// initialize the SDK and register with the OpenTelemetry API
// this enables the API to record telemetry
sdk.start();
// gracefully shut down the SDK on process exit
process.on('SIGTERM', () => {
sdk
.shutdown()
.then(() => console.log('Tracing terminated'))
.catch((error) => console.log('Error terminating tracing', error))
.finally(() => process.exit(0));
});
export default sdk;
<service_name>
: Name of your service.
Step 3. On main.ts
file or file where your app starts import tracer using below command.
The below import should be the first line in the main file of your application (Ex -> main.ts
)
const tracer = require('./tracer')
Step 4. Start the tracer
In the async function boostrap
section of the application code, initialize the tracer as follows:
const tracer = require('./tracer')
import { NestFactory } from '@nestjs/core';
import { AppModule } from './app.module';
// All of your application code and any imports that should leverage
// OpenTelemetry automatic instrumentation must go here.
async function bootstrap() {
// highlight-start
await tracer.start();
//highlight-end
const app = await NestFactory.create(AppModule);
await app.listen(3001);
}
bootstrap();
Step 5. Run the application
nest start
Step 6. You can validate if your application is sending traces to SigNoz cloud here.
In case you encounter an issue where all applications do not get listed in the services section then please refer to the troubleshooting section.
Send Traces to Self-Hosted SigNoz
There are three major steps to using OpenTelemetry:
- Instrumenting your Nestjs application with OpenTelemetry
- Configuring exporter to send data to SigNoz
- Validating that configuration to ensure that data is being sent as expected.
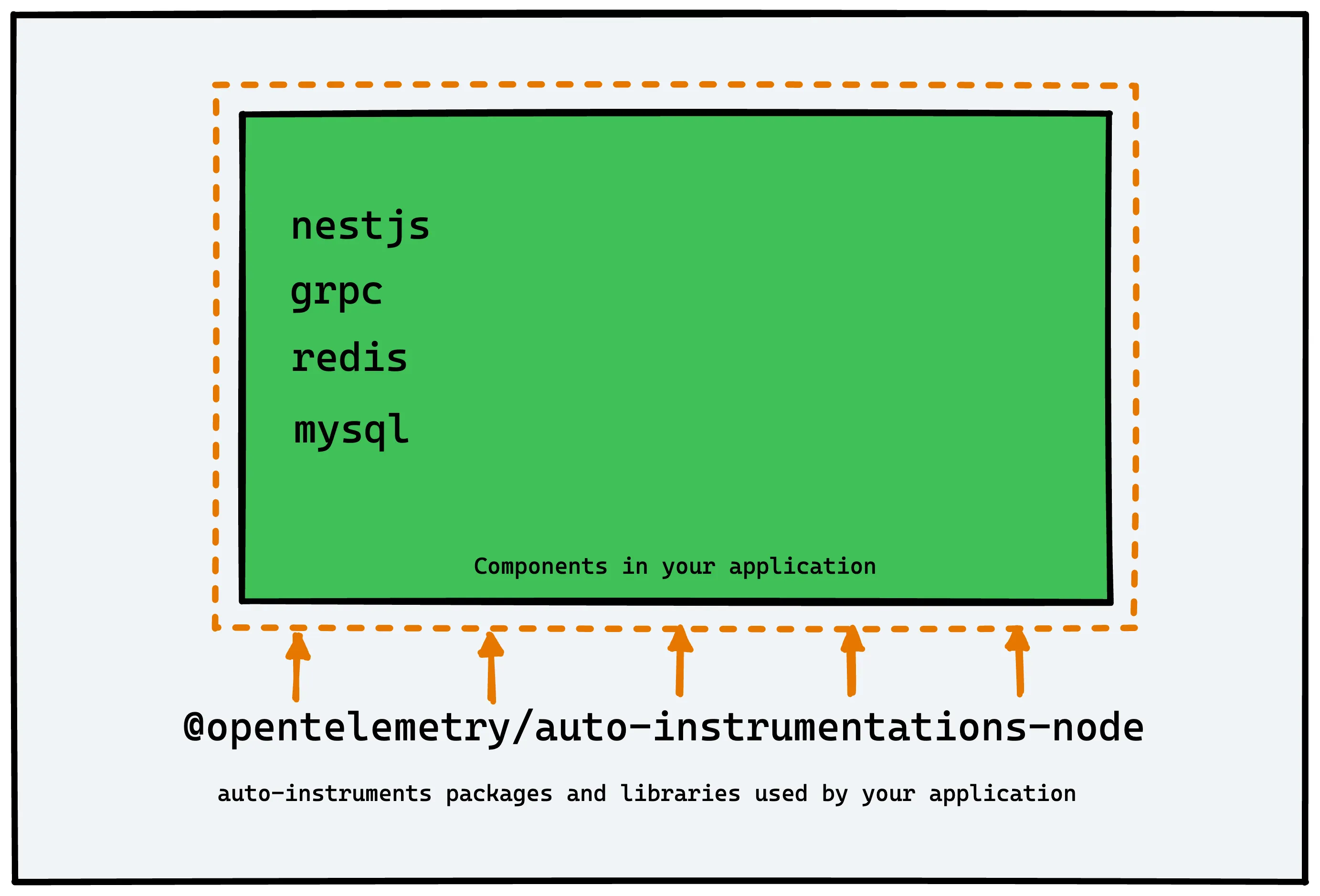
You have two choices for instrumenting your NestJS application with OpenTelemetry.
Use the all-in-one auto-instrumentation library(Recommended)
The auto-instrumentation library of OpenTelemetry is a meta package that provides a simple way to initialize multiple Nodejs instrumnetations.✅ InfoIf you are on K8s, you should checkout opentelemetry operators which enable auto instrumenting Javascript applications very easily.
Use a specific auto-instrumentation library
You can use individual auto-instrumentation libraries too for a specific component of your application. For example, you can use@opentelemetry/instrumentation-nestjs-core
for instrumenting the Nestjs web framework.
Let's see how to instrument your Nestjs application with OpenTelemetry.
Using the all-in-one auto-instrumentation library
The recommended way to instrument your Nestjs application is to use the all-in-one auto-instrumentation library - @opentelemetry/auto-instrumentations-node
. It provides a simple way to initialize multiple Nodejs instrumentations.
Internally, it calls the specific auto-instrumentation library for components used in the application. You can see the complete list here.
Steps to auto-instrument Nestjs application
Install the dependencies
We start by installing the relevant dependencies.npm install --save @opentelemetry/sdk-node npm install --save @opentelemetry/auto-instrumentations-node npm install --save @opentelemetry/exporter-trace-otlp-http
📝 NoteIf you run into any error, you might want to use these pinned versions of OpenTelemetry libraries used in this GitHub repo.
Create a
tracer.ts
file
'use strict';
import { getNodeAutoInstrumentations } from '@opentelemetry/auto-instrumentations-node';
import { OTLPTraceExporter } from '@opentelemetry/exporter-trace-otlp-http';
import { Resource } from '@opentelemetry/resources';
import * as opentelemetry from '@opentelemetry/sdk-node';
import { SemanticResourceAttributes } from '@opentelemetry/semantic-conventions';
// Configure the SDK to export telemetry data to the console
// Enable all auto-instrumentations from the meta package
const exporterOptions = {
//highlight-start
url: 'http://localhost:4318/v1/traces',
//highlight-end
};
const traceExporter = new OTLPTraceExporter(exporterOptions);
const sdk = new opentelemetry.NodeSDK({
traceExporter,
instrumentations: [getNodeAutoInstrumentations()],
resource: new Resource({
[SemanticResourceAttributes.SERVICE_NAME]: '<service_name>',
}),
});
// initialize the SDK and register with the OpenTelemetry API
// this enables the API to record telemetry
sdk.start();
// gracefully shut down the SDK on process exit
process.on('SIGTERM', () => {
sdk
.shutdown()
.then(() => console.log('Tracing terminated'))
.catch((error) => console.log('Error terminating tracing', error))
.finally(() => process.exit(0));
});
export default sdk;
<service_name>
: Name of your service.
On
main.ts
file or file where your app starts import tracer using below command.✅ InfoThe below import should be the first line in the main file of your application (Ex ->
main.ts
)const tracer = require('./tracer')
Start the tracer
In theasync function boostrap
section of the application code, initialize the tracer as follows:const tracer = require('./tracer') import { NestFactory } from '@nestjs/core'; import { AppModule } from './app.module'; // All of your application code and any imports that should leverage // OpenTelemetry automatic instrumentation must go here. async function bootstrap() { // highlight-start await tracer.start(); //highlight-end const app = await NestFactory.create(AppModule); await app.listen(3001); } bootstrap();
Run the application
nest start
The data captured with OpenTelemetry from your application should start showing on the SigNoz dashboard.
In case you encounter an issue where all applications do not get listed in the services section then please refer to the troubleshooting section.
Validating instrumentation by checking for traces
With your application running, you can verify that you’ve instrumented your application with OpenTelemetry correctly by confirming that tracing data is being reported to SigNoz.
To do this, you need to ensure that your application generates some data. Applications will not produce traces unless they are being interacted with, and OpenTelemetry will often buffer data before sending. So you need to interact with your application and wait for some time to see your tracing data in SigNoz.
Validate your traces in SigNoz:
- Trigger an action in your app that generates a web request. Hit the endpoint a number of times to generate some data. Then, wait for some time.
- In SigNoz, open the
Services
tab. Hit theRefresh
button on the top right corner, and your application should appear in the list ofApplications
. - Go to the
Traces
tab, and apply relevant filters to see your application’s traces.
You might see other dummy applications if you’re using SigNoz for the first time. You can remove it by following the docs here.
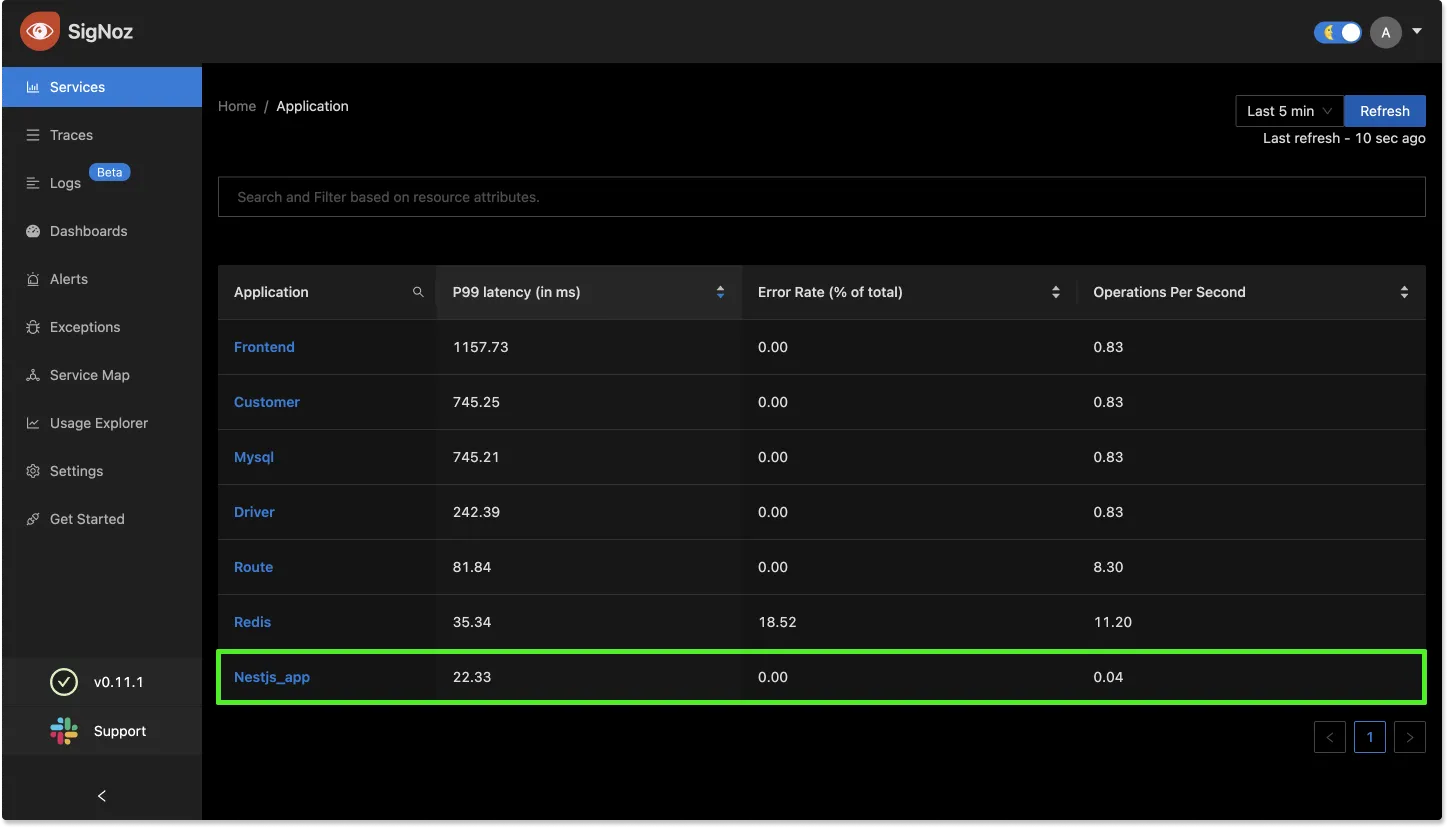
If you don't see your application reported in the list of services, try our troubleshooting guide.
Using a specific auto-instrumentation library
If you want to instrument only your Nestjs framework, then you need to use the following package:
npm install --save @opentelemetry/instrumentation-nestjs-core
In the above case, you will have to install packages for all the components that you want to instrument with OpenTelemetry individually. You can find detailed instructions here.
Instrumentation Modules for Databases
The @opentelemetry/auto-instrumentations-node
can inititialize instrumentation for popular databases. Hence it’s recommended to get started with it.
But if you are using specific auto-instrumentation packages, here’s a list of packages for popular databases.
MongoDB instrumentation
If you’re using @opentelemetry/auto-instrumentations-node
, you don’t need to install specific modules for your database.
Supported Versions
• >=3.3 <5
Module that provides automatic instrumentation for MongoDB:
npm install --save @opentelemetry/instrumentation-mongodb
Redis Instrumentation
If you’re using @opentelemetry/auto-instrumentations-node
, you don’t need to install specific modules for your database.
Supported Versions
This package supports redis@^2.6.0
and redis@^3.0.0
For version redis@^4.0.0
, please use @opentelemetry/instrumentation-redis-4
npm install --save @opentelemetry/instrumentation-redis
MySQL Instrumentation
If you’re using @opentelemetry/auto-instrumentations-node
, you don’t need to install specific modules for your database.
Supported Versions
• 2.x
Module that provides automatic instrumentation for MySQL:
npm install --save @opentelemetry/instrumentation-mysql
Memcached Instrumentation
If you’re using @opentelemetry/auto-instrumentations-node
, you don’t need to install specific modules for your database.
Supported Versions
>=2.2
Module that provides automatic instrumentation for Memcached:
npm install --save @opentelemetry/instrumentation-memcached
Troubleshooting your installation
Set an environment variable to run the OpenTelemetry launcher in debug mode, where it logs details about the configuration and emitted spans:
export OTEL_LOG_LEVEL=debug
The output may be very verbose with some benign errors. Early in the console output, look for logs about the configuration. Next, look for lines like the ones below, which are emitted when spans are emitted to SigNoz.
{
"traceId": "985b66d592a1299f7d12ebca56ca1fe3",
"parentId": "8d62a70aa335a227",
"name": "bar",
"id": "17ada85c3d55376a",
"kind": 0,
"timestamp": 1685674607399000,
"duration": 299,
"attributes": {},
"status": { "code": 0 },
"events": []
}
{
"traceId": "985b66d592a1299f7d12ebca56ca1fe3",
"name": "foo",
"id": "8d62a70aa335a227",
"kind": 0,
"timestamp": 1585130342183948,
"duration": 315,
"attributes": {
"name": "value"
},
"status": { "code": 0 },
"events": [
{
"name": "event in foo",
"time": [1585130342, 184213041]
}
]
}
Running short applications (Lambda/Serverless/etc) If your application exits quickly after startup, you may need to explicitly shutdown the tracer to ensure that all spans are flushed:
opentelemetry.trace.getTracer('your_tracer_name').getActiveSpanProcessor().shutdown()
Sample NestJs Application
- We have included a sample NestJs application with README.md at Sample NestJs App Github Repo.
Further Reading
Frequently Asked Questions
How to find what to use in
IP of SigNoz
if I have installed SigNoz in Kubernetes cluster?Based on where you have installed your application and where you have installed SigNoz, you need to find the right value for this. Please use this grid to find the value you should use for
IP of SigNoz
I am sending data from my application to SigNoz, but I don't see any events or graphs in the SigNoz dashboard. What should I do?
This could be because of one of the following reasons:
Your application is generating telemetry data, but not able to connect with SigNoz installation
Please use this troubleshooting guide to find if your application is able to access SigNoz installation and send data to it.
Your application is not actually generating telemetry data
Please check if the application is generating telemetry data first. You can use
Console Exporter
to just print your telemetry data in console first. Join our Slack Community if you need help on how to export your telemetry data in consoleYour SigNoz installation is not running or behind a firewall
Please double check if the pods in SigNoz installation are running fine.
docker ps
orkubectl get pods -n platform
are your friends for this.
What Cloud Endpoint Should I Use?
The primary method for sending data to SigNoz Cloud is through OTLP exporters. You can either send the data directly from your application using the exporters available in SDKs/language agents or send the data to a collector agent, which batches/enriches telemetry and sends it to the Cloud.
My Collector Sends Data to SigNoz Cloud
Using gRPC Exporter
The endpoint should be ingest.{region}.signoz.cloud:443
, where {region}
should be replaced with in
, us
, or eu
. Note that the exporter endpoint doesn't require a scheme for the gRPC exporter in the collector.
# Sample config with `us` region
exporters:
otlp:
endpoint: "ingest.us.signoz.cloud:443"
tls:
insecure: false
headers:
"signoz-access-token": "<SIGNOZ_INGESTION_KEY>"
Using HTTP Exporter
The endpoint should be https://ingest.{region}.signoz.cloud:443
, where {region}
should be replaced with in
, us
, or eu
. Note that the endpoint includes the scheme https
for the HTTP exporter in the collector.
# Sample config with `us` region
exporters:
otlphttp:
endpoint: "https://ingest.us.signoz.cloud:443"
tls:
insecure: false
headers:
"signoz-access-token": "<SIGNOZ_INGESTION_KEY>"
My Application Sends Data to SigNoz Cloud
The endpoint should be configured either with environment variables or in the SDK setup code.
Using Environment Variables
Using gRPC Exporter
Examples with us
region
OTEL_EXPORTER_OTLP_PROTOCOL=grpc OTEL_EXPORTER_OTLP_ENDPOINT=https://ingest.us.signoz.cloud:443 OTEL_EXPORTER_OTLP_HEADERS=signoz-access-token=<SIGNOZ_INGESTION_KEY>
Using HTTP Exporter
OTEL_EXPORTER_OTLP_PROTOCOL=http/protobuf OTEL_EXPORTER_OTLP_ENDPOINT=https://ingest.us.signoz.cloud:443 OTEL_EXPORTER_OTLP_HEADERS=signoz-access-token=<SIGNOZ_INGESTION_KEY>
Configuring Endpoint in Code
Please refer to the agent documentation.
Sending Data from a Third-Party Service
The endpoint configuration here depends on the export protocol supported by the third-party service. They may support either gRPC, HTTP, or both. Generally, you will need to adjust the host and port. The host address should be ingest.{region}.signoz.cloud:443
, where {region}
should be replaced with in
, us
, or eu
, and port 443
should be used.